These classes are present in the playable portion of the game:
MagneTank's ManagedGameScene
class builds upon the ManagedGameScene
class presented in Chapter 5, Scene and Layer Management, by adding a stepped loading screen to show what the game is loading for each level. The idea behind using loading steps is the same as showing a loading screen for one frame before loading the game, much like how the SceneManager
class functions when showing a new scene, but the loading screen is updated for each loading step instead of just once at the first showing of the loading screen.
This class is based on the following recipes:
The GameLevel
class brings all of the other in-game classes together to form the playable part of MagneTank. It handles the construction and execution of each actual game level. It extends a customized ManagedGameScene
class that incorporates a list of LoadingRunnable
objects, which create the level in steps that allow each progression of the level construction to be shown on the screen. The GameLevel
class also determines the completion or failure of each game level using the GameManager
class to test for win or lose conditions.
This class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Applying text to a layer in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Using modifiers and entity modifiers in Chapter 2, Working with Entities
Using parallax backgrounds to create perspective in Chapter 3, Designing Your Menu
Introducing the camera object in Chapter 4, Working with Cameras
Limiting camera area with the bound camera in Chapter 4, Working with Cameras
Taking a closer look with zoom cameras in Chapter 4, Working with Cameras
Applying a HUD to the camera in Chapter 4, Working with Cameras
Customizing managed scenes and layers in Chapter 5, Scene and Layer Management
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers
Creating sprite pools in Chapter 8, Maximizing Performance
The LoadingRunnable
class acts as a Runnable
object while also updating the loading screen in the ManagedGameScene
class. An ArrayList
type of LoadingRunnable
objects is present in each ManagedGameScene
class to give the developer as much or as little control over how much loading progression is shown to the player. It is important to note that, while the updating of the loading screen is not processor-intensive in MagneTank, a more complicated, graphically complex loading screen may take a large toll on the loading times of eah level.
The Levels
class holds an array of all of the levels that can be played in the game as well as helper methods to retrieve specific levels.
The BouncingPowerBar
class displays a bouncing indicator to the player that indicates how powerful each shot from the vehicle will be. It transforms the visible location of the indicator to a fractional value, which then has a cubic curve applied to add even more of a challenge when trying to achieve the most powerful shot. The following image shows what the power bar looks like after being constructed from three separate images:
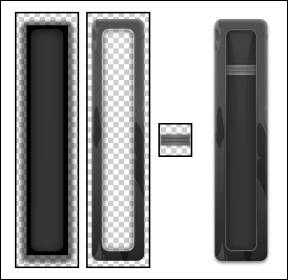
The BouncingPowerBar
class is based on the following recipes:
The MagneTank
class creates and controls the vehicle that the game is based on. It pieces together Box2D bodies using joints to create the physics-enabled vehicle, and uses the player's input, via BoundTouchInputs
, to control how each part of the vehicle moves and functions. The following image shows the MagneTank before and after construction:
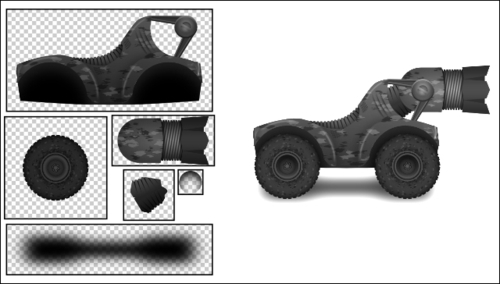
The MagneTank
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Using relative rotation in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Limiting camera area with the bound camera in Chapter 4, Working with Cameras
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Creating unique bodies by specifying vertices in Chapter 6, Applications of Physics
Using forces, velocities, and torque in Chapter 6, Applications of Physics
Working with joints in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers
Applying a sprite-based shadow in Chapter 10, Getting More From AndEngine
The MagneticCrate
class extends the MagneticPhysObject
class. It creates and handles the various types of crates available to launch from the MagneTank vehicle. Each crate is displayed in the form of a tiled sprite, with the tiled sprite's image index set to the crate's type. The MagneticCrate
class makes use of Box2D's postSolve()
method from the physics world's ContactListener
. The following image shows the various sizes and types of crates available in the game:
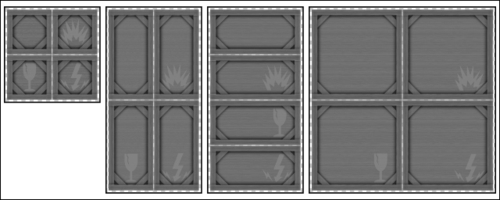
The MagneticCrate
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Using preSolve and postSolve in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers
The MagneticOrb
class creates a visual effect around MagneTank's current projectile. It rotates two swirl images (see the following image) in opposite directions to give the illusion of a spherical force. The MagneticOrb
class forms and fades as projectiles are loaded and shot.
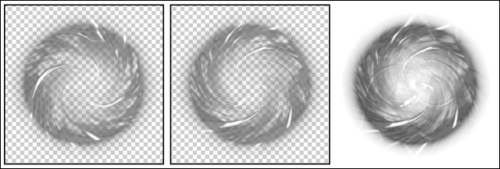
The MagneticOrb
class is based on the following recipes:
The MagneticPhysObject
class extends the PhysObject
class to allow an object to be grabbed, or released, by the MagneTank vehicle. When grabbed, the object has anti-gravity forces applied as well as forces that pull the object toward the MagneTank turret.
