A Vue application is a combination of various components, bound together and orchestrated by the Vue framework. Knowing how to make your component is important. Each component is like a brick in the wall and needs to be made in a way that, when placed, doesn't end up needing other bricks to be reshaped in different ways around it. In this recipe, we are going to learn how to make a base component while following some important principles that focus on organization and clean code.
Getting ready
The prerequisite for this recipe is Node.js 12+.
The Node.js global objects that are required for this recipe are as follows:
- @vue/cli
- @vue/cli-service-global
To start our component, we can create our Vue project with the Vue CLI, as we learned in the Creating your first project with the Vue CLI recipe, or start a new one.
How to do it...
To start a new component, open a Terminal (macOS or Linux) or Command Prompt/PowerShell (Windows) and execute the following command:
> vue create my-component
The Command-Line Interface (CLI) will ask some questions that will help you create the project. You can use the arrow keys to navigate, the Enter key to continue, and the Spacebar to select an option. Choose the default option:
? Please pick a preset: (Use arrow keys)
❯ default (babel, eslint)
Manually select features
Let's create our first hello world component by following these steps:
- Let's create a new file called CurrentTime.vue file in the src/components folder.
- In this file, we will start with the <template> part of our component. It will be a shadowed-box card that will display the current date, formatted:
<template>
<div class='cardBox'>
<div class='container'>
<h2>Today is:</h2>
<h3>{{ getCurrentDate }}</h3>
</div>
</div>
</template>
- Now, we need to create the <script> part. We will start with the name property. This will be used when debugging our application with vue-devtools to identify our component and helps the Integrated Development Environment (IDE) too. For the getCurrentDate computed property, we will create a computed property that will return the current date, formatted by the Intl browser function:
<script>
export default {
name: 'CurrentTime',
computed: {
getCurrentDate() {
const browserLocale =
navigator.languages && navigator.languages.length
? navigator.languages[0]
: navigator.language;
const intlDateTime = new Intl.DateTimeFormat(
browserLocale,
{
year: 'numeric',
month: 'numeric',
day: 'numeric',
hour: 'numeric',
minute: 'numeric'
});
return intlDateTime.format(new Date());
}
}
};
</script>
- For styling our box, we need to create a style.css file in the src folder, then add the cardBox style to it:
.cardBox {
box-shadow: 0 5px 10px 0 rgba(0, 0, 0, 0.2);
transition: 0.3s linear;
max-width: 33%;
border-radius: 3px;
margin: 20px;
}
.cardBox:hover {
box-shadow: 0 10px 20px 0 rgba(0, 0, 0, 0.2);
}
.cardBox>.container {
padding: 4px 18px;
}
[class*='col-'] {
display: inline-block;
}
@media only screen and (max-width: 600px) {
[class*='col-'] {
width: 100%;
}
.cardBox {
margin: 20px 0;
}
}
@media only screen and (min-width: 600px) {
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
@media only screen and (min-width: 768px) {
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
@media only screen and (min-width: 992px) {
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
@media only screen and (min-width: 1200px) {
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
- In the App.vue file, we need to import our component so that we can see it:
<template>
<div id='app'>
<current-time />
</div>
</template>
<script>
import CurrentTime from './components/CurrentTime.vue';
export default {
name: 'app',
components: {
CurrentTime
}
}
</script>
- In the main.js file, we need to import the style.css file so that it's included in the Vue application:
import { createApp } from 'vue';
import './style.css';
import App from './App.vue';
createApp(App).mount('#app');
- To run the server and see your component, you need to open a Terminal (macOS or Linux) or Command Prompt/PowerShell (Windows) and execute the following command:
> npm run serve
Here is the component rendered and running:
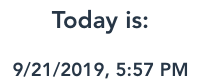
How it works...
The Vue component works almost like the Node.js packages. To use it in your code, you need to import the component and then declare it inside the components property on the component you want to use.
Like a wall of bricks, a Vue application is made of components that call and use other components.
For our component, we used the Intl.DateTimeFormat function, a native function that can be used to format and parse dates to declared locations. To get the local format, we used the navigator global variable.
See also
- You can find out more information about Intl.DateTimeFormat at https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/DateTimeFormat.
- You can find out more information about Vue components at https://v3.vuejs.org/guide/single-file-component.html.