About the Book
If you’re looking for a programming language to develop flexible and efficient apps, JavaScript is a great choice. However, whilst offering real benefits, the complexity of the entire JavaScript ecosystem can be overwhelming. This Workshop is a smarter way to learn JavaScript. It is specifically designed to cut through the noise and help build your JavaScript skills from scratch, while sparking your interest with engaging activities and clear explanations.
Starting with explanations of JavaScript’s fundamental programming concepts, this book will introduce the key tools, libraries and frameworks that programmers use in everyday development. You will then move on and see how to handle data, control the flow of information in an application, and create custom events. You’ll explore the differences between client-side and server-side JavaScript, and expand your knowledge further by studying the different JavaScript development paradigms, including object-oriented and functional programming.
By the end of this JavaScript book, you’ll have the confidence and skills to tackle real-world JavaScript development problems that reflect the emerging requirements of the modern web.
Audience
Our goal at Packt is to help you be successful, in whatever it is you choose to do. The JavaScript Workshop is an ideal tutorial for the JavaScript beginner who is just getting started. A basic understanding of HTML and CSS will help you to get the best out of this Workshop. Pick up a Workshop today and let Packt help you develop skills that stick with you for life.
About the Chapters
Chapter 1, Getting to Know JavaScript, introduces a foundational background of JavaScript in order to move forward and achieve competency. This chapter introduces you to JavaScript, through its history through to modern implementations, with additional information on various uses of the language to provide a proper context for what comes next.
Chapter 2, Working with JavaScript, covers some hands-on work with the language. We'll provide an overview of some popular tools for writing JavaScript and the various available runtimes for executing your code. We'll pay particular attention to the primary target for most JavaScript applications and a great tool in itself, the modern web browser.
Chapter 3, Programming Fundamentals, serves as an introduction to the fundamental concepts and structures involved when working in JavaScript and programming in general. We'll cover all the basics, from object types to conditionals and looping structures, how to go about writing and invoking functions, and even commenting and debugging your code.
Chapter 4, JavaScript Libraries and Frameworks, focuses on plain-old vanilla JavaScript, along with various frameworks and libraries that exist today. The primary focus of this chapter is to provide an understanding that, while extensions to the core language can be great, sometimes, core JavaScript is really all you need.
Chapter 5, Beyond the Fundamentals, explains that data is represented differently in different languages and runtimes. JavaScript is based on the ECMAScript specification and has definitive rules for how data is represented. This chapter discusses data in JavaScript, how to convert between types, and how types are passed around within a script.
Chapter 6, Understanding Core Concepts, utilizes an HTML page using JavaScript in this book, as well as being the first chapter to explain the abstract nature of the event messaging system. Understanding these concepts is very valuable when building a useful web application in JavaScript. Throughout this chapter, you will explore the various nuances of event message bubbling and capturing, along with how it can best be used to control the flow of information within an application. You will also see how to halt these events in their tracks and to create your own custom events. This chapter will give you the foundations to tackle applications of any size or complexity.
Chapter 7, Popping the Hood, clarifies how the thing many people think of as 'just JavaScript' can actually be broken down into separate components: the JavaScript engine, consisting of the call stack, memory heap, and the garbage collector; and the JavaScript runtime environment, such as a browser, or Node.js, which contains the JavaScript engine, and gives the engine access to additional functions and interfaces, such as setTimeout()
or a filesystem interface. We will also look at how JavaScript manages memory allocation and deallocation, and how even though it is managed automatically, it's important for developers to bear in mind the processes involved, in order to write code that enables the garbage collector to work correctly.
Chapter 8, Browser APIs, introduces a few of the most useful and interesting browser APIs that open up wide-ranging functionality that we can make use of in our JavaScript application. We'll see that while these APIs are most commonly accessed through JavaScript, they are not a part of the ECMAScript specification to which the JavaScript engines are programmed and are not part of JavaScript core functionality.
Chapter 9, Working with Node.js, guides us in unifying the entire web application development around a single programming language, as opposed to learning different languages and building different projects for server-side and client-side. In this chapter, you will go through how the node works in the background and how it processes requests asynchronously. Furthermore, you will study different types of modules and how to use them.
Chapter 10, Accessing External Resources, explores the fact that web pages are static and of limited use without fresh data. This chapter covers various approaches to using Ajax to obtain data, primarily from RESTful services.
