When a TextField or TextArea control gains focus in either the iPhone or Android, the default keyboard is what springs up onto the screen. However, there will be times when you wish to change this behavior example, you may only want to have the user input numeric characters into a TextField when they are providing a numerical amount (such as their age, or a monetary value). Additionally, Keyboard Toolbars can be created to appear above the keyboard itself, which will allow you to provide the user with other options such as removing the keyboard from the Window, or allowing copy/paste operations via a simple button tap.
In the following recipe, we are going to create a toolbar that contains both a system button, and another system component called FlexibleSpace. These will be added to the top of our numeric keyboard which will appear whenever the TextField for amount or interest rate gains focus. Note that in this example we have updated the tfAmount
and tfInterestRate
TextField objects to now contain keyboardType
and returnKeyType
properties.
Note that toolbars are iPhone-specific, and that they may not be available for Android in the current Titanium SDK.
Open up your app.js
file and type in the following code. If you have been following along from the previous recipe, this code should replace the previous recipe's code for adding the amount and interest rate TextFields:
//flexible space for button bars var flexSpace = Titanium.UI.createButton({ systemButton:Titanium.UI.iPhone.SystemButton.FLEXIBLE_SPACE }); //done system button var buttonDone = Titanium.UI.createButton({ systemButton:Titanium.UI.iPhone.SystemButton.DONE, bottom: 0 }); //add the event listener 'click' event to our done button buttonDone.addEventListener('click', function(e){ tfAmount.blur(); tfInterestRate.blur(); tfInterestRate.top = 150; labelInterestRate.top = 150; interestRate = tfInterestRate.value; tfAmount.visible = true; labelAmount.visible = true; }); //creating the textfield for our loan amount input var tfAmount = Titanium.UI.createTextField({ width: 140, height: 30, top: 100, right: 20, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED, returnKeyType:Titanium.UI.RETURNKEY_DONE, hintText: '1000.00', keyboardToolbar: [flexSpace,buttonDone], keyboardType:Titanium.UI.KEYBOARD_PHONE_PAD }); view.add(tfAmount); //creating the textfield for our percentage interest rate //input var tfInterestRate = Titanium.UI.createTextField({ width: 140, height: 30, top: 150, right: 20, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED, returnKeyType:Titanium.UI.RETURNKEY_DONE, value: interestRate, keyboardToolbar: [flexSpace,buttonDone], keyboardType:Titanium.UI.KEYBOARD_PHONE_PAD }); //if the interest rate is focused change its top value so we //can see it (only for the iphone platform though!) tfInterestRate.addEventListener('focus', function(e){ if(Ti.Platform.osname == 'iphone') { tfInterestRate.top = 100; labelInterestRate.top = 100; tfAmount.visible = false; labelAmount.visible = false; } }); view.add(tfInterestRate);
In this recipe we are creating a TextField and adding it to our View. By now, you should have noticed how many properties are universal among the different UI components; width
, height
, top,
and right
are just four that are used in our TextField called tfAmount
that have been used in previous recipes for other components. Many touch screen phones do not have physical keyboards; instead we are using a touch screen keyboard to gather our input data. Depending on the data you require, you may not need a full keyboard with all of the QWERTY keys and may want to just display a numeric keyboard (as seen in the following screenshot); such as when you were using the telephone dialling features on your iPhone or Android device. Additionally, you may require the QWERTY keys but in a specific format; custom keyboards make user input quicker and less frustrating for the user by presenting custom options such as keyboards for inputting web addresses and emails with all of the 'www' and '@' symbols in convenient touch locations.
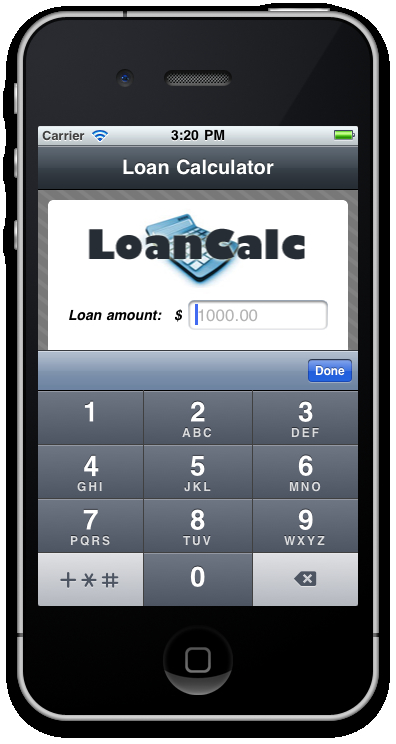
Try experimenting with other Keyboard styles in your Titanium app!