Web development has significantly evolved in recent years, particularly with the apparition of web frameworks. We will learn how to use the Django framework to create a complete website.
In this chapter, we will discuss the following:
- The changes in the Web
- A presentation of Django
- MVC development pattern
The Web that you see today has not always been as it appears today. Indeed, many technologies such as CSS, AJAX, or the new HTML 5 version have improved the Web.
The Web was born 25 years ago, thanks to growing new technologies. Two of these have been very decisive:
- The HTML language is a display language. It allows you to organize information with nested tags.
- The HTTP protocol is a communication network protocol that allows a client and a server to communicate. The client is often a browser such as Firefox or Google Chrome, and the server is very often a web server such as Nginx, Apache, or Microsoft IIS.
In the beginning, developers used the <table>
tag to organize various elements of their page as the menu, header, or content. The images displayed on the web pages were of low resolutions to avoid the risk of making the page heavy. The only action that users could perform was to click on the hypertext links to navigate to other pages.
These hypertext links enabled users to navigate from one page to another by sending only one type of data: the URL of the page. The Uniform Resource Locator (URL) defines a unique link to get resources such as an HTML page, picture, or PDF file. No data other than the URL was sent by the user.
The term Web 2.0 was coined by Dale Dougherty, O'Reilly Media Company, and was mediated in October 2004 by Tim O'Reilly during the first Web 2.0 conference.
This new Web became interactive and reachable to beginners. It came as a gift to many technologies, including the following:
- The server-side languages such as PHP, Java Server Page (JSP), or ASP. These languages allow you to communicate with a database to deliver dynamic content. This also allows users to send data in HTML forms in order to process data using the web server.
- Databases store a lot of information. This information can be used to authenticate a user or display an item list from older to more recent entries.
- Client-side script such as JavaScript enables users to perform simple tasks without refreshing the page. Asynchronous JavaScript and XML (AJAX) brings an important feature to the current Web: asynchronous swapping between the client and the server. Thanks to this, there is no need to refresh the page in order to enjoy the website.
Today, Web 2.0 is everywhere, and it is a part of our everyday life. Facebook is a perfect example of a Web 2.0 site, with complete interaction between users and the storage of massive amounts of information in its database. Web applications have been popularized as webmails or Google web applications.
It's in this philosophy that Django emerged.
Django was born in 2003 in a press agency of Lawrence, Kansas. It is a web framework that uses Python to create websites. Its goal is to write very fast dynamic websites. In 2005, the agency decided to publish the Django source code in the BSD license. In 2008, the Django Software Foundation was created to support and advance Django. Version 1.00 of the framework was released a few months later.
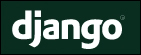
Django's slogan is explicit. This framework was created to accelerate the development phase of a site, but not exclusively. Indeed, this framework uses the MVC pattern, which enables us to have a coherent architecture, as we will see in the next chapter.
Until 2013, Django was only compatible with Python version 2.x, but Django 1.5 released on February 26, 2013, points towards the beginning of Python 3 compatibility.
Today, big organizations such as the Instagram mobile website, Mozilla.org, and Openstack.org are using Django.
A framework is a set of software that organizes the architecture of an application and makes a developer's job easier. A framework can be adapted to different uses. It also gives practical tools to make a programmer's job faster. Thus, some features that are regularly used on a website can be automated, such as database administration and user management.
Once a programmer handles a framework, it greatly improves their productivity and the code quality.
Django – a web framework
A framework is a set of software that organizes the architecture of an application and makes a developer's job easier. A framework can be adapted to different uses. It also gives practical tools to make a programmer's job faster. Thus, some features that are regularly used on a website can be automated, such as database administration and user management.
Once a programmer handles a framework, it greatly improves their productivity and the code quality.
Before the MVC framework existed, web programming mixed the database access code and the main code of the page. This returned an HTML page to the user. Even if we are storing CSS and JavaScript files in external files, server-side language codes are stored in one file that is shared between at least three languages: Python, SQL, and HTML.
The MVC pattern was created to separate logic from representation and have an internal architecture that is more tangible and real. The Model-View-Controller (MVC) represents the three application layers that the paradigm recommends:
- Models: These represent data organization in a database. In simple words, we can say that each model defines a table in the database and the relations between other models. It's thanks to them that every bit of data is stored in the database.
- Views: These contain all the information that will be sent to the client. They make views that the final HTML document will generate. We can associate the HTML code with the views.
- Controllers: These contain all the actions performed by the server and are not visible to the client. The controller checks whether the user is authenticated or it can generate the HTML code from a template.
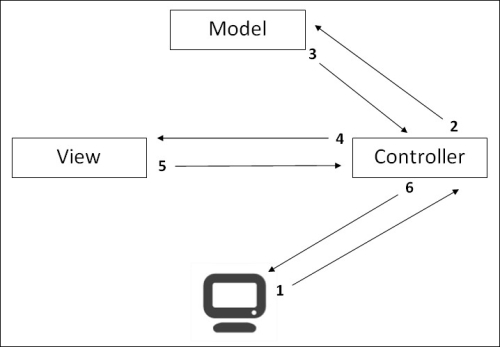
The following are the steps that are followed in an application with the MVC pattern:
- The client sends a request to the server asking to display a page.
- The controller uses a database through models. It can create, read, update, or delete any record or apply any logic to the retrieved data.
- The model sends data from the database; for example, it sends a product list if we have an online shop.
- The controller injects data into a view to generate it.
- The view returns its content depending on the data given by the controller.
- The controller returns the HTML content to the client.
The MVC pattern enables us to get coherence for each project's worker. In a web agency where there is a web designer and there are developers, the web designer is the head of the views. Given that views contain only the HTML code, the web designer will not be disturbed by the developer's code. Developers edit their models and controllers.
Django, in particular, uses an MVT pattern. In this pattern, views are replaced by templates and controllers are replaced by views. In the rest of this book, we will be using MVT patterns. Hence, our HTML code will be templates, and our Python code will be views and models.
The following is a nonexhaustive list of the advantages of using Django:
- Django is published under the BSD license, which assures that web applications can be used and modified freely without any problems; it's also free.
- Django is fully customizable. Developers can adapt to it easily by creating modules or overridden framework methods.
- This modularity adds other advantages. There are a lot of Django modules that you can integrate into Django. You can get some help with other people's work because you will often find high-quality modules that you might need.
- Using Python in this framework allows you to have benefits from all Python libraries and assures a very good readability.
- Django is a framework whose main goal is perfection. It was specifically made for people who want clear code and a good architecture for their applications. It totally respects the Don't Repeat Yourself (DRY) philosophy, which means keeping the code simple without having to copy/paste the same parts in multiple places.
- With regards to quality, Django integrates lots of efficient ways to perform unit tests.
- Django is supported by a good community. This is a very important asset because it allows you to resolve issues and fix bugs very fast. Thanks to the community, we can also find code examples that show the best practices.
Django has got some disadvantages too. When a developer starts to use a framework, he /she begins with a learning phase. The duration of this phase depends on the framework and the developer. The learning phase of Django is relatively short if the developer knows Python and object-oriented programming.
Also, it can happen that a new version of the framework is published that modifies some syntax. For example, the syntax of the URLs in the templates was changed with Version 1.5 of Django. (For more details, visit https://docs.djangoproject.com/en/1.5/ref/templates/builtins/#url.) Despite this, the documentation provides details of each Django update.
In this chapter, we studied the changes that have enabled the Web to evolve into Web 2.0. We also studied the operation of MVC that separates logic from representation. We finished the chapter with an introduction to the Django framework.
In the next chapter, we will set up our development environment with Python, PIP, and Django.