We studied Grunt in Chapter 3, Improving PHP 7 Application Performance. We only used it to merge CSS and JavaScript files and minify them. However, Grunt is not used only for this purpose. It is a JavaScript task runner, which can run tasks either by watching specific files for changes or by manually running tasks. We studied how we can run tasks manually, so now we will study how to use grunt watch to run specific tasks when some changes are made.
Grunt watch is useful and saves a lot of time because it runs the specific tasks automatically instead of running the tasks manually every time we change something.
Let's recall our examples from Chapter 3, Improving PHP 7 Application Performance. We used Grunt to combine and compress CSS and JavaScript files. For this purpose, we created four tasks. One task was combining all CSS files, the second task was combining all JavaScript files, the third task was compressing the CSS files, and the fourth task was compressing all JavaScript files. It will be very time consuming if we run all these tasks manually every time we make some changes. Grunt provides a feature called watch that watches different destinations for file changes, and if any change occurs, it executes the tasks that are defined in the watch.
First, check whether the grunt watch
module is installed or not. Check the node_modules
directory and see whether there is another directory with the name grunt-contrib-watch
. If this directory is there, then watch is already installed. If the directory is not there, then just issue the following command in the terminal at the project root directory where GruntFile.js
is located:
npm install grunt-contrib-watch
The preceding command will install Grunt watch and the grunt-contrib-watch
directory will be available with the watch
module.
Now, we will modify this GruntFile.js
file to add the watch
module, which will monitor all the files in our defined directories, and if any changes occur, it will run these tasks automatically. This will save a lot of time in manually executing these tasks again and again. Look at the following code; the highlighted code is the modified section:
module.exports = function(grunt) { /*Load the package.json file*/ pkg: grunt.file.readJSON('package.json'), /*Define Tasks*/ grunt.initConfig({ concat: { css: { src: [ 'css/*' //Load all files in CSS folder ], dest: 'dest/combined.css' //Destination of the final combined file. },//End of CSS js: { src: [ 'js/*' //Load all files in js folder ], dest: 'dest/combined.js' //Destination of the final combined file. }, //End of js }, //End of concat cssmin: { css: { src : 'dest/combined.css', dest : 'dest/combined.min.css' } }, //End of cssmin uglify: { js: { files: { 'dest/combined.min.js' : ['dest/combined.js']//destination Path : [src path] } } }, //End of uglify //The watch starts here watch: { mywatch: { files: ['css/*', 'js/*', 'dist/*'], tasks: ['concat', 'cssmin', 'uglify'] }, }, }); //End of initConfig grunt.loadNpmTasks('grunt-contrib-watch'); //Include watch module grunt.loadNpmTasks('grunt-contrib-concat'); grunt.loadNpmTasks('grunt-contrib-uglify'); grunt.loadNpmTasks('grunt-contrib-cssmin'); grunt.registerTask('default', ['concat:css', 'concat:js', 'cssmin:css', 'uglify:js']); }; //End of module.exports
In preceding highlighted code, we added a watch
block. The mywatch
title can be any name. The files
block is required, and it takes an array of the source paths. The Grunt watch watches for changes in these destinations and executes the tasks that are defined in the tasks block. Also, the tasks that are mentioned in the tasks
block are already created in GruntFile.js
. Also, we have to load the watch
module using grunt.loadNpmTasks
.
Now, open the terminal at the root of the project where GruntFile.js
is located and run the following command:
grunt watch
Grunt will start watching the source files for changes. Now, modify any file in the paths defined in the files
block in GruntFile.js
and save the file. As soon as the file is saved, the tasks will be executed and the output for the tasks will be displayed in the terminal. A sample output can be seen in the following screenshot:
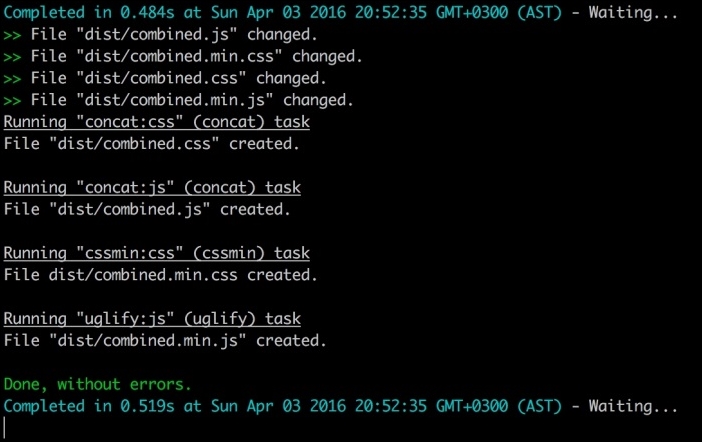
It is possible to watch as many tasks as required in the watch
block, but these tasks should be present in GruntFile.js
.