As mentioned, Xamarin created a new platform in which the development efforts target multiple operating systems and a variety of devices. Most importantly, compiled applications do not run an interpreted sequence but have a native code base (such as Xamarin.iOS) or an integrated .NET application runtime (such as Xamarin.Android). In essence, Xamarin replaces the Common Language Runtime and IL for .NET applications with compiled binaries and an execution context, the so-called mono runtime.
With Android applications, mono runtime is placed right on top of the Linux kernel. This creates a parallel execution context to the Android runtime. Xamarin code is then compiled into IL and accessed by mono runtime. On the other hand, Android runtime is accessed by the so-called Managed Callable Wrappers (MCW) which is a marshalling wrapper between the two runtimes. The MCW layer is responsible for converting managed types to Android runtime types and invoking Android code at execution time. Every time that .NET code needs to invoke Java code, this JNI (Java Interop) bridge is used. MCW provides a wide range of applications including inheriting Java types, overriding methods and implementing Java interfaces.
The following image shows the Xamarin.Android architecture:
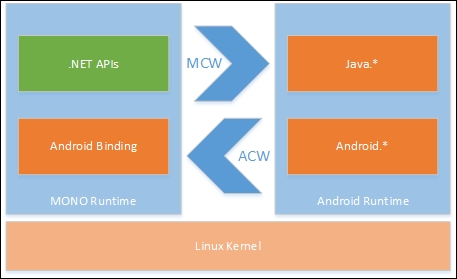
Figure 1: Xamarin.Android Architecture
Android.*
and Java.*
namespaces are used throughout the MCWs to access device- and platform-specific features in Android runtime and Java APIs such as facilities like audio, graphics, OpenGL, and telephony .
Using the interop libraries, it is also possible to load native libraries and execute native code in the execution context with Xamarin.Android. The reverse callback execution in this case is handled through
Android Callable Wrappers (ACW). ACW is a JNI bridge which allows the Android runtime to access the .NET domain. An ACW is generated at compile-time for each managed class that is directly or indirectly related to Java types (those that inherit Java.Lang.Object
).
In iOS applications, the use of an integrated parallel runtime is (unfortunately) not permissible under the iOS SDK agreement. According to the iOS SDK agreement, interpreted code can only be used if all of the the scripts and code are downloaded and run by Apple's WebKit framework.
With this restriction in place, developers can still develop applications in .NET and share code over the other three platforms. At compile time, projects are first compiled into IL code and then (with the Mono Touch Ahead-Of-Time compiler—mtouch) into static native iOS bits. This means that iOS applications developed with Xamarin are completely native applications.
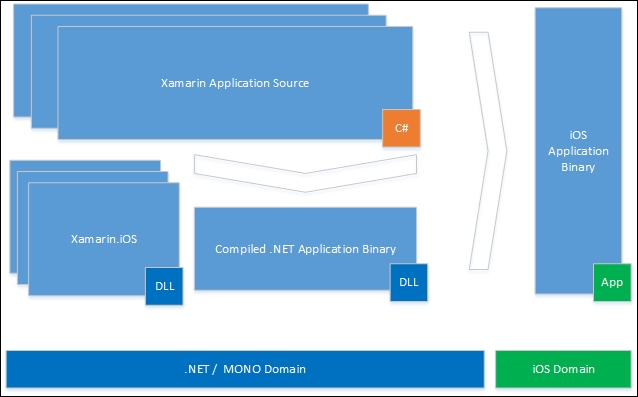
Figure 2: Xamarin.iOS Compilation
Xamarin.iOS, like Xamarin.Android, contains an interop engine that bridges the .NET world with the Objective-C world. Through this bridge, under the ObjCRuntime
namespace, users are able to access iOS C-based APIs, as well as using the Foundation namespace, and can use and derive from native types and access Objective-C properties. For instance, Objective-C types like NSObject
, NSString
, and NSArray
are exposed in C# and provide binding to underlying types. These types can be used either as memory references or as strongly-typed objects. This improves the development experience and also increases type-safety.
This static compilation is the main reason for using a build machine to develop iOS applications with Xamarin on the Windows platform. Therefore, there is no reverse-callback functionality in Xamarin.iOS where calls to native runtime from .NET code are supported but calls from native code back to .NET domain are not. There are other features that are disabled because of the way that Xamarin.iOS applications are compiled. For example, no generic types are allowed to inherit from NSObject
. Another important limitation is the fact that no dynamic type creation is allowed at runtime which, in return, disables the use of dynamic keywords in Xamarin.iOS applications.
Note
Xamarin.iOS application packages, if built in a debug configuration, are much larger than their Release counterparts when compared to other platforms. These packages are instrumented and not optimized by the linker. Profiling of these packages is not allowed in Xamarin.iOS applications.
In a similar way to Xamarin.Android development, with Xamarin.iOS, it is also possible to re-use native code and libraries from managed code. To do this, Xamarin provides a project template called a binding library. A binding library helps developers create managed wrappers for native Objective-C code.
Even though Xamarin does not include Windows Runtime as a target platform nor provide specialized tools for it (other than Xamarin.Forms), cross-platform projects that involve Xamarin can and generally do include Windows Runtime projects. Since .NET and C# are indigenous to Windows Runtime, most of the shared projects (such as portable libraries, shared projects, and Xamarin.Forms projects) can be reused in Windows Runtime with no further modification.
With Windows Runtime, developers can create both Windows Phone 8.1 and Windows Store applications. Windows Phone 8 and Windows Phone 8.1 Silverlight can also be targeted and included in the PCL description.