Before we dive deep into MQTT, we must understand the publish-subscribe pattern, also known as the pub-sub pattern. In the publish-subscribe pattern, a client that publishes a message is decoupled from the other client or clients that receive the message. The clients don't know about the existence of the other clients. A client can publish messages of a specific type and only the clients that are interested in that specific type of message will receive the published messages.
The publish-subscribe pattern requires a server, also known as a broker. All the clients establish a connection with the server. A client that sends a message through the server is known as the publisher. The server filters the incoming messages and distributes them to the clients that are interested in that type of received messages. Clients that register to the server as interested in specific types of messages are known as subscribers. Hence, both publishers and subscribers establish a connection with the server.
It is easy to understand how things work with a simple diagram. The following diagram shows one publisher and two subscribers connected to a server:
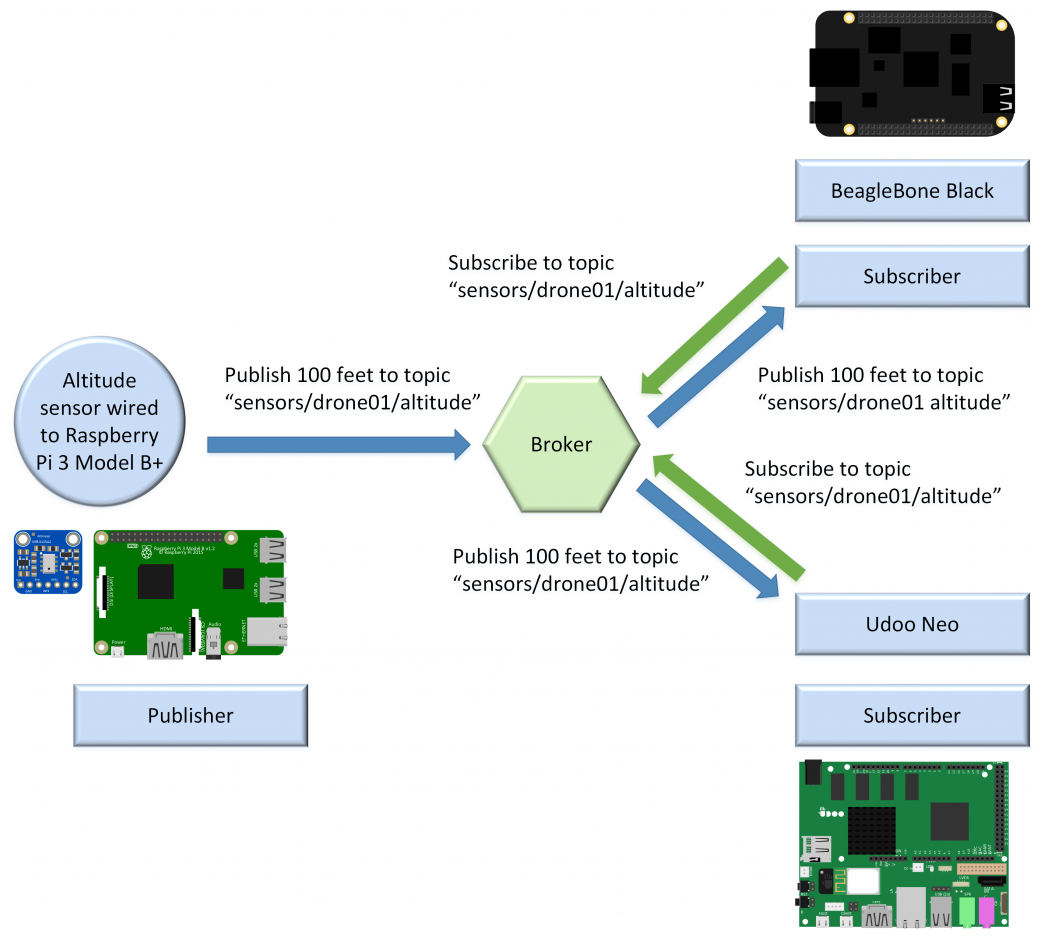
A Raspberry Pi 3 Model B+ board with an altitude sensor wired to it is a publisher that establishes a connection with the server. A BeagleBone Black board and a Udoo Neo board are two subscribers that establish a connection with the server.
The BeagleBone Black board indicates to the server that it wants to subscribe to all messages that belong to the sensors/drone01/altitude topic. The Udoo Neo board indicates the same to the server. Hence, both boards are subscribed to the sensors/drone01/altitude topic.
A topic is a named logical channel, and it is also referred to as a channel or subject. The server will send subscribers only messages published to topics to which they are subscribed.
The Raspberry Pi 3 Model B+ board publishes a message with 100 feet as the payload and sensors/drone01/altitude as the topic. This board, that is, the publisher, sends the publish request to the server.
The server distributes the message to the two clients that are subscribed to the sensors/drone01/altitude topic: the BeagleBone Black and the Udoo Neo boards.
Publishers and subscribers are decoupled in space because they don't know each other. Publishers and subscribers don't have to run at the same time. The publisher can publish a message and the subscriber can receive it later. In addition, the publish operation isn't synchronized with the receive operation.
A publisher requests the server to publish a message, and the different clients that have subscribed to the appropriate topic can receive the message at different times. The publisher can send a message as an asynchronous operation to avoid being blocked until the server receives the message. However, it is also possible to send a message to the server as a synchronous operation with the server and to continue the execution only after the operation was successful. In most cases, we will want to take advantage of asynchronous operations.
A publisher that requires sending a message to hundreds of clients can do it with a single publish operation to a server. The server is responsible for sending the published message to all the clients that have subscribed to the appropriate topic. Because publishers and subscribers are decoupled, the publisher doesn't know whether any subscriber is going to listen to the messages it is going to send. Hence, sometimes it is necessary to make the subscriber become a publisher too and to publish a message indicating that it has received and processed a message. The specific requirements depend on the kind of solution we are building. MQTT offers many features that make our lives easier in many of the scenarios we have been analyzing. We will use these different features throughout the book.