Ethereum accounts
Accounts are the main building blocks for the Ethereum ecosystem. It is an interaction between accounts that Ethereum wants to store as transactions in its ledger. There are two types of accounts available in Ethereum – externally owned accounts and contract accounts. Each account, by default, has a property named balance
that helps in querying the current balance of Ether.
Externally owned accounts
Externally owned accounts are accounts that are owned by people on Ethereum. Accounts are not referred to by name in Ethereum. When an externally owned account is created on Ethereum by an individual, a public/private key is generated. The private key is kept safe with the individual while the public key becomes the identity of this externally owned account. This public key is generally of 256 characters; however, Ethereum uses the first 160 characters to represent the identity of an account.
If Bob, for example, creates an account on an Ethereum network, whether private or public, he will have his private key available to himself while the first 160 characters of his public key will become his identity. Other accounts on the network can then send Ether or other cryptocurrencies based on Ether to this account.
An account on Ethereum looks like the one shown in the following screenshot:

Figure 1.8 – An externally owned account identifier
An externally owned account can hold Ether in its balance
and does not have any code associated with it. It can execute transactions with other externally owned accounts, and it can also execute transactions by invoking functions within contracts.
Contract accounts
Contract accounts are very similar to externally owned accounts. They are identified using their public address. They do not have a private key. They can hold Ether similar to externally owned accounts; however, they contain code for smart contracts consisting of functions and state variables.
Externally owned accounts are responsible for initiating transactions and each transaction in Ethereum requires gas, which is the cost of the transaction to be provided by the sender of the transaction. Gas has a relationship to its currency known as Ether. The next section will discuss the concepts related to Ether, gas, and transactions.
Ether, gas, and transactions
Ether is the currency of Ethereum. Every activity on Ethereum that modifies its state charges Ether as a fee. And miners who are successful in generating and writing a block in a chain are also rewarded Ether. Ether can easily be converted to dollars or other currencies through crypto exchanges.
Ethereum has a metric system of denominations known as units of Ether. The smallest denomination or base unit of Ether is called wei. The following is a list of the named denominations and their value in wei, which is available at https://github.com/ethereum/web3.js/blob/0.15.0/lib/utils/utils.js#L40:
var unitMap = { 'wei' : '1' 'kwei': '1000', 'ada': '1000', 'femtoether': '1000', 'mwei': '1000000', 'babbage': '1000000', 'picoether': '1000000', 'gwei': '1000000000', 'shannon': '1000000000', 'nanoether': '1000000000', 'nano': '1000000000', 'szabo': '1000000000000', 'microether': '1000000000000', 'micro': '1000000000000', 'finney': '1000000000000000', 'milliether': '1000000000000000', 'milli': '1000000000000000', 'ether': '1000000000000000000', 'kether': '1000000000000000000000', 'grand': '1000000000000000000000', 'einstein': '1000000000000000000000', 'mether': '1000000000000000000000000', 'gether': '1000000000000000000000000000', 'tether': '1000000000000000000000000000000' };
Gas
In the previous section, it was mentioned that fees are paid using Ether for any transaction execution that leads to a state change in Ethereum. Ether is traded on public exchanges, and its price fluctuates daily. If Ether is used for paying fees, then the cost of using the same service can be high on a certain day while being low on other days. People will wait for the price of Ether to fall to execute their transactions. This is not ideal for a platform such as Ethereum. Gas helps in alleviating this problem. This is the internal currency of Ethereum. The execution and resource utilization costs are predetermined in Ethereum in terms of gas units. For each unit of gas, a price can be ascribed, known as the gas price. For example, for each unit of gas, a price of 10 gwei can be assigned. When both the number of gas units and gas price are multiplied together, it results in the gas cost. The gas price can be adjusted to a lower price when the price of Ether increases and a higher price when the price of Ether decreases.
Transactions
A transaction is an agreement between a buyer and a seller, a supplier and a consumer, or a provider and a consumer that there will be an exchange of assets, products, or services for currency, cryptocurrency, or some other asset, either in the present or in the future. Ethereum helps in executing the transaction. The following are the three types of transactions that can be executed in Ethereum:
- The transfer of Ether from one account to another: The accounts can be externally owned accounts or contract accounts. The following are the possible cases:
- An externally owned account sending Ether to another externally owned account in a transaction
- An externally owned account sending Ether to a contract account in a transaction
- A contract account sending Ether to another contract account in a transaction
- A contract account sending Ether to an externally owned account in a transaction
- Deployment of a smart contract: An externally owned account can deploy a contract using a transaction in an EVM.
- Using or invoking a function within a contract: Executing a function in a contract that changes state is considered a transaction in Ethereum. If executing a function does not change a state, it does not require a transaction.