Chapter 11, Creating Clean and Maintainable Code, introduces you to best practices for clean and maintainable coding. You will learn that refactored code that uses clean coding techniques results in code that is much longer than before. But you will see that the code is much cleaner and easier to understand and test compared to the original. The value of this programming style really shows itself more in complex real-world applications, and it is good practice to work this way. Developers and tech leads need to decide what standards and clean coding practices make sense for their particular project.
Chapter 12, Using Next-Generation JavaScript, looks at the various tools available in the market for advanced development in JavaScript. We will learn how to use the latest JavaScript syntax in older browsers and identify the different options for the development of JavaScript applications in other languages. We will also explore various package managers, such as npm and Yarn, that are compatible with JavaScript, along with several different frameworks, such as AngularJS, React, and Vue.js. Finally, we will look at some server-side libraries, such as Express, Request, and Socket.IO.
Chapter 13, JavaScript Programming Paradigms, teaches you that JavaScript is a multi-paradigm programming language. We can use it to write code in procedural, object-oriented, and functional design patterns. During the learning phase of any programming language, people usually code in a procedural way where, instead of planning, they put most of their focus on the execution and understanding the concepts of that particular programming language. But when it comes to practical execution in real life, the object-oriented programming paradigm, or OOP, is a scalable option.
Chapter 14, Understanding Functional Programming, discusses how functional programming is quite different from other programming paradigms such as imperative and object-oriented approaches, and it takes some getting used to. But, properly applied, it is a very powerful way of structuring programs to be more declarative, correct, and testable with fewer errors. Even if you don't use pure functional programming in your projects, there are many useful techniques that can be used on their own. This is especially true for the map
, reduce
, and filter
array methods, which can have many applications. The topics covered in this chapter will help you bolster the skills you need to pursue a programming project in the functional style.
Chapter 15, Asynchronous Tasks, discusses how asynchronous tasks allow the execution of the main thread of a program to proceed even while waiting for data, an event, or the result of another process, and achieve snappier UIs and some forms of multitasking. Recent enhancements to the language, such as promises and the async
/await
keywords, simplify such development and make it easier to write clean and maintainable asynchronous code.
Note
There are also two bonus chapters available at: http://packt.live/2Z6F4fT
Conventions
Code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles are shown as follows:
"The if
, else if
, and else
statements give you four structures for selecting or skipping blocks of code."
Words that you see on the screen, for example, in menus or dialog boxes, also appear in the text like this: "Press the F12 key to launch the debugger or select More Tools
| Developer Tools
from the menu."
A block of code is set as follows:
function logAndReturn( value ) { console.log("logAndReturn:" +value ); return value; } if ( logAndReturn (true) || logAndReturn (false)) { console.log("|| operator returned truthy."); }
New terms and important words are shown like this: "Timer events provide forced asynchronous functionality within your applications. They allow you to invoke a function after a period of time; either once or repeatedly."
Long code snippets are truncated and the corresponding names of the code files on GitHub are placed at the top of the truncated code. The permalinks to the entire code are placed below the code snippet. It should appear as follows:
activity.html
1 <!doctype html> 2 <html lang="en"> 3 <head> 4 <meta charset="utf-8"> 5 <title>To-Do List</title> 6 <link href=https://fonts.googleapis.com/css?family=Architects+Daughter|Bowlby+One+SC rel="stylesheet"> 7 <style> 8 body { 9 background-color:rgb(37, 37, 37); 10 color:aliceblue; 11 padding:2rem; 12 margin:0; 13 font-family:'Architects Daughter', cursive; 14 font-size: 12px; 15 }
The full code is available at: https://packt.live/2Xc9Y4o
Before You Begin
Each great journey begins with a humble step. Our upcoming adventure with JavaScript programming is no exception. Before we can do awesome things using JavaScript, we need to be prepared with a productive environment. In this short note, we will see how to do that. If you have any issues or questions about installation please email us at [email protected]
.
Installing Visual Studio Code
Here are the steps to install Visual Studio Code (VSCode):
- Download the latest VSCode from https://packt.live/2BIlniA:
Figure 0.1: Downloading VSCode
- Open the downloaded file, follow the installation steps, and complete the installation process.
