Lists and sequences are everywhere in games. For this reason, you'll frequently need to keep track of lists of data of the same type: all enemies in the level, all weapons that have been collected, all power ups that could be collected, all spells and items in the inventory, and so on. One type of list is the array. Each item in the array is, essentially, a unit of information that has the potential to change during gameplay, and so a variable is suitable to store each item. However, it's useful to collect together all the related variables (all enemies, all weapons, and so on) into a single, linear, and traversable list structure. This is what an array achieves. In C#, there are two kinds of arrays: static and dynamic. Static arrays might hold a fixed and maximum number of possible entries in memory, decided in advance, and this capacity remains unchanged throughout program execution, even if you only need to store fewer items than the capacity. This means some slots or entries could be wasted. Dynamic arrays might grow and shrink in capacity, on demand, to accommodate exactly the number of items required. Static arrays typically perform better and faster, but dynamic arrays feel cleaner and avoid memory wastage. This chapter considers only static arrays, and dynamic arrays are considered later, as shown in the following code sample 1-4:
01 using UnityEngine; 02 using System.Collections; 03 04 public class MyScriptFile : MonoBehaviour 05 { 06 //Array of game objects in the scene 07 public GameObject[] MyObjects; 08 09 // Use this for initialization 10 void Start () 11 { 12 } 13 14 // Update is called once per frame 15 void Update () 16 { 17 } 18 }
In code sample 1-4, line 07 declares a completely empty array of GameObjects
, named MyObjects
. To create this, it uses the []
syntax after the data type GameObject
to designate an array, that is, to signify that a list of GameObjects
is being declared as opposed to a single GameObject
. Here, the declared array will be a list of all objects in the scene. It begins empty, but you can use the Object Inspector in the Unity Editor to build the array manually by setting its maximum capacity and populating it with any objects you need. To do this, select the object to which the script is attached in the scene and type in a Size value for the My Objects field to specify the capacity of the array. This should be the total number of objects you want to hold. Then, simply drag-and-drop objects individually from the scene hierarchy panel into the array slots in the Object Inspector to populate the list with items, as shown here:
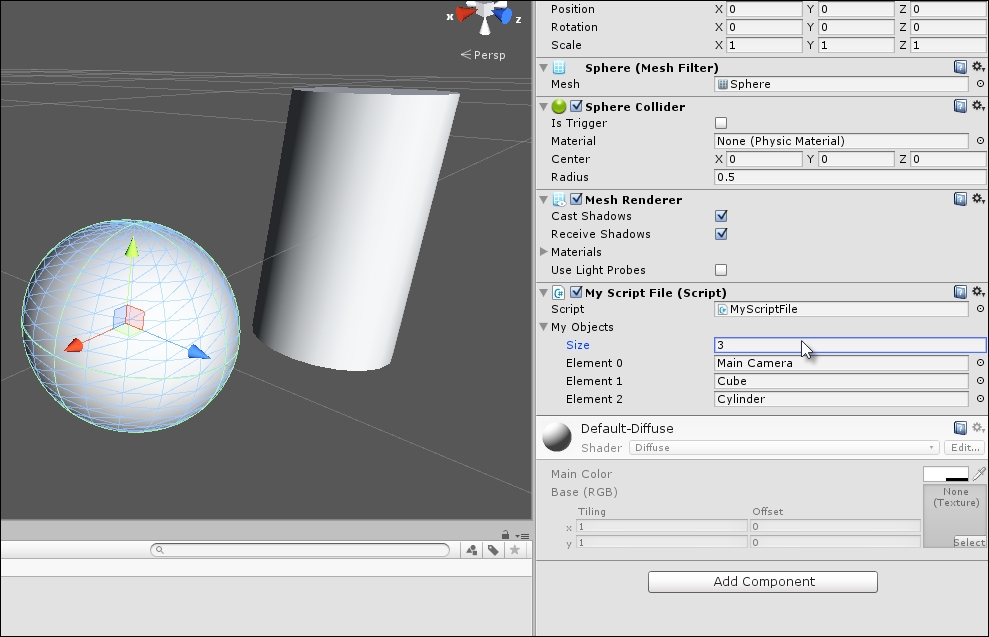
Building arrays from the Unity Object Inspector
You can also build the array manually in code via the Start
function instead of using the Object Inspector. This ensures that the array is constructed as the level begins. Either method works fine, as shown in the following code sample 1-5:
01 using UnityEngine; 02 using System.Collections; 03 04 public class MyScriptFile : MonoBehaviour 05 { 06 //Array of game objects in the scene 07 public GameObject[] MyObjects; 08 09 // Use this for initialization 10 void Start () 11 { 12 //Build the array manually in code 13 MyObjects = new GameObject[3]; 14 //Scene must have a camera tagged as MainCamera 15 MyObjects[0] = Camera.main.gameObject; 16 //Use GameObject.Find function to 17 //find objects in scene by name 18 MyObjects[1] = GameObject.Find("Cube"); 19 MyObjects[2] = GameObject.Find("Cylinder"); 20 } 21 22 // Update is called once per frame 23 void Update () 24 { 25 } 26 }
The following are the comments for code sample 1-5:
Line 10: The
Start
function is executed at level startup. Functions are considered in more depth later in this chapter.Line 13: The
new
keyword is used to create a new array with a capacity of three. This means that the list can hold no more than three elements at any one time. By default, all elements are set to the starting value ofnull
(meaning nothing). They are empty.Line 15: Here, the first element in the array is set to the main camera object in the scene. Two important points should be noted here. First, elements in the array can be accessed using the array subscript operator
[]
. Thus, the first element ofMyObjects
can be accessed withMyObjects[0]
. Second, C# arrays are "zero indexed". This means the first element is always at position0
, the next is at1
, the next at2
, and so on. For theMyObjects
three-element array, each element can be accessed withMyObjects[0]
,MyObjects[1]
, andMyObjects[2]
. Notice that the last element is2
and not3
.Lines 18 and 19: Elements
1
and2
of theMyObjects
array are populated with objects using the functionGameObject.Find
. This searches the active scene for game objects with a specified name (case sensitive), inserting a reference to them at the specified element in theMyObjects
array. If no object of a matching name is found, thennull
is inserted instead.
Tip
More information on arrays and their usage in C# can be found online at http://msdn.microsoft.com/en-GB/library/9b9dty7d.aspx.