As far as I remember, I came across the term scaffolding (available at http://en.wikipedia.org/wiki/Scaffold_(programming)) while reading about Ruby on Rails framework. Scaffolding generates the boilerplate code for us, thereby reducing the grunt work we have to do. For example, it might generate a controller, some views, and the database table based on a model; or it might generate an agreed upon (based on the best practices for that language or framework) folder structure for us at the start of a project.
Yeoman (available at http://yeoman.io/) is a scaffolding tool for writing modern web applications. It is available as an NPM package, so you can install it globally (for a machine) using NPM as follows:
npm install –g yo
The Yeoman workflow comprises of three tools (which help us automate the three required tasks as discussed previously):
Yeoman also provides a generator ecosystem. As per the Yeoman website, "A generator is basically a plugin that can be run with the yo
command to scaffold complete projects or their useful parts." So, for example, there are generators for scaffolding an AngularJS, Backbone, or Ember application. The different generators available are listed at http://yeoman.io/generators/.
You can install the Angular generator using the following command:
npm install -g generator-angular
Once this is installed, there are commands for generating an Angular controller, directive, view, service, and so on. Let's generate a new Angular application now. Make a new directory and cd
into it and run the following command:
yo angular bookExamples
When we run the preceding command, we are asked to choose from several options such as whether we want to use Sass (with Compass) or include Bootstrap, and then we are asked to choose between different modules (as shown in the following screenshot). So you can see the choices I made here (an (*
) symbol means you need to include the module, otherwise exclude it):
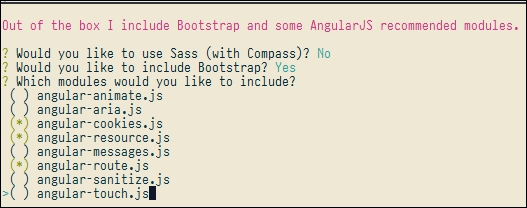
After making our choices, when we press Enter, the angular generator generates a directory structure which looks as shown in the following two screenshots. The preceding command also runs bower install
and npm install
internally (which we can also run separately).
Notice the following important points about the first screenshot:
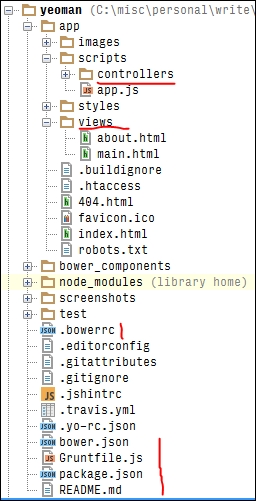
Folders and files generated by the yo angular command.
The root folder has the app
, node_modules
, and test
folders.
The app
folder has scripts
, views
, and styles
folders.
The scripts
folder has a controllers
folder.
The root
folder also has the bower.json
, Gruntfile.js
, and package.json
files.
Let's look at the contents and purpose of some of the preceding files:
{ "name": "book-examples", "version": "0.0.0", "dependencies": { "angular": "^1.3.0", "bootstrap": "^3.2.0", "angular-cookies": "^1.3.0", "angular-resource": "^1.3.0", "angular-route": "^1.3.0" }, "devDependencies": { "angular-mocks": "^1.3.0" }, "appPath": "app", "moduleName": "bookExamplesApp" }
(bower.json
)
As you know by now bower is a dependency management tool, so this JSON file lists all the dependencies under the dependencies
key. There are some dependencies like the one on angular-mocks
, which are only required during development (which we don't ship), so they are grouped under the devDependencies
key. Also notice that the name of the main module is bookExamplesApp
as specified by the value of the moduleName
key in the preceding table. Please remember that we ran the angular-generator using the bookExamples
value. Let's check the contents of the .bowerrc
file, as shown in the following code:
{ "directory": "bower_components" }
(.bowerrc
)
This file specifies the folder where bower dependencies should be placed. So as shown in the preceding file, the bower dependencies are stored in the bower_components
folder. Let's look at a part of the package.json
file:
{ "name": "bookexamples", "version": "0.0.0", "dependencies": {}, "repository": {}, "devDependencies": { "grunt": "^0.4.5", "grunt-autoprefixer": "^2.0.0", "grunt-contrib-cssmin": "^0.12.0", "grunt-contrib-htmlmin": "^0.4.0", "grunt-contrib-imagemin": "^0.9.2", "grunt-contrib-jshint": "^0.11.0", "grunt-contrib-uglify": "^0.7.0", "grunt-contrib-watch": "^0.6.1", "grunt-karma": "^0.10.1", "grunt-ng-annotate": "^0.9.2", "grunt-svgmin": "^2.0.0", "jasmine-core": "^2.2.0", "karma": "^0.12.31", "karma-jasmine": "^0.3.5", "karma-phantomjs-launcher": "^0.1.4", "load-grunt-tasks": "^3.1.0", "time-grunt": "^1.0.0" }, "engines": { "node": ">=0.10.0" }, "scripts": { "test": "grunt test" } }
(package.json
)
This file again lists dependencies
and devDependencies
, and these dependencies are for various npm
modules.
Bower and NPM are both used for managing dependencies. However, Bower manages dependencies for the frontend libraries/components (such as Bootstrap, UnderscoreJS, and so on) whereas NPM does the same for various Node.js modules.
Take a look at the second screenshot shown here:
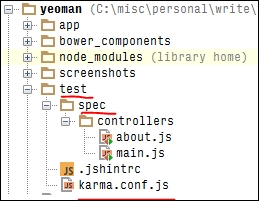
Files and folders for unit testing generated by the yo angular command.
The root
folder has a test
folder.
The test
folder has a spec
folder, and the spec
folder has a controllers
folder.
The test
folder also has a karma.conf.js
file. Karma (available at https://github.com/karma-runner/karma) is a test runner for JavaScript and can run tests written in Jasmine (available at http://jasmine.github.io/), Mocha (http://mochajs.org/), and so on.
For any UI application, we can write two types of tests—unit tests and end-to-end (E2E) tests, where the tests actually spin up a UI and interact with it as a user would. For the unit testing Angular code (available at https://docs.angularjs.org/guide/unit-testing), we can use any of the existing JS unit test frameworks such as Jasmine, Mocha, and so on. However, for E2E testing (available at https://docs.angularjs.org/guide/e2e-testing), Angular recommends Protractor (available at http://angular.github.io/protractor/#/), which uses Jasmine for its test syntax. Once we have written the tests, we need a test runner which can run these tests. A good test runner is one which can be configured to run automatically (from continuous integration scripts). So, Karma is the recommended test runner for Angular applications.