Let's now try and execute the sum() and sqrt() functions in R. Follow the steps given below:
- Open the R console on your system.
- Type the code as follows:
sum(1, 2, 3, 4, 5)
sqrt(144)
- Execute the code.
Output: The preceding code provides the following output:
Functions such as sum() and sqrt() are called base functions, which are built into R. This means that they come pre-installed when R is downloaded.
We could build all of our code right in the R console, but eventually, we might want to see our files, a list of everything in our global environment, and more, so instead, we'll use RStudio. Close R, and when it asks you to save the workspace image, for now, click Don't Save. (More explanation on workspace images and saving will come later in this chapter.)
Open RStudio. We'll use RStudio for all of our code development from here on. One major benefit of RStudio is that we can use Projects, which will organize all of the files for analysis in one folder on our computer automatically. Projects will keep all of the parts of your analysis organized in one space in a chosen folder on your machine. Any time you start a new project, you should start a new project in RStudio by going to File | New Project, as shown in the below screenshot, or by clicking the new project button (blue, with a green plus sign
).
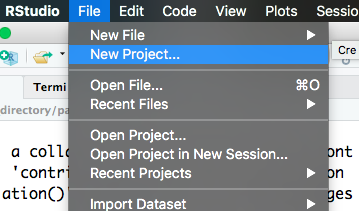
Creating a project from a New Directory allows us to create a folder on our drive (here E:\) to store all code files, data, and anything else associated with the book. If there was an existing folder on our drive that we'd like to make the directory for the project, we would choose the Existing Directory option. The Version Control option allows you to clone a repository from GitHub or another version control site. It makes a copy of the project stored on GitHub and saves it on your computer:
The working directory in R is the folder where all of the code files and output will be saved. It should be the same as the folder you choose when you create a project from a new or existing directory. To verify the working directory at any time, use the getwd() function. It will print the working directory as a character string (you can tell because it has quotation marks around it). The working directory can be changed at any time by using the following syntax:
setwd("new location/on the/computer")
To create a new script in R, navigate to File | New File | R Script, as shown in the screenshot below, or click the button on the top left that looks like a piece of paper with a green arrow on it
.
Inside New File, there are options to create quite a few different things that you might use in R. We'll be creating R scripts throughout this book.
Custom functions are fairly straightforward to create in R. Generally, they should be created using the following syntax:
name_of_function <- function(input1, input2){
operation to be performed with the inputs
}
The example custom function is as follows:
area_triangle <- function(base, height){
0.5 * base * height
}
Once the custom function code has been run, it will display in the Global Environment in the upper right corner and is now available for use in your RStudio project, as shown in the following screenshot:
One crucial step upon exiting RStudio or when you close your computer is to save a copy of the global environment. This will save any data or custom functions in the environment for later use and is done with the save.image() function, into which you'll need to enter a character string of what you want the saved file to be called, for example, introDSwR.RData. It will always be named with the extension .RData. If you were to open your project again some other time and want to load the saved environment, you use the load() function, with the same character string inside it.