A transaction has some of the following important properties related to it:
- The
from
account property denotes the account that originates the transaction and represents an account that is ready to send some gas or Ether. Both gas and Ether concepts were discussed earlier in this chapter. Thefrom
account can be externally owned or a contract account. - The
to
account property refers to an account that is receiving Ether or benefits in lieu of an exchange. For transactions related to the deployment of the contract, theto
field is empty. It can be externally owned or a contract account. - The
value
account property refers to the amount of Ether that is transferred from one account to another. - The
input
account property refers to the compiled contract bytecode and is used during contract deployment in an EVM. It is also used for storing data related to smart contract function calls along with their parameters. A typical transaction in Ethereum where a contract function is invoked is shown here. In the following screenshot, note theinput
field containing the function call to contract, along with its parameters:
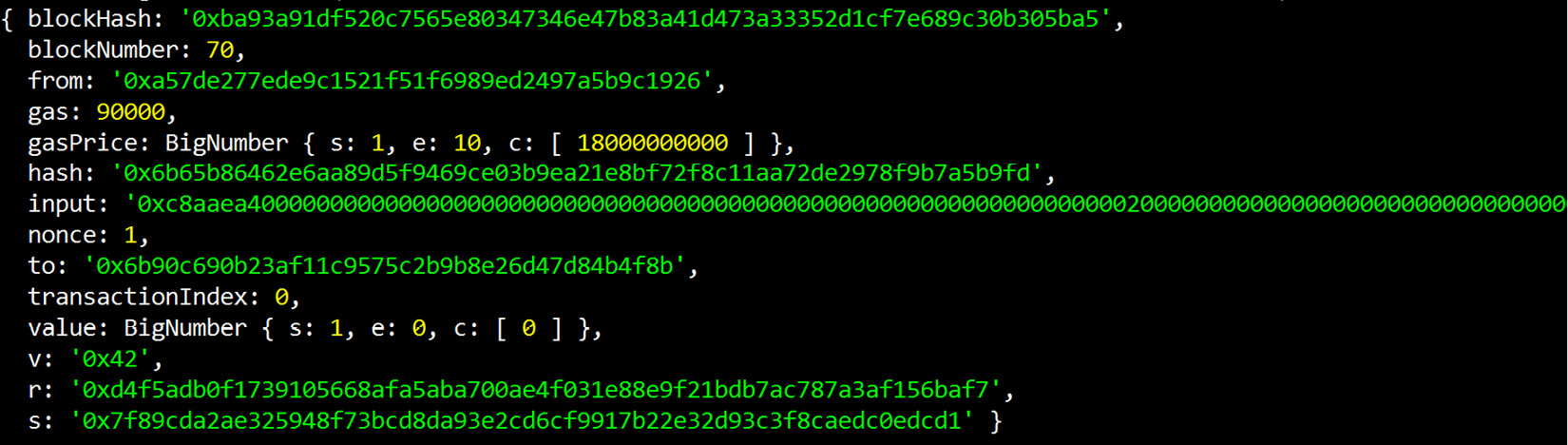
Figure 1.9 – The transaction properties in a block
- The
blockHash
account property refers to the hash of the block to which this transaction belongs. - The
blockNumber
account property is the block to which this transaction belongs. - The
gas
account property refers to the amount of gas supplied by the sender who is executing this transaction. - The
gasPrice
account property refers to the price per gas the sender was willing to pay in wei (we learned about wei in the Ether section earlier in this chapter). Total gas is computed at gas units x gas price. - The
hash
account property refers to the hash of the transaction. - The
nonce
account property refers to the number of transactions made by the sender prior to the current transaction. - The
transactionIndex
account property refers to the serial number of the current transactions in the block. - The
value
account property refers to the amount of Ether transferred in wei. - The
v
,r
, ands
account properties relate to digital signatures and the signing of the transaction.
