Routing in Blazor WebAssembly
In Blazor WebAssembly, routing is handled on the client, not on the server. As you navigate in the browser, Blazor intercepts that navigation and renders the component with the matching route.
The URLs are resolved relative to the base path that is specified in the wwwroot/index.html
file. It is specified in the head
element using the following syntax:
<base href="/" />
Unlike other frameworks that you may have used, the route is not inferred from the location of its file. For example, in the Demo
project, the Counter
component is in the /Pages/Counter
folder, yet it uses the following route:
@page "/counter"
Route parameters
The Router
component uses route parameters to populate the parameters of the corresponding component. The parameters of both the component and the route must have the same name, but they are not case sensitive.
Since optional route parameters are not supported, you may need to provide more than one @page
directive to a component to simulate optional parameters. The following example shows how to include multiple @page
parameters:
RoutingExample.razor
@page "/routing" @page "/routing/{text}" <h1>Blazor WebAssembly is @Text!</h1> @code { [Parameter] public string Text { get; set; } protected override void OnInitialized() { Text = Text ?? "fantastic"; } }
In the preceding code, the first @page
directive allows navigation to the component without a parameter and the second @page
directive allows a route parameter. If a value for text
is provided, it is assigned to the Text
property of the component. If the Text
property of the component is null
, it is set to fantastic
.
The following URL will route the user to the RoutingExample
component:
/routing
The following URL will also route the user to the RoutingExample
component, but this time the Text
parameter will be set by the route:
/routing/amazing
This screenshot shows the results of using the indicated route:

Figure 2.3 – RoutingExample component
Important note
Route parameters are not case sensitive.
Catch-all route parameters
Catch-all route parameters are used to capture paths across multiple folder boundaries. This type of route parameter is a string
type and can only be placed at the end of the URL.
This is a sample component that uses a catch-all route parameter:
CatchAll.razor
@page "/{*path}" <h1>Catch All</h1> Route: @Path @code { [Parameter] public string Path { get; set; } }
For the /error/type/3
URL, the preceding code will set the value of the Path
parameter to error/type/3
:
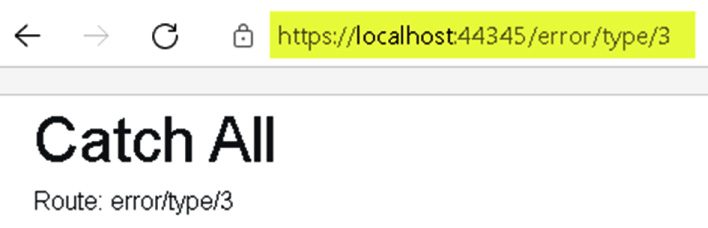
Figure 2.4 – Catch-all route parameter example
Route constraints
Route constraints are used to enforce the datatype of a route parameter. To define a constraint, add a colon followed by the constraint type to the parameter. In the following example, the route is expecting a route parameter named Increment
with the type of int
:
@page "/counter/{increment:int}"
The following route constraints are supported:
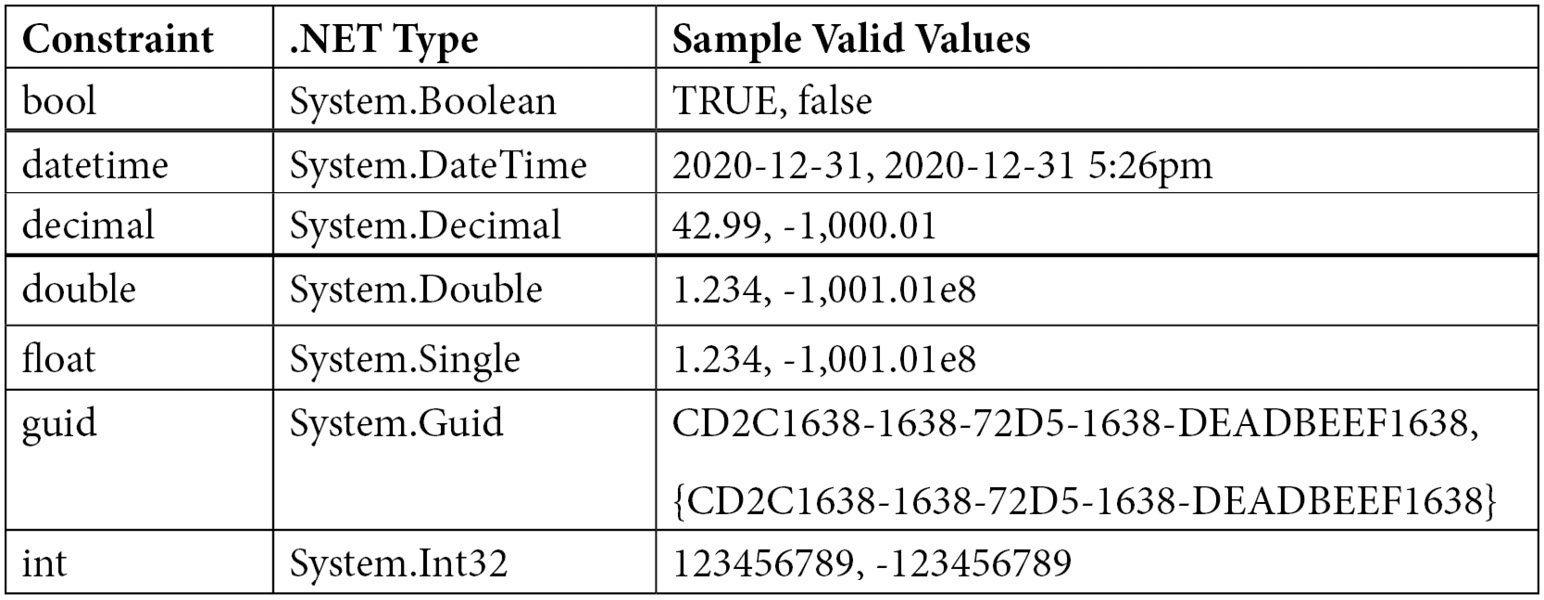
Figure 2.5 – Supported route constraints
The following types are not currently supported as constraints:
- Regular expressions
- Enums
- Custom constraints
Routing is handled on the client. We can use both route parameters and catch-all route parameters to enable routing. Route constraints are used to ensure that a route parameter is of the required datatype. Razor components use Razor syntax to seamlessly merge HTML with C# code, which is what we will see in the next section.