Events are essentially functions used in a distinctive way. Both the Start
and Update
functions, which we have already seen, would more accurately be described as Unity-specific events. Events are functions called to notify an object that something significant has happened: the level has begun, a new frame has started, an enemy has died, the player has jumped, and others. In being called at these critical times, they offer objects the chance to respond if necessary. The
Start
function is called automatically by Unity when the object is first created, typically at level startup. The Update
function is also called automatically, once on each frame. The Start
function, therefore, gives us an opportunity to perform specific actions when the level begins, and the Update
function on each frame many times per second. The Update
function is especially useful, therefore, to achieve motion and animation in your games. Refer to code sample 1-9, which rotates an object over time:
01 using UnityEngine; 02 using System.Collections; 03 04 public class MyScriptFile : MonoBehaviour 05 { 06 // Use this for initialization 07 void Start () 08 { 09 } 10 11 // Update is called once per frame 12 void Update () 13 { 14 //Rotate object by 2 degrees per frame around the Y axis 15 transform.Rotate(new Vector3(0.0f, 2.0f, 0.0f)); 16 } 17 }
Line 15 in code sample 1-9 is called once per frame. It continually rotates an object 2 degrees around the y axis. This code is frame rate dependent, which means that it'll turn objects faster when run on machines with higher frame rates, because Update
will be called more often. There are techniques to achieve frame rate independence, ensuring that your games perform consistently across all machines, regardless of the frame rate. We'll see these in the next chapter. You can easily check the frame rate for your game directly from the Unity Editor Game tab. Select the Game tab and click on the Stats button in the top-right hand corner of the toolbar. This will show the Stats panel, offering a general, statistical overview of the performance of your game. This panels displays the game frames per second (FPS), which indicates both how often Update
is called on your objects and the general performance of your game on your system. In general, an FPS lower than 15 indicates a significant performance problem. Strive for FPS rates of 30 or above. Refer to the following screenshot to access the Stats panel:
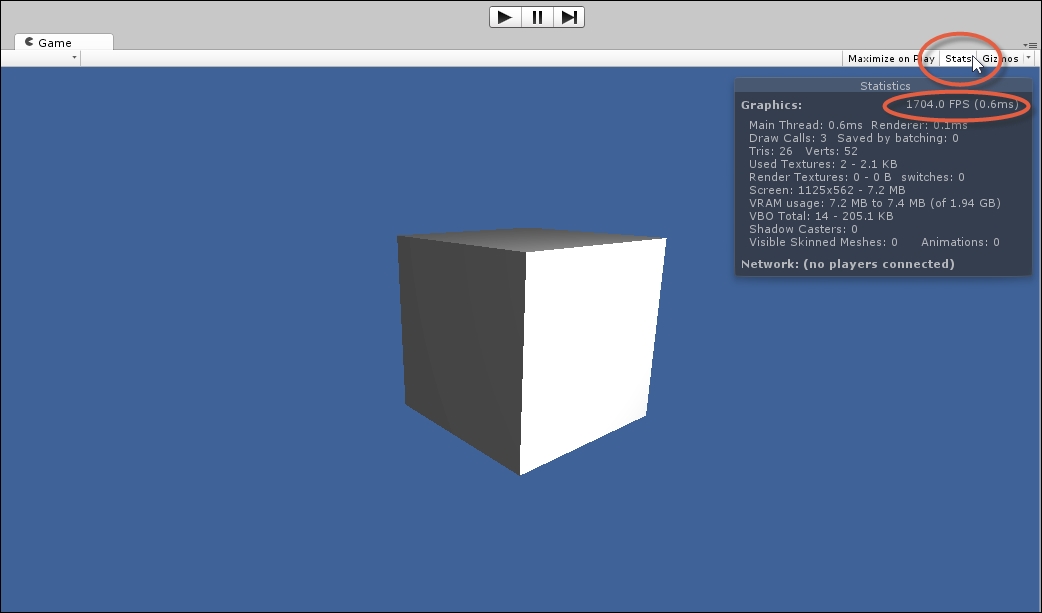
Accessing the Stats panel for the Game tab to view FPS
Tip
There are too many event types to list comprehensively. However, some common events in Unity, such as Start
and Update
, can be found in the MonoBehaviour
class. More information on MonoBehaviour
is available at http://docs.unity3d.com/ScriptReference/MonoBehaviour.html.