Using functions
A function is used to give a name to a block of instructions so that it can be used in different places in the program. In general, in a function, we group a set of instructions that are used to carry out a particular task, for example:
- Display the list of the first 10 integers.
- Calculate the sum of the first 10 numbers (from 0 to 9).
- Calculate the sum of the first N numbers (from 0 to N-1). In this case,
N
would be a parameter of the function because it can change with each call (or use) of the function.
The functions described above are very simple but show that the role of functions is to encapsulate any process by summarizing in one sentence what is expected of this process. The name given to the function symbolizes the action expected in return, which allows the developer to easily understand the sequence of instructions (including for an external developer who has not participated in the development). Let’s discuss the three functions we listed one by one.
Function displaying the list of the first 10 integers
Let’s write the first function, which displays the list of the first 10 integers. We will call this function display_10_first_integers()
. The name must be as explicit as possible because a JavaScript program is composed of many functions whose names must be unique in the program (if two function names are the same, only the last one is taken into account because it overwrites the former).
A function is defined using the keyword function
, followed by the name of the function, followed by parentheses. Then, we indicate in the braces that follow the instructions that make up the function. It is this instruction block that will be executed each time the function is called in the program.
Let’s write the function display_10_first_integers()
, which displays the first 10 integers:
Display first 10 integers with a function (testnode.js file)
function display_10_first_integers() { for (var i=0; i <= 10; i++) console.log("i = " + i); }
The function is defined using the function
keyword, followed by the function name and parentheses.
The function statements are grouped in the block that follows between the braces. We find as instructions the previous for()
loop, but it could also be the while()
loop, which works in the same way.
Let’s run this program assuming it’s included in the testnode.js
file:
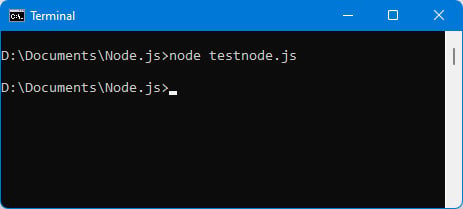
Figure 1.18 – Using a function to display numbers from 1 to 10
As we can see in the preceding figure, the screen remains blank as no display is registered in the console.
Indeed, we have simply defined the function, but we must also use it, that is, call it in our program. You can call it as many times as you want – this is the purpose of functions: we should be able to call (or use) them at any time. But it must be done at least once; otherwise, it is useless, as seen in the preceding figure.
Let’s add the function call following the function definition:
Definition and call of the function
// function definition function display_10_first_integers() { for (var i=0; i <= 10; i++) console.log("i = " + i); } // function call display_10_first_integers();
The result of the preceding code can be seen in the following figure:
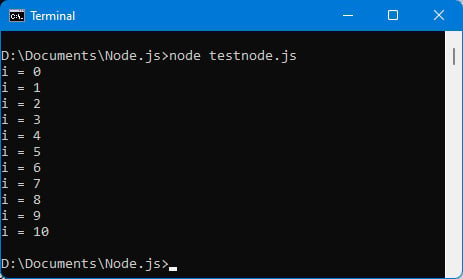
Figure 1.19 – Call of the display_10_first_integers() function
Interestingly, the function can be called in several places of the program. Let’s see how in the following example:
Successive calls to the display_10_first_integers() function
// function definition function display_10_first_integers() { for (var i=0; i <= 10; i++) console.log("i = " + i); } // function call console.log("*** 1st call *** "); display_10_first_integers(); console.log("*** 2nd call *** "); display_10_first_integers(); console.log("*** 3rd call *** "); display_10_first_integers();
In the preceding code, the function is called three times in succession, which displays the list of the first 10 integers as many times. The order of the calls is indicated before each list as follows:
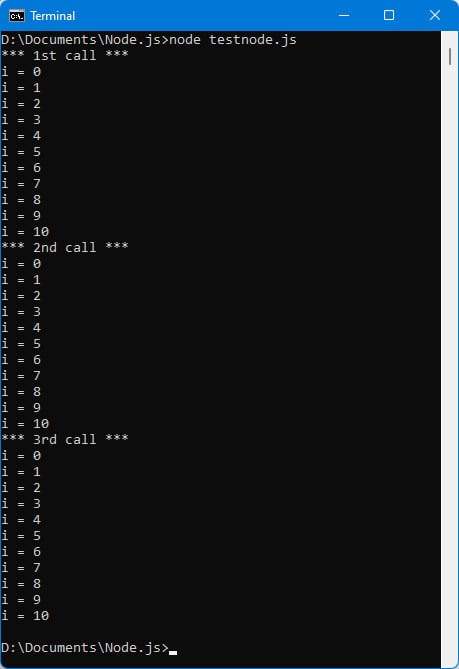
Figure 1.20 – Successive calls to the display_10_first_integers() function
Function calculating the sum of the first 10 integers
We now want to create a function that calculates the sum of the first 10 integers, that is, 1+2+3+4+5+6+7+8+9+10
. The result is 55
. This will allow us to show how a function can return a result to the outside (that is, to the program that uses it). Here, the function should return 55
.
