In modern software development, it goes without saying that you will need some SCM (Software Configuration Management) software to keep track of all changes in your code. Today, the most-used tool is Git, which we'll also be using. Git was created in 2005 by Linus Torvalds (who also created Linux!) for the development of the Linux kernel; not a small task considering that its source is over 25 million lines of code!
We won't be delving into how Git works, what commands to use, and so on, because that would be material enough for a book! We will focus on how to use Git with VSC. This is rather simple because not only was VSC written with Git access in mind, but there are also some extensions that can make work even easier, so you don't have to memorize lots of commands and options; take look at following illustration:
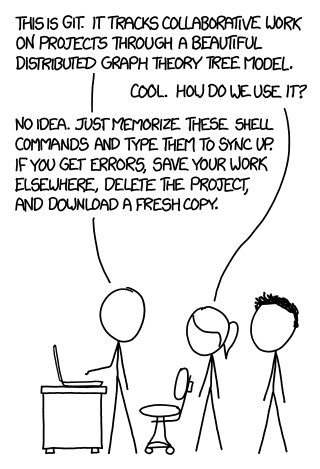
This XKCD comic is available online at https://xkcd.com/1597/.