About the Course
Have you always wanted to learn Python, but never quite known how to start?
More applications than we realize are being developed using Python because it is easy to learn, read, and write. You can now start learning the language quickly and effectively with the help of this interactive tutorial.
The Python Workshop starts by showing you how to correctly apply Python syntax to write simple programs, and how to use appropriate Python structures to store and retrieve data. You'll see how to handle files, deal with errors, and use classes and methods to write concise, reusable, and efficient code.
As you advance, you'll understand how to use the standard library, debug code to troubleshoot problems, and write unit tests to validate application behavior.
You'll gain insights into using the pandas and NumPy libraries for analyzing data, and the graphical libraries of Matplotlib and Seaborn to create impactful data visualizations. By focusing on entry-level data science, you'll build your practical Python skills in a way that mirrors real-world development. Finally, you'll discover the key steps in building and using simple machine learning algorithms.
By the end of this Python book, you'll have the knowledge, skills and confidence to creatively tackle your own ambitious projects with Python.
About the Chapters
Chapter 1, Vital Python: Math, Strings, Conditionals, Loops, explains how to write basic Python programs, and outlines the fundamentals of the Python language.
Chapter 2, Python Structures, covers the essential elements that are used to store and retrieve data in all programming languages.
Chapter 3, Executing Python: Programs, Algorithms, Functions, explains how to write more powerful and concise code through an increased appreciation of well-written algorithms, and an understanding of functions
Chapter 4, Extending Python, Files, Errors, Graphs, covers the basic I/O (input-output) operations for Python and covers using the matplotlib and seaborn libraries to create visualizations.
Chapter 5, Constructing Python: Classes and Methods, introduces one of the most central concepts in object-oriented programming classes, and it will help you write code using classes, which will make your life easier.
Chapter 6, The standard library, covers the importance of the Python standard library. It explains how to navigate in the standard Python libraries and overviews some of the most commonly used modules.
Chapter 7, Becoming Pythonic, covers the Python programming language, with which you will enjoy writing succinct, meaningful code. It also demonstrates some techniques for expressing yourself in ways that are familiar to other Python programmers.
Chapter 8, Software Development, covers how to debug and troubleshoot our applications, how to write tests to validate our code, and the documentation for other developers and users.
Chapter 9, Practical Python: Advanced Topics, explains how to take advantage of parallel programming, how to parse command-line arguments, how to encode and decode Unicode, and how to profile Python to discover and fix performance problems.
Chapter 10, Data Analytics with pandas and NumPy, covers data science, which is the core application of Python. We will be covering NumPy and pandas in this chapter.
Chapter 11, Machine Learning, covers the concept of machine learning and the steps involved in building a machine learning algorithm
Conventions
Code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles are shown as follows: "Python provides the collections.defaultdict
type."
A block of code is set as follows:
cubes = [x**3 for x in range(1,6)] print(cubes)
New important words are shown like this: "Typically, standalone .py files are either called scripts or modules".
Words that you see on the screen, for example, in menus or dialog boxes, appear in the text like this: "You can also use Jupyter (New -> Text File
)."
Long code snippets are truncated and the corresponding names of the code files on GitHub are placed at the top of the truncated code. The permalinks to the entire code are placed below the code snippet. It should look as follows:
Exercise66.ipynb
def annotate_heatmap(im, data=None, valfmt="{x:.2f}", textcolors=["black", "white"], threshold=None, **textkw): import matplotlib if not isinstance(data, (list, np.ndarray)):
https://packt.live/2ps1byv
Before You Begin
Each great journey begins with a humble step. Our upcoming adventure in the land of Python is no exception. Before you can begin, you need to be prepared with the most productive environment. In this section, you will see how to do that.
Installing Jupyter on your system
We will be using Python 3.7 (from https://python.org):
To install Jupyter on windows, MacOS and Linux follow these steps:
- Head to https://www.anaconda.com/distribution/ to install the Anaconda Navigator, which is an interface through which you can access your local Jupyter notebook.
- Now, based on your Operating system (Windows, macOS or Linux) you need to download the Anaconda Installer.
