We created a "bare bone" GUI framework in order to avoid repetition of the code that creates widgets. Similar to this concept, there is another way to avoid writing boilerplate code by using what are named
custom GUI mixins. Take for example, the code of 8.13 creating custom mixins.py
. This program creates an interface similar to the one shown here:
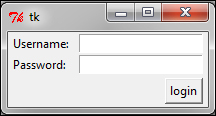
Let's look at the code of 8.13 creating custom mixins.py
:
from Tkinter import * def frame(parent, row, col): widget = Frame(parent) widget.grid(row= row, column=col) return widget def label(parent, row, col, text): widget = Label(parent, text=text) widget.grid(row=row, column=col, sticky='w', padx=2) return widget def button(parent, row, col, text, command): widget = Button(parent, text=text, command=command) widget.grid(row= row, column=col, sticky='e', padx=5, pady=3) return widget def entry(parent, row, col, var): widget = Entry(parent,textvariable= var) widget.grid(row= row, column=col, sticky='w', padx=5) return widget def button_pressed(uname, pwd): print'Username: %s' %uname print'Password: %s'%pwd if __name__ == '__main__': root = Tk() frm = frame(root, 0, 0) label(frm, 1, 0, 'Username:') uname= StringVar() entry(frm, 1, 1, uname) label(frm, 2, 0, 'Password:') pwd= StringVar() entry(frm, 2, 1, pwd) button(frm, 3, 1, 'login', lambda: button_pressed(uname.get(), pwd.get()) ) root.mainloop()
The description of the preceding code is as follows:
This program first creates functions for different widgets, such as Frame, Label, Button, and Entry. Each method can be named a mixin, because it takes care of both widget creation and its geometry management using the grid method. These are essentially convenience functions to help us avoid writing similar code for a similar set of widgets.
Now, in the main section of the program, we can create a widget in a single line of code without having to add a separate line for handling its geometry. The end result of this is fewer lines of code in our actual program. This strategy can reduce the size of your program by many lines if there are a large number of widgets in your program.
Note
However, mixins are highly case specific. A mixin defined for one particular case scenario or application may not be applicable to another application. For instance, while defining the earlier mentioned mixins, we made a few assumptions, such as all our widgets will use the grid geometry manager, and similarly, buttons would stick to east and entries would stick to the west side. These assumptions may not hold for a different application.