The MagneticPhysObject
class is based on the following recipes:
Introduction to the Box2D physics extension in Chapter 6 , Applications of Physics
Understanding different body types Chapter 6 Applications of Physics
Using forces, velocities, and torque Chapter 6 Applications of Physics
Applying anti-gravity to a specific body Chapter 6 Applications of Physics
What are update handlers? Chapter 6 Working with Update Handlers
The MechRat
class extends the PhysObject
class to take advantage of the postSolve()
method that gets called when it collides with another physics-enabled object. If the force is great enough, MechRat is destroyed, and previously loaded particle effects are immediately shown. MechRat also has wheels connected by revolute joints, which add to the challenge of destroying it. The following image shows the visual composition of MechRat:
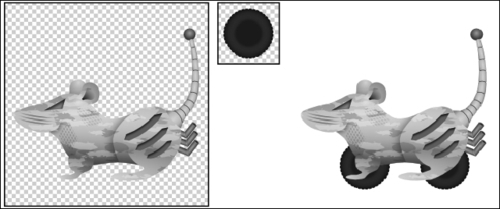
This class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Update Handlers
Bringing a scene to life with sprites in Chapter 2, Working with Update Handlers
Overriding the onManagedUpdate method in Chapter 2, Working with Update Handlers
Working with particle systems in Chapter 2, Working with Update Handlers
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Creating unique bodies by specifying vertices in Chapter 6, Applications of Physics
Working with joints in Chapter 6, Applications of Physics
Using preSolve and postSolve in Chapter 6, Applications of Physics
Creating destructible objects in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers
This class represents the non-static, physics-enabled girders seen in the game. The length of each beam can be set thanks to its repeating texture.
The MetalBeamDynamic
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Using relative rotation in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Similar to the MetalBeamDynamic
class above, this class also represents a girder, but the BodyType
option of this object is set to Static
to create an immobile barrier.
The MetalBeamStatic
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Using relative rotation in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
The ParallaxLayer
class, which was written and released by the co-author of this book, Jay Schroeder, allows for the easy creation of ParallaxEntity
objects that give the perception of depth when the Camera
object is moved across a scene. The level of parallax effect can be set, and the ParallaxLayer
class takes care of correctly rendering each ParallaxEntity
object. The following image shows the background layers of MagneTank that are attached to a ParallaxLayer
class:
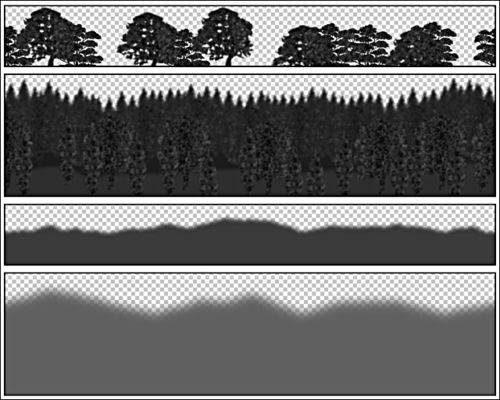
The ParallaxLayer
class is based on the following recipes:
The PhysObject
class is used in MagneTank to delegate contacts received from the physics world's ContactListener
. It also facilitates a destroy()
method to make destroying physics objects easier.
The PhysObject
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Using preSolve and postSolve in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers
The RemainingCratesBar
class gives a visual representation to the player of which crates are left to be shot by MagneTank. The size, type, and number of crates left in each level are retrieved from the GameLevel
class and vary from level to level. When one crate is shot, the RemainingCratesBar
class animates to reflect the change in the game state.
This class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Working with OpenGL in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Using modifiers and entity modifiers in Chapter 2, Working with Entities
The TexturedBezierLandscape
class creates two textured meshes and a physics body that represent the ground of the level. As the name implies, the landscape is comprised of Bezier curves to show rising or falling slopes. The textured meshes are made from repeating textures to avoid any visible seams between landscaped areas. The following image shows the two textures used to create the landscape as well as an example of how the combined meshes appear after a Bezier slope has been applied:
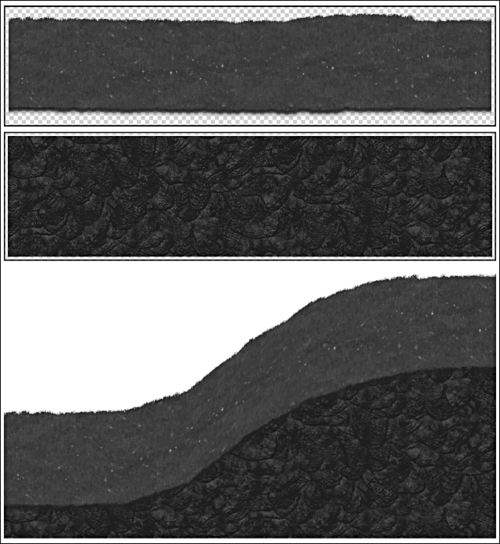
The TexturedBezierLandscape
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Working with OpenGL in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Creating unique bodies by specifying vertices in Chapter 6, Applications of Physics
Textured meshes in Chapter 10, Getting More From AndEngine
This class is the same TexturedMesh
class as found in the recipe, Textured meshes in Chapter 10, Getting More From AndEngine.
This class is similar to the MetalBeam
classes, but adds a health aspect that causes the WoodenBeamDynamic
class to be replaced with a particle effect once its health reaches zero.
The WoodenBeamDynamic
class is based on the following recipes:
Understanding AndEngine entities in Chapter 2, Working with Entities
Bringing a scene to life with sprites in Chapter 2, Working with Entities
Using relative rotation in Chapter 2, Working with Entities
Overriding the onManagedUpdate method in Chapter 2, Working with Entities
Working with particle systems in Chapter 2, Working with Entities
Introduction to the Box2D physics extension in Chapter 6, Applications of Physics
Understanding different body types in Chapter 6, Applications of Physics
Using preSolve and postSolve in Chapter 6, Applications of Physics
What are update handlers? in Chapter 7, Working with Update Handlers