Modern browsers are slowly getting more powerful features that can be accessed directly from JavaScript. You can easily add video and audio with the new HTML5 tags and create interactive components through the use of the HTML5 canvas. A rather new addition to this feature set is the support of WebGL. With WebGL you can directly make use of the processing resources of your graphics card and create high-performance 2D and 3D computer graphics. Programming WebGL directly from JavaScript to create and animate 3D scenes is a very complex and error-prone process. Three.js is a library that makes this a lot easier. The following list shows some of the things that Three.js makes easy:
Creating simple and complex 3D geometries
Animating and moving objects through a 3D scene
Applying textures and materials to your objects
Loading objects from 3D modeling software
Creating 2D sprite-based graphics
With a couple lines of JavaScript you can create anything from simple 3D models to photorealistic real-time scenes as shown:
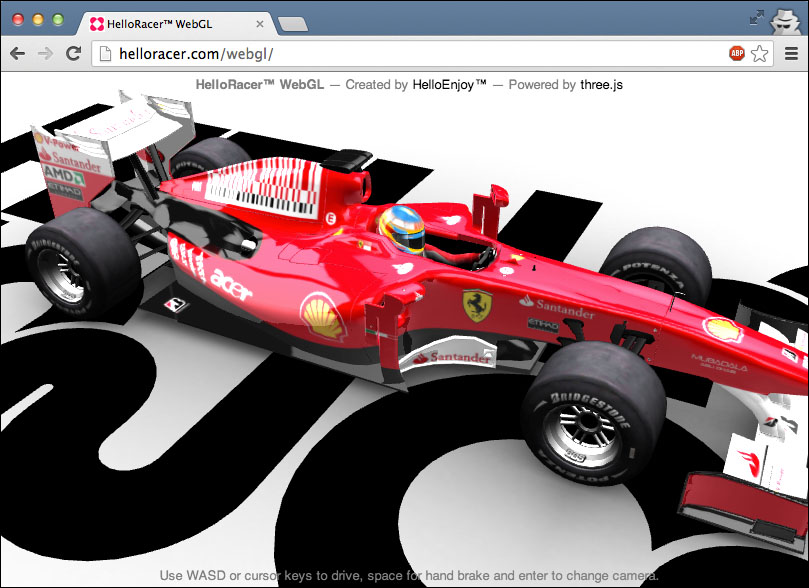
In the first chapter, we'll directly dive into Three.js and create a couple of examples that show you how Three.js works and you can use them to play around with. We won't dive into all the technical details yet; that's something you'll learn in the later chapters. In this chapter we'll cover the following points:
Tools required for working with Three.js
Downloading the source code and examples used in this book
Creating your first Three.js scene
Improving the first scene with materials, lights, and animations
Introducing a couple of helper libraries for statistics and controlling the scene
We'll start this book with a short introduction into Three.js and then quickly move on to the first examples and code samples. Before we get started, let's quickly look at the most important browsers out there and their support for WebGL.
At the moment Three.js works with the following browsers:
Browser |
Support |
---|---|
Mozilla Firefox |
Supported since Version 4.0. |
Google Chrome |
Supported since Version 9. |
Safari |
Supported since Version 5.1 and newly installed on Mac OS X Mountain Lion, Lion, and Snow Leopard. Make sure you enable WebGL in Safari. You can do this by navigating to Preferences | Advanced and checking the option Show develop menu in menu bar. After that navigate to Develop | Enable WebGL. |
Opera |
Supported since Version 12.00. You still have to enable this by opening the file |
Internet Explorer |
Internet Explorer had long been the only major player who didn't support WebGL. Starting with IE11, Microsoft has added WebGL support. |
Basically, Three.js runs in any of the modern browsers, except most versions of IE. So if you want to use an older version of IE, you've got two options: you can get WebGL support through the use of Google Chrome Frame, which you can download from the following URL: https://developers.google.com/chrome/chrome-frame/. An alternative you can use instead of Google Chrome Frame is the iewebgl
plugin, which you can get from http://iewebgl.com/. This installs inside IE and enables WebGL.
Tip
Currently the guys behind Three.js are working on a renderer that uses the new CSS-3D specification, which is supported by a lot of browsers (even IE10). Besides desktop browsers, a number of mobile and tablet browsers also support CSS-3D.
In this chapter, you'll directly create your first 3D scene and will be able to run this in any of the mentioned browsers. We won't introduce too many complex Three.js features, but at the end of this chapter you'll have created the Three.js scene that you can see in the following screenshot:
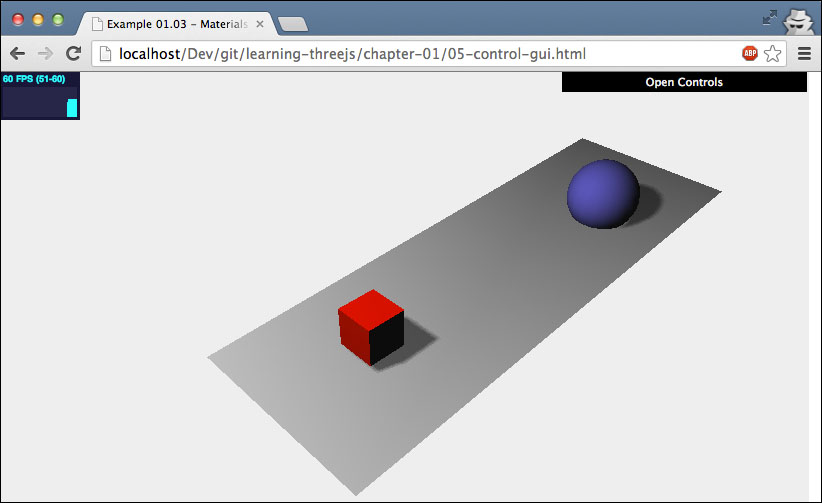
For this first scene you'll learn about the basics of Three.js and also create your first animation. Before you start your work on this example, in the next couple of sections we'll first look at the tools that you need to easily work with Three.js and how you can download the examples that are shown in this book.