Hello World Application
Now that we know a bit about what we’re looking at in a freshly initialized SvelteKit project, it seems appropriate to build a “Hello, World!” application. We’ll begin by opening src/routes/+page.svelte
in our editor. At this point, it should only contain basic HTML code:
<h1>Welcome to SvelteKit</h1> <p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
Since this file lives directly inside the src/routes/
directory and not a sub-directory, it is available in the browser as the URL's root route (i.e., /
). If we were to create a new folder within the routes directory (i.e., src/routes/hello/
) and place another +page.svelte
file inside of that (i.e., src/routes/hello/+page.svelte
), then we would make the /hello
route available as a valid URL for our app. We’ll cover more advanced routing techniques in later chapters, but for now, just know that to add a new route, you’ll need to create a folder using the desired route name in the routes
directory as well as a +
page.svelte
file.
Svelte Components
Readers familiar with Svelte will have noticed that the +page.svelte
file extension is that of a Svelte component. That’s because it is a Svelte component! As such, we can adjust the HTML in it, customize the look with CSS in <style>
tags, write JS inside of <script>
tags, and import other Svelte components. If you’re unfamiliar with Svelte components, it’s recommended to learn at least the basics before continuing further. Check out Svelte 3 Up and Running by Allessandro Segala or visit the official Svelte website (https://svelte.dev) for more information.
Let’s make some changes to src/routes/+page.svelte
to see what’s happening. Change the inner text of the <h1>
tag to read Hello, World!
, like so:
<h1>Hello, World!</h1>
Thanks to Vite, the page in our browser is updated immediately after saving. If your setup has dual monitors available with code shown on one and your browser on the other, you’ll quickly see how valuable Hot Module Replacement (HMR) can be. The change we’ve made is all well and good, but it isn’t really a “Hello, World!” application if users can’t change the text. For a true “Hello, World!” example, we need to show some text that has been provided by the user. The following code is an implementation showing just that:
<script> let name = 'World'; </script> <form> <label for="name" >What is your name?</label> <input type="text" name="name" id="name" bind:value={name} /> </form> <h1>Hello, {name}!</h1>
This simple Svelte component creates a variable named name
with the default value of “World
.” From there, the HTML gives us a basic form binding the text input value to our variable and outputting the text inside an HTML <h1>
tag. Thanks to Svelte’s reactivity and the binding of the text input value to the name
variable, the text provided is shown immediately, even as it is typed.
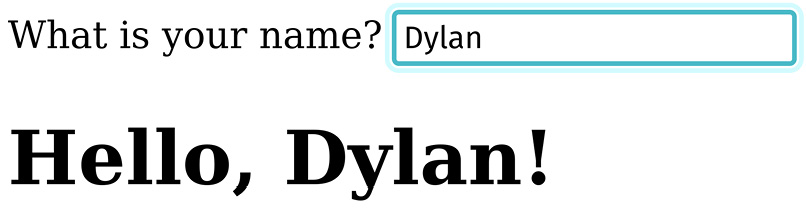
Figure 1.2 – The output from our “Hello, World!” component