Sliders and Switches are two UI components that are simple to implement and can bring an extra level of interactivity to your apps. Switches, as the name suggests, have only two states—on and off—which are represented by Boolean values (true and false).
Sliders, on the other hand, take two float values, a minimum and maximum, and allow the user to select any number between and including these two values. In addition to it's default styling, the Slider API also allows you to use images for both sides of the 'track' and the 'slider thumb' image that runs along it. This allows you to create some truly customised designs.
We are going to add a Switch to indicate an on/off state and a Slider to hold the loan length, with values ranging from a minimum of 6 to a maximum of 72 months. Also, we'll add some event handlers to capture the changed value from each component, and in the case of the Slider, update an existing Label with the new Slider value. Don't worry if you aren't 100 percent sure about how event handlers work yet, as we will explain this in further detail in Chapter 6, Getting To Grips With Events & Properties.
The complete source code for this recipe can be found in the /Chapter 1/Recipe 6
folder.
If you are following with the LoanCalc app, the code below should be placed into your window2.js
file for the Switch. We'll also add in a label to identify what the Switch component does and a View component to hold it all together:
//reference the current window var win1 = Titanium.UI.currentWindow; //create the view, this will hold all of our UI controls var view = Titanium.UI.createView({ width: 300, height: 70, left: 10, top: 10, backgroundColor: '#fff', borderRadius: 5 }); //create a label to identify the switch control to the user var labelSwitch = Titanium.UI.createLabel({ width: 'auto', height: 30, top: 20, left: 20, font: {fontSize: 14, fontFamily: 'Helvetica', fontWeight: 'bold'}, text: 'Auto Show Chart?' }); view.add(labelSwitch); //create the switch object var switchChartOption = Titanium.UI.createSwitch({ right: 20, top: 20, value: false }); view.add(switchChartOption); win1.add(view);
Now, let's write Slider code. Go back to your app.js
file and type in the following code underneath the line view.add(tfInterestRate);
:
//create the slider to change the loan length var lengthSlider = Titanium.UI.createSlider({ width: 140, top: 200, right: 20, min: 12, max: 60, value: numberMonths, thumbImage: 'sliderThumb.png', selectedThumbImage: 'sliderThumbSelected.png', highlightedThumbImage: 'sliderThumbSelected.png' }); lengthSlider.addEventListener('change', function(e){ //output the value to the console for debug Ti.API.info(lengthSlider.value); //update our numberMonths variable numberMonths = Math.round(lengthSlider.value); //update label labelLoanLength.text = 'Loan length (' + Math.round(numberMonths) + ' months):'; }); view.add(lengthSlider);
In this recipe we are adding two new components to two separate Views within two separate Windows. The first component, a Switch, is fairly straight forward, and apart from the standard layout and positioning properties, takes one main Boolean value to determine its on or off status. It also has only the one event, change
, which is executed whenever the Switch changes from the on to off position or vice versa.
On the Android platform, the Switch can be altered to appear as a toggle button (default) or as a checkbox. Additionally, Android users can also display a text label using the title
property, which can be changed programmatically using the titleOff
and titleOn
properties.
The Slider component is more interesting and has many more properties than a Switch. Sliders are useful in instances where you want to allow the user to choose between a range of values, in our case, a numeric range of months from 12 to 60. For instance, this is a much more effective method of choosing a number from a range than it would be to list all of the possible options in a Picker, and a much safer way than letting a user enter in possibly invalid values via a TextField or TextArea component.
Pretty much all of the Slider can be styled using the default properties available in the Titanium API, including thumbImage
, selectedThumbImage
, and highlightedThumbImage
as we have done in this recipe. The highlightedThumbImage
works similar to how you might be used to in CSS. The image for the thumbnail in this case changes only when a user taps and holds on to the component in order to change its value.
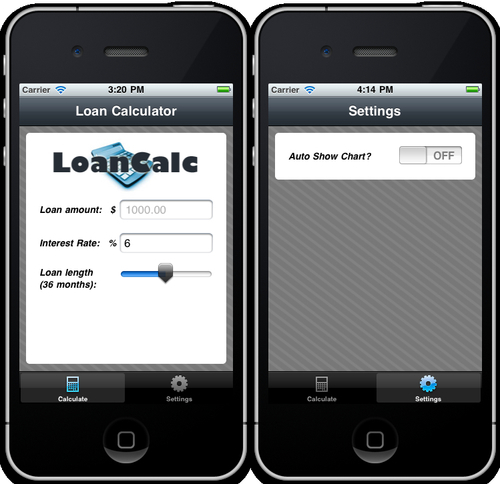