When you specify a Tkinter variable as a textvariable
for a widget (textvariable = myvar)
, the widget automatically gets updated whenever the value of the variable changes. However, there might be times when, in addition to updating the widget, you need to do some extra processing at the time of reading or writing (or modifying) the variable.
Tkinter provides a method to attach a callback method that would be triggered every time the value of a variable is accessed. Thus, the callback acts as a variable observer
. The callback method is named trace_variable(self, mode, callback)
, or simply trace(self, mode, callback)
.
The mode argument can take any one of 'r'
, 'w'
, 'u'
values, which stand for read, write, or undefined. Depending upon the mode specifications, the callback method is triggered if the variable is read or written.
The callback method gets three arguments by default. The arguments in order of their position are:
Name of the Tkinter variable
The index of the variable, if the Tkinter variable is an array, else an empty string
The access modes (
'w'
, 'r'
, or 'u'
)
Note that the triggered callback function may also modify the value of the variable. This modification does not, however, trigger any additional callbacks.
Let's see a small example of variable tracing in Tkinter, where writing into the Tkinter variable into an entry widget triggers a callback function (refer to the 8.01 trace variable.py
Python file available in the code bundle):
from Tkinter import * root = Tk() myvar = StringVar() def trace_when_myvar_written(var,indx,mode): print"Traced variable %s"%myvar.get() myvar.trace_variable("w", trace_when_myvar_written) Label(root, textvariable=myvar).pack(padx=5, pady=5) Entry(root, textvariable=myvar).pack(padx=5, pady=5) root.mainloop()
The description of the preceding code is as follows:
This code creates a trace variable on the Tkinter variable
myvar
in the write ("w"
) modeThe trace variable is attached to a callback method named
trace_when_myvar_written
(this means that every time the value ofmyvar
is changed, the callback method will be triggered)
Now, every time you write into the entry widget, it modifies the value of myvar
. Because we have set a trace on myvar
, it triggers the callback method, which in our example, simply prints the new value into the console.
The code creates a GUI window similar to the one shown here:
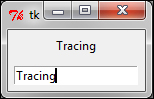
It also produces a console output in IDLE, which shows like the following once you start typing in the GUI window:
Traced variable T Traced variable Tr Traced variable Tra Traced variable Trac Traced variable Traci Traced variable Tracin Traced variable Tracing