As an alternative to animating using jQuery, we can use browser-supported CSS3 transitions. Using CSS3 transitions is beneficial in terms of performance because in some browsers and mobile devices, transitions are hardware-accelerated. The rendering engine of the browser can use frame skipping to keep performance smooth and also increase efficiency by reducing the update frequency of animations that are being used in hidden browser tabs.
In the code bundle for this book, open the 4-css-animation.html
file in your preferred text editor to follow along. Also, if you have not been using Google Chrome or a browser that supports CSS3 transitions until now, be sure to use one while performing the steps listed under this recipe.
Add the proper CSS3 transition properties in addition to the existing CSS properties that we have already defined for the Masonry elements.
<style> .masonry, .masonry .masonry-brick { -webkit-transition-duration: 0.5s; -moz-transition-duration: 0.5s; -ms-transition-duration: 0.5s; -o-transition-duration: 0.5s; transition-duration: 0.5s; } .masonry { -webkit-transition-property: height, width; -moz-transition-property: height, width; -ms-transition-property: height, width; -o-transition-property: height, width; transition-property: height, width; } .masonry .masonry-brick { -webkit-transition-property: left, right, top; -moz-transition-property: left, right, top; -ms-transition-property: left, right, top; -o-transition-property: left, right, top; transition-property: left, right, top; } </style>
Build the standard Masonry HTML structure and add as many Masonry items as necessary.
<div id='masonry-container'> <div class='masonry-item '> Maecenas faucibus mollis interdum. </div> <div class='masonry-item '> Maecenas faucibus mollis interdum. Donec sed odio dui. Nullam quis risus eget urna mollis ornare vel eu leo. Vestibulum id ligula porta felis euismod semper. </div> <div class='masonry-item '> Nullam quis risus eget urna mollis ornare vel eu leo. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Aenean eu leo quam. Pellentesque ornare sem lacinia quam venenatis vestibulum. </div> </div>
Add the standard Masonry functions without the
isAnimated
option.<script> $(function() { $('#masonry-container').masonry({ itemSelector : '.masonry-item', }); }); </script>
Instead of using jQuery, we assigned CSS3 transition properties to the Masonry elements. After Masonry is run, it assigns additional classes and inline styles to the elements; for example, it assigns the masonry
class to the container and the masonry-brick
class to the individual items.
We assigned the transition-duration
property to all items, the container itself and the Masonry items inside it, so that all transitions will last for half of a second. We then assigned the height and width of the container and the left / right / top positions to transition-property
. This means that whenever those values change, that is, the size of the container and the position of the Masonry items change, the transition effect will be applied to those changes.
Using CSS3 animations is great—they're efficient and they boost performance if the browser supports them. Luckily, if the browser doesn't support CSS3, we can use Modernizr and go back to using jQuery. Modernizr is a JavaScript library that detects the HTML5 and CSS3 features that are available in a user's browser, which is a great advantage.
Note
Modernizr is available for downloading at http://modernizr.com/download/. You can build your own custom Modernizr tests, such as adding CSS animations and transitions. Modernizr is available for free under the MIT license.
To enable Modernizr, we will have to include the Modernizr script into our page and then add the isAnimated
option to the Masonry script with the value !Modernizr.csstransitions
, as shown in the following code snippet:
<script src="js/modernizr.csstrans.js"></script> <script> $(function() { $('#masonry-container').masonry({ itemSelector : '.masonry-item', isAnimated: !Modernizr.csstransitions }); }); </script>
This will detect whether CSS transitions are available in our browser; if not, we will go back to using jQuery animations. In the browser, we can view information about the DOM to see whether the CSS transitions and animations are enabled. In the following screenshot, we can see that CSS transitions are enabled in Firebug for Firefox:
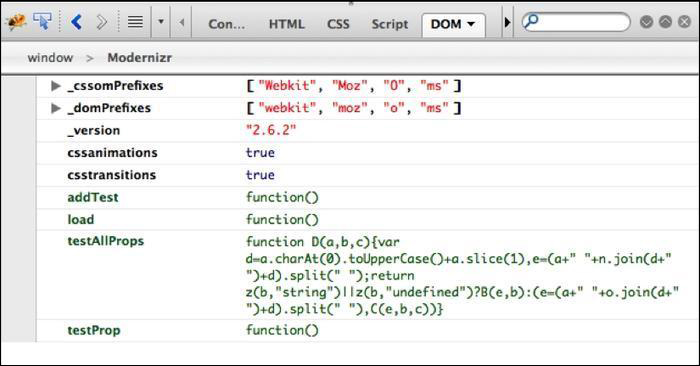
As opposed to that, in the following screenshot we can see that CSS transitions are turned off in Internet Explorer 9; we need to use jQuery to enable transitions:
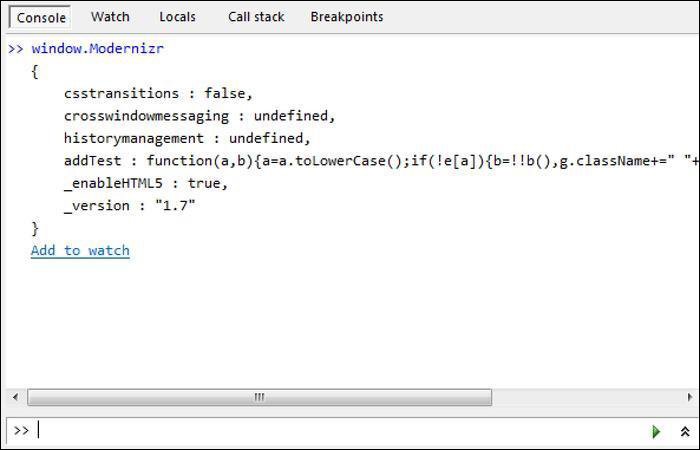