When talking about cascade in CSS, there will no doubt be a mention of the browser default settings getting a higher precedence than the author's preferred styling. When writing Less code, you will overwrite the browser's default styling. In other words, anything that you do not define will be assigned a default styling, which is defined by the browser. This behavior plays a major role in many cross-browser issues. To prevent these sorts of problems, you can perform a CSS reset. The most famous browser reset is Eric Meyer's CSS Reset (accessible at http://meyerweb.com/eric/tools/css/reset/).
CSS resets overwrite the default styling rules of the browser and create a starting point for styling. This starting point looks and acts the same on all (or most) browsers. In this book, normalize.css v2 is used. Normalize.css is a modern, HTML5-ready alternative to CSS resets and can be downloaded from http://necolas.github.io/normalize.css/. It lets browsers render all elements more consistently and makes them adhere to modern standards.
To use a CSS reset, you can make use of the @import
directive of Less. With @import
, you can include other Less files in your main Less file. The syntax is @import "{filename}";
. By default, the search path for the directives starts at the directory of the main file. Although setting alternative search paths is possible (by setting the path's variable of your Less environment), it will not be used in this book.
The example Less files in this book will contain @import "normalize.less";
in the first few lines of the code. Again, you should note that normalize.less
does contain the CSS code. You should pay particular attention to the profits of this solution!
If you want to change or update the CSS reset, you will only have to replace one file. If you have to manage or build more than one project, which most of you should be doing, then you can simply reuse the complete reset code.
A new feature in CSS3 is the possibility of adding a gradient in the background color of an element. This acts as a replacement for complex code and image fallbacks.
It is possible to define different types of gradient and use two or more colors. In the following figure, you will see a background gradient of different colors:

A gradient example (from W3schools.com)
In the next example, you can use a linear gradient of two colors. The background gradients use vendor-specific rules.
You can make use of the example code from the rounded corners example to add gradients to it.
The first step is to copy or open less/gradient.less
and add a new mixin at the start of this file as shown in the following code:
/* Mixin */ .gradient (@start: black, @stop: white,@origin: left) { background-color: @start; background-image: -webkit-linear-gradient(@origin, @start, @stop); background-image: -moz-linear-gradient(@origin, @start, @stop); background-image: -o-linear-gradient(@origin, @start, @stop); background-image: -ms-linear-gradient(@origin, @start, @stop); background-image: linear-gradient(@origin, @start, @stop); }
This will create gradients from the left (@origin
) to the right with colors from @start
to @stop
. This mixin has default values.
IE9 (and its earlier versions) do not support gradients. A fallback can be added by adding background-color: @start;
, which will create a uniform colored background for older browsers.
After adding the mixin to your code, you can call on it for our #header
, #body
, and #footer
selectors as shown in the following code:
#header{ background-color: red; .roundedcornersmixin(); .gradient(red,lightred); } #content{ background-color: white; min-height: 300px; .roundedcornersmixin(20px); .gradient(); } #footer{ background-color: navy; .roundedcornersmixin(20px); .gradient(navy,lightblue); }
For example, if you renamed the Less file to less/gradient.less
, you would have also had to change the reference in your HTML file to the following code:
<link rel="stylesheet/less" type="text/css" href="less/gradient.less" />
If you now load the HTML file in the browser, your results should be like the following screenshot:
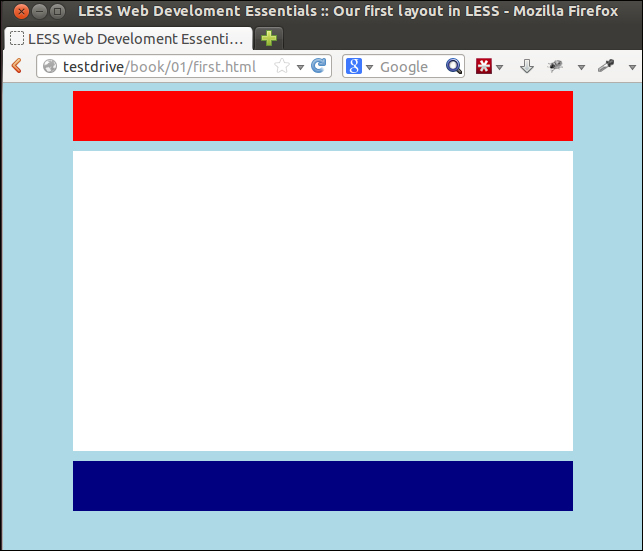
Gradients in the header, content, and footer from the example code