A typical transaction in Ethereum, where an externally owned account sends some Ether to another externally owned account, is shown here. Note that the input
field is not used here. Since two Ethers were sent in the transaction, the value
field is showing the value accordingly in wei, as shown in the following screenshot:
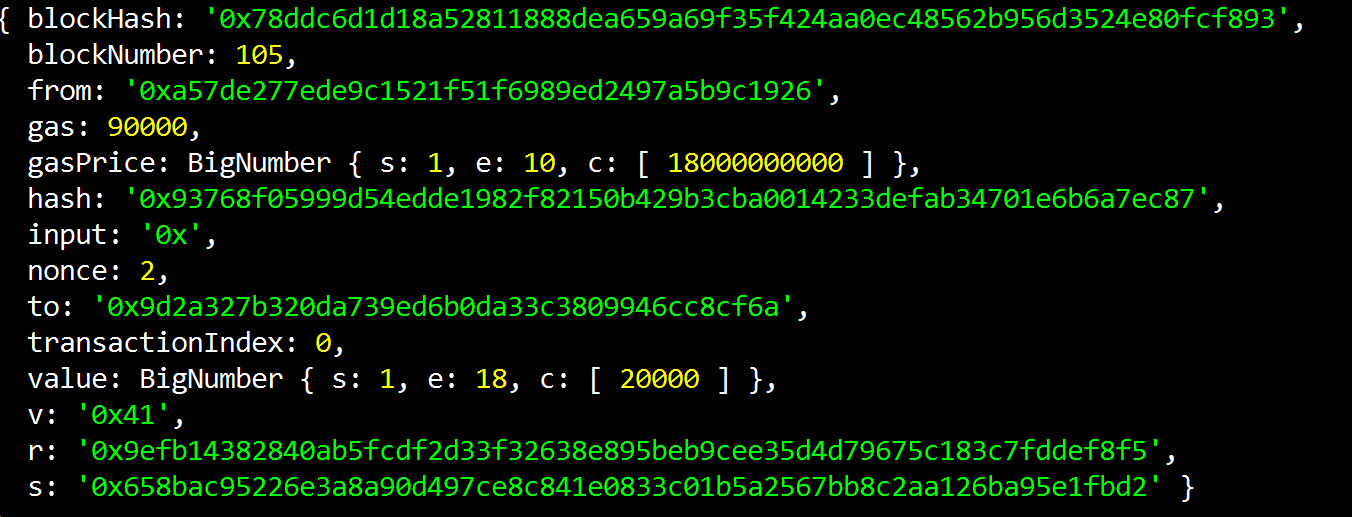
Figure 1.10 – Block properties in a block
One method to send Ether from an externally owned account to another externally owned account is shown in the following code snippet using the web3
JavaScript framework, which will be covered later in this book:
web.eth.sendTransaction({from: web.eth.accounts[0], to: "0x9d2a327b320da739ed6b0da33 c3809946cc8cf6a", value: web. toWei(2, 'ether')})
A typical transaction in Ethereum where a contract is deployed is shown in the following screenshot. In the following screenshot, note the input
field containing the bytecode of the contract:
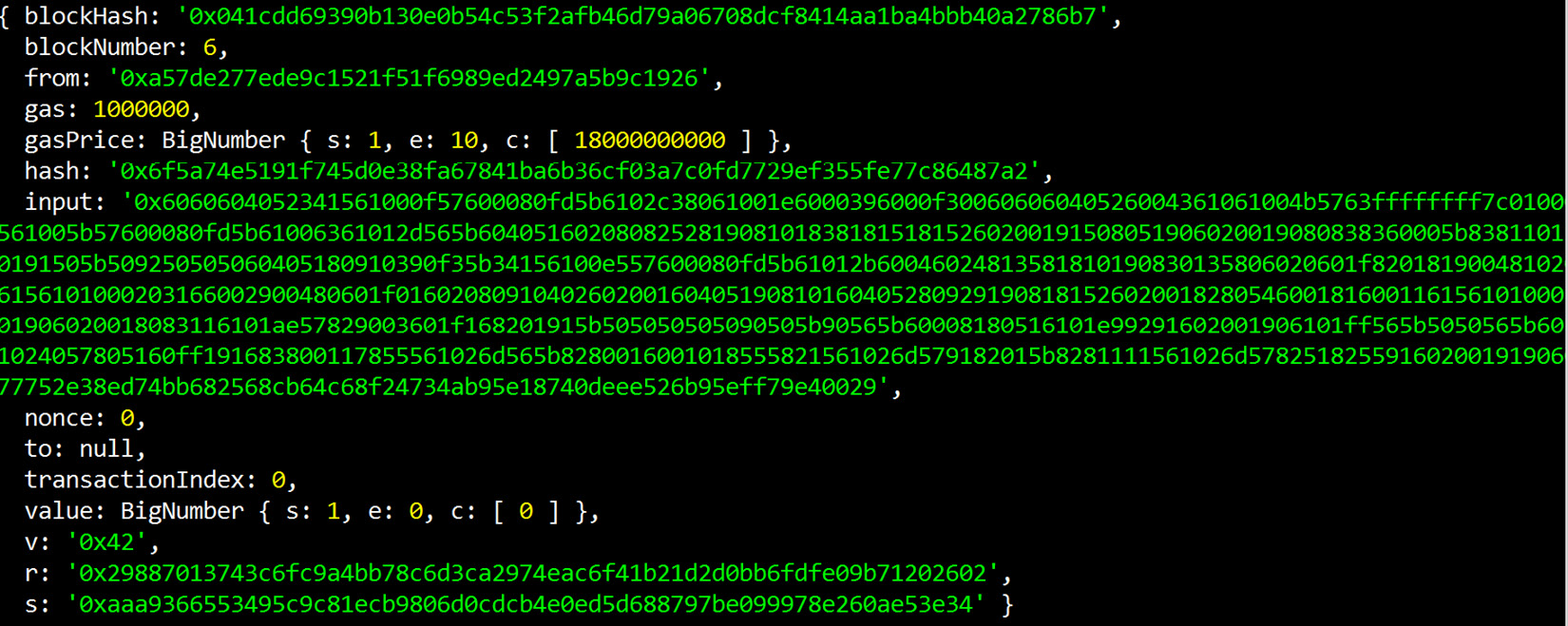
Figure 1.11 – A sample transaction in a block