So far, we have only dealt with freestanding classes, let us now put three classes together in a class hierarchy. We start with the baseclass Person
, which has a constructor that initializes the field m_stName
, and a method Print
that writes the name. Print
is marked as virtual; this means that dynamic binding will come into effect when we, in the main
function, define a pointer to an object of this class. We do not have to mark the methods as virtual in the subclasses, it is sufficient to do so in the baseclass. The constructors of a class cannot be virtual. However, every other member, including the destructor, can be virtual and I advise you to always mark them as virtual in your baseclass. Non-virtual methods are not a part of the object-oriented model and were added to C++ for performance reasons only.
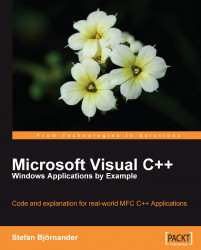
Microsoft Visual C++ Windows Applications by Example
By :
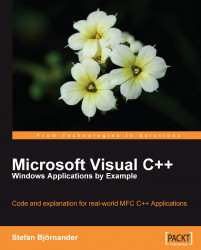
Microsoft Visual C++ Windows Applications by Example
By:
Overview of this book
Table of Contents (15 chapters)
Microsoft Visual C++ Windows Applications by Example
Credits
About the Author
About the Reviewer
Preface
Introduction to C++
Object-Oriented Programming in C++
Windows Development
Ring: A Demonstration Example
Utility Classes
The Tetris Application
The Draw Application
The Calc Application
The Word Application
Index
Customer Reviews