The TypeScript programming language (http://www.typescriptlang.org) was created by Microsoft and was later open sourced under the Apache 2.0 license. The source code of the language is available on GitHub over at https://github.com/Microsoft/TypeScript. At the time of writing, TypeScript now has 51,705 stars and 7,116 forks on GitHub and its popularity continues to rise.
The first thing to realize is that TypeScript compiles to JavaScript. This means that the output of the TypeScript compiler can run wherever JavaScript code can run, which actually means, nowadays, basically everywhere, since JavaScript can run in the following:
- Web browser
- Backend (for example, with Node.js)
- Desktop (for example, with Electron)
- Mobile, with frameworks such as React Native, NativeScript, Ionic, and many others
- The cloud, with platforms such as Azure Functions, Google Cloud Functions, and Firebase
As stated earlier, TypeScript is compiled and not interpreted like JavaScript is. Actually, people often talk about transpilation rather than compilation in the case of TypeScript, since the TypeScript compiler actually does source-to-source transformation.
The second key point is that TypeScript is a superset of JavaScript as shown in the following diagram:
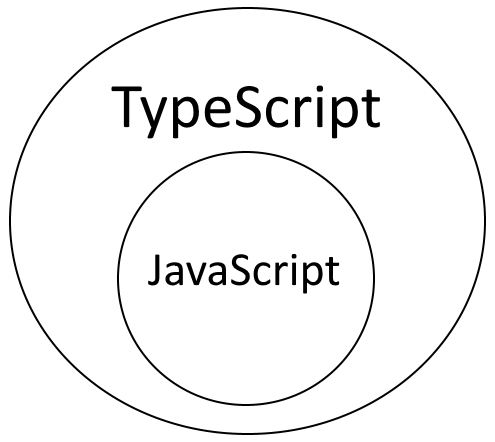
This means that any valid JavaScript code is also valid TypeScript code. This is great because it means that it is very easy to introduce TypeScript into an existing JavaScript code base. It does not stop there, though! As you'll see throughout the book, TypeScript adds a lot over vanilla JavaScript.
A third takeaway is that TypeScript is (optionally) typed. If you're familiar with JavaScript, then you probably know that it is a dynamically and weakly typed language. As any JavaScript code is also valid TypeScript code, it also means that you can declare variables without specifying their type and later assign different types to them (for example, numbers, strings, and more).
Although the fact that defining types is optional by default in TypeScript does not mean that you should avoid defining types. Instead, TypeScript shines when you make clever use of its type system.
TypeScript allows you to clearly specify the type of your variables and functions. In addition, it also has very powerful type inference, support for classes, generics, enums, mixins, and many other cool things that we’ll see throughout the book.
We'll see later in the book how to configure the TypeScript compiler to be stricter and we'll also learn the benefits of enforcing and leveraging strong typing at work, as well as for personal projects.
This quick introduction to TypeScript is useful for you to grasp what it is, but it does not explain why it exists and why it makes sense for you to use it.