We are going to start off with the very building blocks of all Titanium applications, Windows and Views. By the end of this recipe you will understand how to implement a Window and add Views to it, as well as understand the fundamental difference between the two, which is not as obvious as it may seem at first glance.
If you are intending to follow the entire chapter and build the LoanCalc app, then pay careful attention to the first few steps of this chapter, as you will need to perform these steps again for each subsequent app in the book.
Note
We are assuming that you have already downloaded and installed Titanium Studio and either Apple XCode with the iOS SDK or Google's Android SDK, or both. If not, you can follow along with the installation process via the online tutorial at http://boydlee.com/titanium-appcelerator-cookbook/setup.
To follow this recipe you will need Titanium Studio installed. We are using version 1.0.7, which is the latest version at the time of writing. Additionally, you will also need either the iOS SDK with XCode or the Google Android SDK installed. All of our examples generally work on either platform unless specified explicitly at the start of a chapter. You will also need an IDE to write your code. Any IDE including Notepad, TextMate, Dashcode, Eclipse, and so on, can be used. However, since June 2011, Appcelerator has been providing its own IDE called "Titanium Studio", which is based on Aptana. Titanium Studio allows developers to build, test, and deploy iOS, Android, Blackberry, and mobile web apps from within a single development environment. All of the recipes within this book are based on the assumption that you are using the Titanium Studio product, which can be downloaded for free from https://my.appcelerator.com/auth/signup/offer/community.
To prepare for this recipe, open Titanium Studio and log in if you have not already done so. If you need to register a new account, you can do so for free directly from within the application. Once you are logged in, click on File | New | New Titanium Mobile Project, and the details window for creating a new project will appear. Enter in LoanCalc the name of the app, and fill in the rest of the details with your own information as shown in the following screenshot. You can also uncheck the "iPad" option, as we will only be building our application for the iPhone and Android platforms.
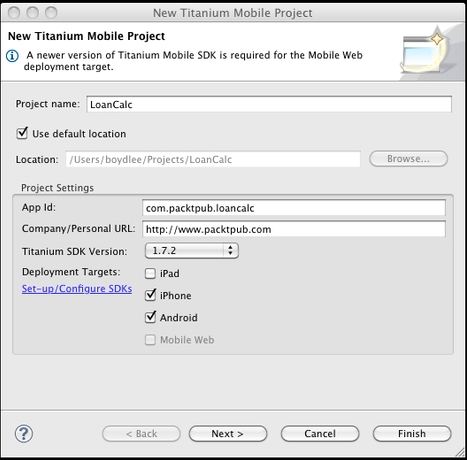
Note
Pay attention to the app identifier, which is written normally in reverse domain notation (that is, com.packtpub.loancalc
). This identifier cannot be easily changed after the project is created and you will need to match it exactly when creating provisioning profiles for distributing your apps later on.
Complete source code for this recipe can be found in the /Chapter1/Recipe1
folder.
First, open the app.js
file in Titanium Studio. If this is a new project, by default Titanium Studio creates a sample app containing a couple of Windows inside of a TabGroup
which is certainly useful but we will cover TabGroups in a later recipe, so go ahead and remove all of the generated code. Now let's create a Window object to which we will add a View object. This View object will hold all of our controls, such as TextFields and Labels.
In addition to creating our base Window and View, we will also create an ImageView
component to display our app logo before adding it to our View (you can get the image we used from the source code for chapter).
Finally, we'll call the open()
method on the Window
to launch it:
//create the window var win1 = Titanium.UI.createWindow({ width: 320, height: 480, top: 0, left: 0, backgroundImage: 'background.png' }); //create the view, this will hold all of our UI controls //note the height of this view is the height of the window //minus 40px for the status bar and padding var view = Titanium.UI.createView({ width: 300, height: win1.height - 40, left: 10, top: 10, backgroundColor: '#fff', borderRadius: 5 }); //we will give the logo a left margin so it centers neatly //within our view var _logoMarginLeft = (view.width - 253) / 2; //now let's add our logo to an imageview and add that to our //view object var logo = Titanium.UI.createImageView({ image: 'logo.png', width: 253, height: 96, left: _logoMarginLeft, top: 0 }); view.add(logo); //add the view to our window win1.add(view); //finally, open the window to launch the app win1.open();
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.PacktPub.com. If you purchased this book elsewhere, you can visit http://www.PacktPub.com/support and register to have the files e-mailed directly to you.
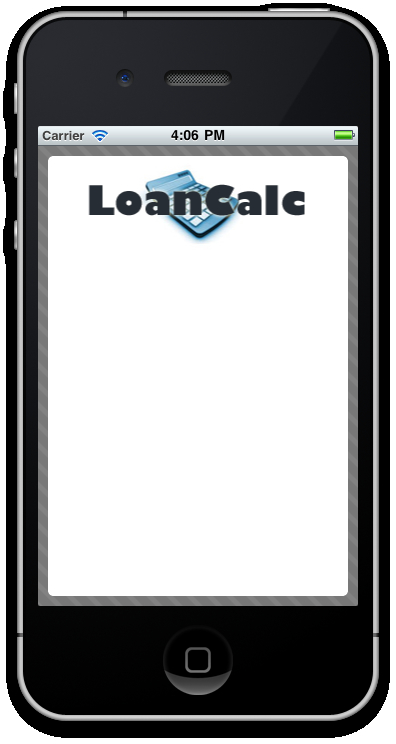
Firstly, it's important to explain the differences between Windows
and Views
as there are a few fundamental differences that may influence your decision on using one compared to the other. Unlike Views, Windows have some additional abilities including an open()
and close()
method. If you come from a desktop development background, you can imagine a Window as the equivalent of a form or screen. If you prefer web analogies, then a Window is more like a page whereas Views are more like a Div. In addition to these methods, Windows also have display properties such as fullscreen
and modal
which are not available in Views. You will also notice that when creating a new object the create
keyword pops up, that is Titanium.UI.createView()
. This naming convention is used consistently throughout the Titanium API, and almost all components are instantiated this way.
Windows and Views can be thought of as the building blocks of your Titanium application. All of your UI components are added to either a Window, or a View, which is a child of a Window. There are a number of formatting options available for both of these objects, the properties and syntax of which will be very familiar to anyone who has used CSS in the past. Font, Color, BorderWidth, BorderRadius, Width, Height, Top, and Left are all properties that function exactly the same way as you would expect them to in CSS and apply to Windows and almost all Views.
Tip
It's important to note that your app requires at least one Window to function and that Window must be called from within your entry point which is the app.js file.
You may have also noticed that we sometimes instantiated objects or called methods using Titanium.UI.createXXX
, and at other times used Ti.UI.createXXX
. Using "Ti" is simply a short-hand namespace designed to save your time during coding, and will execute your code in exactly the same manner as the full "Titanium" namespace does.