Introducing React Native
React Native is a framework that makes it possible to write React code and deploy it to multiple platforms. The most well known are iOS and Android, but you can use React Native to create apps for Windows, macOS, Oculus, Linux, tvOS, and much more. With React Native for Web, you can even deploy a mobile application as a web app using the same code.
Tip
If you don’t want to spend an hour setting up the development environment for creating a new React Native app and trying out the code examples, you could install the Expo CLI using npm
or yarn
:
npm install -g expo-cli OR yarn global add expo-cli
After that, setting up a new React Native app just takes running one command in the terminal:
expo init NameOfYourApp
Pro tip: The default package manager for a new app created by running expo init
is yarn. If you want to use npm
instead, add --npm
to the expo init
command.
In the next section, you will learn how cross-platform development is made possible in the React Native framework.
React Native basics
As React Native is heavily based on React, the code looks much the same; you use components to structure the code, props to hand over parameters from one component to another, and JSX in a return statement to render the view. One of the main differences is the type of basic JSX components you can use.
In React, they look a lot like XML/HTML tags, as we have seen in the previous section. In React Native, the so-called core components are imported from the react-native
library and look different:
import React from 'react'; import {ScrollView, Text, View} from 'react-native'; const App = () => { return ( <ScrollView contentInsetAdjustmentBehavior="automatic"> <View> <Text>Hello World!</Text> </View> </ScrollView> ); }; export default App;
React Native does not use web views to render the JavaScript code on the device like some other cross-platform solutions; instead, it converts the UI written in JavaScript into native UI elements. The React Native View
component, for example, gets converted into a ViewGroup
component for Android, and into a UIView
component for iOS. This conversion is done via the Yoga engine (https://yogalayout.com).
React Native is powered by two threads – the JavaScript thread, where the JavaScript code is executed, and the native thread (or UI thread), where all device interaction such as user input and drawing screens happens.
The communication between these two threads takes place over the so-called Bridge, which is a kind of interface between the JavaScript code and the native part of the app. Information such as native events or instructions is sent in serialized batches from the native UI thread over the Bridge to the JavaScript thread and back. This process is shown in the following diagram:
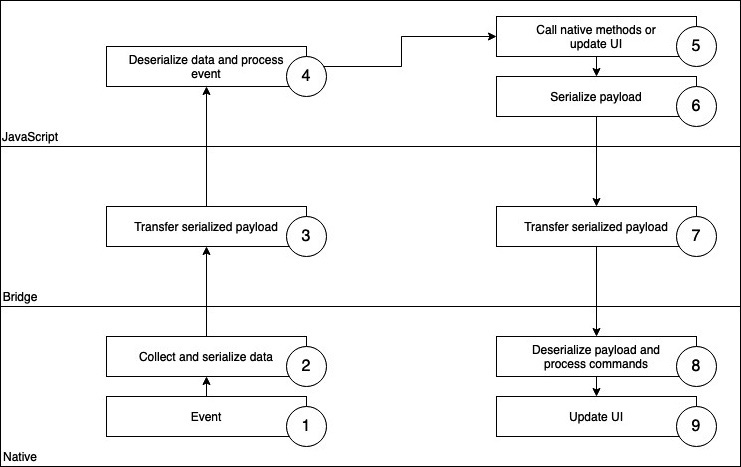
Figure 1.1 – React Native Bridge
As you can see, events are collected in the native thread. The information is then serialized and passed to the JavaScript thread via the Bridge. In the JavaScript thread, information is deserialized and processed. This also works the other way round, as you can see in Steps 5 to 8 of the preceding diagram. You can call methods, which are provided by native components, or React Native can update the UI when necessary. This is also done by serializing the information and passing it to the native thread via the Bridge. This Bridge makes it possible to communicate between native and JavaScript in an asynchronous way, which is great to create real native apps with JavaScript.
But it also has some downsides. The serialization and deserialization of information, as well as being the only central point of communication between native and JS, makes the bridge a bottleneck that can cause performance issues in some situations. This is why React Native was completely rewritten between 2018 and 2022.
The new React Native (2022)
Because of the architectural problems mentioned previously, the React Native core was rearchitectured and rewritten completely. The main goal was to get rid of the Bridge and the performance issues tied to it. This was done by introducing JSI, the JavaScript interface, which allows direct communication between native and JavaScript code without the need for serialization/deserialization.
The JS part is truly aware of the native objects, which means you can directly call methods synchronously. Also, a new renderer was introduced during the rearchitecture, which is called Fabric. More details on the React Native rearchitecture will be provided in Chapter 3, Hello React Native.
The rearchitecture made the awesome React Native framework even more awesome by improving its out-of-the-box performance significantly. At the time of writing, more and more packages are being adapted to the new React Native architecture.
More React Native advantages
Ever since it was open-sourced in 2015, there has been a huge and ever-growing community that develops and provides a lot of add-on packages for a multitude of different problems and use cases. This is one of the main advantages that React Native has over other, similar cross-platform approaches.
These packages are mostly well maintained and provide nearly all native functionality that currently exists, so you only have to work with JavaScript to write your apps.
This means using React Native for mobile app development makes it possible to reduce the size of the developer team greatly, as you no longer need both Android and iOS specialists, or you can at least reduce the team size of native specialists significantly.
And the best thing about working with these well-maintained packages is that things such as the React Native core rewrites come to your app automatically when the packages are updated.
Additionally, the hot reload feature speeds up the development process by making it possible to see the effect of code changes in a matter of seconds. Several other tools make the life of a React Native developer even more comfortable, which we will look at in more detail in Chapter 9, Essential Tools for Improving React Native Development.
Now that we understand what React and React Native are, and how they are related to each other, let’s have a look at a tool that makes the whole development process much easier – Expo.