Let’s call the function add_10_first_integers()
. This can be written as follows:
Function that adds the first 10 integers
// function definition function add_10_first_integers() { var total = 0; for (var i = 0; i <= 10; i++) total += i; return total; } // function call var total = add_10_first_integers(); console.log("Total = " + total);
We define the total
variable in the function. This variable is a local variable to the function because it is defined using the var
or let
keyword. This allows this total
variable to not be the same as the one defined outside the function, even if the names are the same.
Note
If the total
variable in the function was not defined using the var
or let
keyword, it would create a so-called global variable that would be directly accessible even outside the function. This is not good programming because you want to use global variables as little as possible.
The function uses a for()
loop to add the first 10 integers, then returns that total using the return
keyword. This keyword makes it possible to make accessible, outside the function, the value of any variable, in our example, the total
variable.
Let’s run the previous program. We should see the following output:
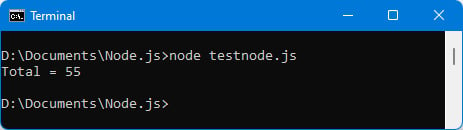
Figure 1.21 – Calculation of the sum of the first 10 integers
Function calculating the sum of the first N integers
The previous function is not very useful because it always returns the same result. A more useful function would be to calculate the sum of the first N
integers, knowing that N
can be different each time the function is called.
N
would in this case be a parameter of the function. Its value is indicated in parentheses when using the function.
Let’s call the add_N_first_integers()
function to calculate this sum. The N
parameter would be indicated in parentheses following the function name. A function can use several parameters, and it suffices to indicate them in succession, separated by a comma. In our example, a single parameter is enough.
Let’s write the add_N_first_integers(n)
function and use that to calculate the sum of the first 10, then 25, then 100 integers. The values 10, 25, and 100 will be used as parameters during successive calls to the function and will replace the parameter n
indicated in the definition of the function:
Function that adds the first N integers
// function definition function add_N_first_integers(n) { var total = 0; for (var i = 0; i <= n; i++) total += i; return total; } // calculation of the first 10 integers var total_10 = add_N_first_integers(10); console.log("Total of the first 10 integers = " + total_10); // calculation of the first 25 integers var total_25 = add_N_first_integers(25); console.log("Total of the first 25 integers = " + total_25); // calculation of the first 100 integers var total_100 = add_N_first_integers(100); console.log("Total of the first 100 integers = " + total_100);
The add_N_first_integers(n)
function is very similar to the add_10_first_integers()
function written earlier. It uses the parameter n
indicated between the parentheses and does not loop from 0
to 10
as before, but from 0
to n
. Depending on the value of n
that will be used when calling the function, the loop will thus be different, and the result returned by the function as well.
When calling the function, it passes the parameters 10
, 25
, then 100
as desired. The result is returned by the function’s total
variable, and then used by the total_10
, total_25
, and total_100
variables outside the function:
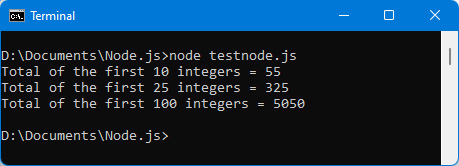
Figure 1.22 – Calculation of the sum of the first 10, then 25, then 100 integers