Installing the "Open in Default Browser" Extension
- Open your VSCode, click on the
Extensions
icon, and type inOpen In Default Browser
in the search bar, as shown in the following screenshot:Figure 0.2: Open in Default Browser extension search
- Click on
Install
to complete the installation process, as shown in the following screenshot:
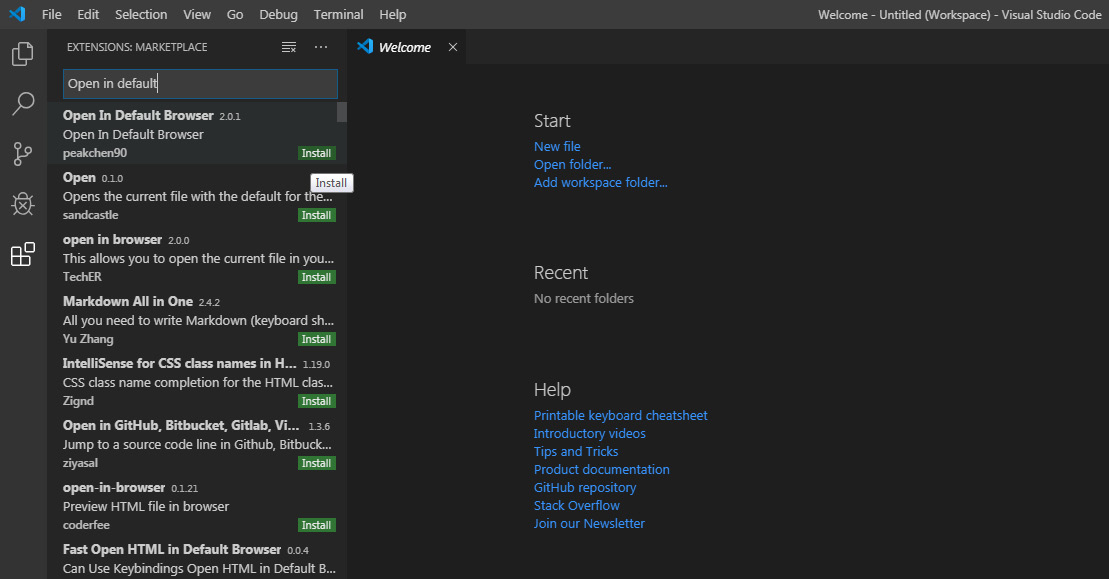
Figure 0.3: Installing the extension
Downloading Node.js
Node.js is open source and you can download it from its official website https://nodejs.org/en/download/ for all platforms. It supports all three major platforms: Windows, Linux, and macOS.
Windows
Visit their official website and download the latest stable .MSI installer. The process is very simple. Just execute the .MSI
file and follow the instructions to install it on the system. There will be some prompts about accepting license agreements. You have to accept those and then click on Finish
. That's it.
Mac
You have to download the .pkg file from the official website and execute it. Then, follow the instructions. You may have to accept the license agreement. After that, follow the prompts to finish the installation process.
Linux
In order to install Node.js on Linux, execute the following commands as root in the same order they are mentioned:
$ cd /tmp
$ wget http://nodejs.org/dist/v8.11.2/node-v8.11.2-linux-x64.tar.gz
$ tar xvfz node-v8.11.2-linux-x64.tar.gz
$ mkdir -p /usr/local/nodejs
$ mv node-v8.11.2-linux-x64./* /usr/local/nodejs
Here, you first change the current active directory to the temporary directory (tmp
) of the system. Second, you download the tar
package of the node
from their official distribution directory. Third, you extract the tar
package to the tmp
directory. This directory contains all the compiled and executable files. Fourth, you create a directory in the system for Node.js
. In the last command, you are moving all the complied and executable files of the package to that directory.
Verifying the Installation
After the installation process, you can verify whether it is installed properly in the system by executing the following command in the directory of Node.js:
$ node -v && npm -v
It will output the current installed version of Node.js and npm:
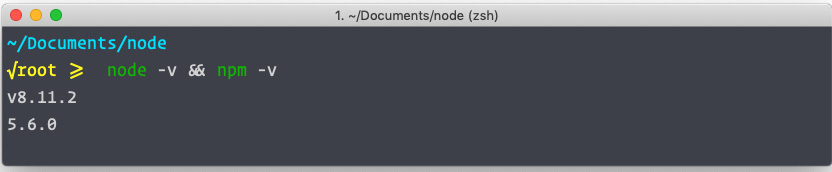
Figure 0.4: Installed version of Node.js and npm
Here, it is showing that the 8.11.2 version of Node.js is installed on the system, as is the 5.6.0 version of npm.
Installing the Code Bundle
Download the code and relevant files from GitHub at https://github.com/PacktWorkshops/The-JavaScript-Workshop and place them in a new folder called C:\Code
on your local system. Refer to these code files for the complete code bundle.