Have a look at the following figure where we have downloaded the Anaconda files for Windows:
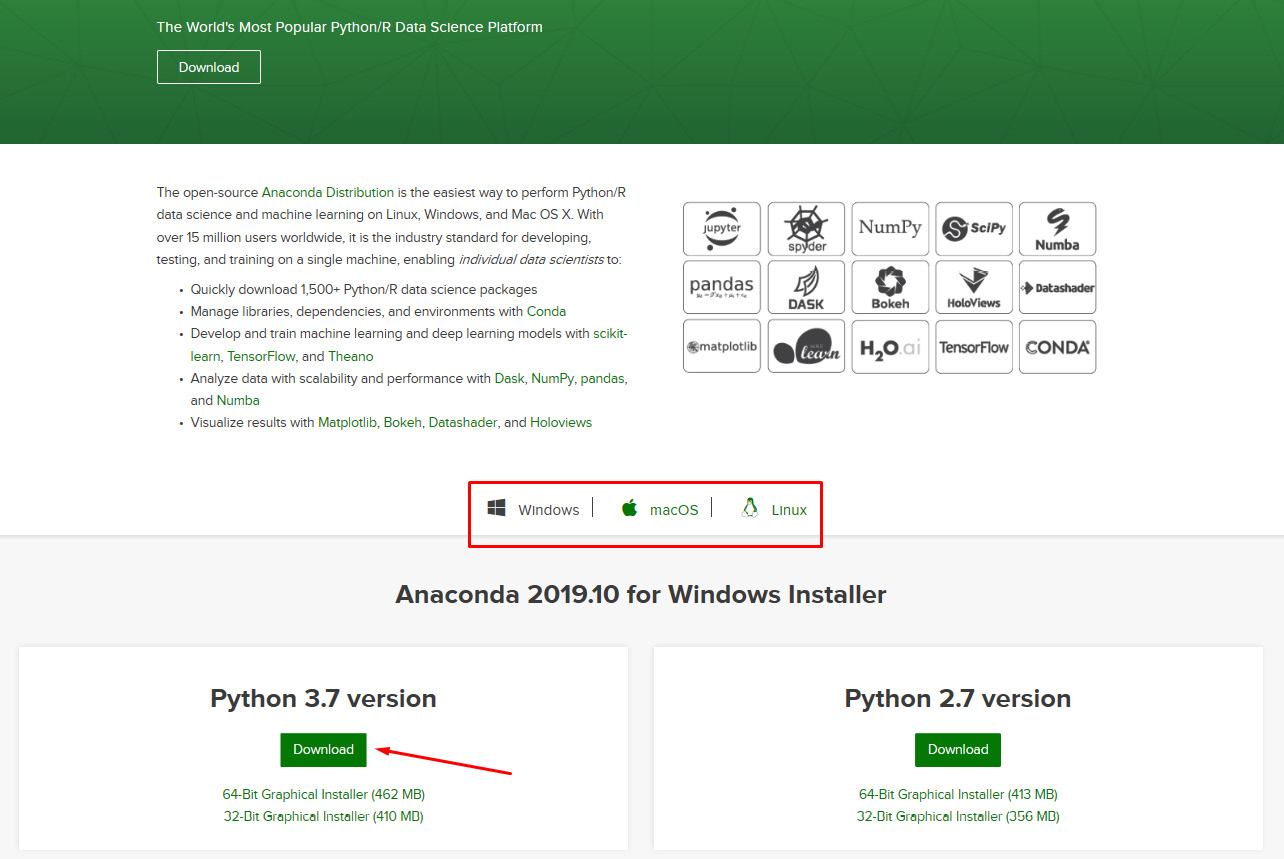
Figure 0.1: The Anaconda homescreen
Launching the Jupyter Notebook
To Launch the Jupyter Notebook frrom the Anaconda Navigator you need to follow the mentioned steps below:
- Once you install the Anaconda Navigator you will have the following screen at your end as shown in Figure 0.2.
Figure 0.2: Anaconda installation screen
- Now, click on Launch under the Jupyter Notebook option and launch the notebook on your local system:
Figure 0.3: Jupyter notebook launch option
Congratulations! You have successfully installed Jupyter Notebook onto your system.
To Install the Python Terminal on your system
To install the Python terminal on your system, follow these steps:
- Open the following link, which is the Python community website URL: https://www.python.org/downloads/.
- Select the Operating System (Windows, macOS or Linux) you would be working on as highligthed in the following screenshot:
Figure 0.4: The Python homescreen
- Once you have downloaded the software, you need to install it.
- Have a look at the following screenshot in which we have installed the Python terminal on a Windows system. We load it through the Start menu and search for Python and click on the software.
The Python terminal will look like this:
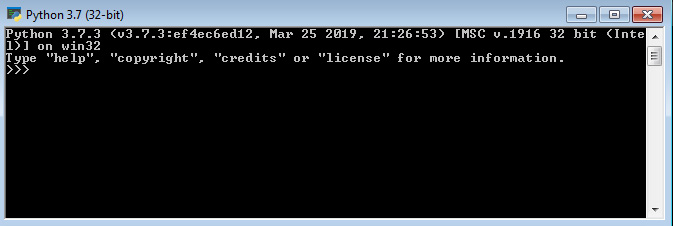
Figure 0.5: Python terminal interface
Congratulations! You have successfully installed the Python terminal onto your system.
A Few Important Packages
Some of the exercises in this chapter require the following packages:
- Matplotlib
- Seaborn
- NumPy
Install them by following this guide. On Windows, open up the command prompt. On macOS or Linux, open up the terminal. Type the following commands:
pip install matplotlib seaborn numpy
If you prefer to use Anaconda to manage your packages, type in the following:
conda install matplotlib seaborn numpy
To install Docker
- Head to https://docs.docker.com/docker-for-windows/install/ to install Docker for Windows.
- Head to https://docs.docker.com/docker-for-mac/install/ to install Docker for macOS.
- Head to https://docs.docker.com/v17.12/install/linux/docker-ce/ubuntu/ to install Docker on Linux.
If you have any issues or questions about installation please email us at [email protected]
.
Installing the Code Bundle
Download the code files from GitHub at https://packt.live/2PfducF and place them in a new folder called C:\Code
on your local system. Refer to these code files for the complete code bundle.