The first thing we need to do is create an empty HTML skeleton page that we can use as the base for all our examples. This HTML skeleton is shown as follows:
<!DOCTYPE html> <html> <head> <title>Example 01.01 - Basic skeleton</title> <script type="text/javascript" src="../libs/three.js"></script> <script type="text/javascript" src="../libs/jquery-1.9.0.js"></script> <style> body{ /* set margin to 0 and overflow to hidden, to use the complete page */ margin: 0; overflow: hidden; } </style> </head> <body> <!-- Div which will hold the Output --> <div id="WebGL-output"> </div> <!-- Javascript code that runs our Three.js examples --> <script type="text/javascript"> // once everything is loaded, we run our Three.js stuff. $(function () { // here we'll put the Three.js stuff }); </script> </body> </html>
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
As you can see from this listing, the skeleton is a very simple HTML page, with only a couple of elements. In the <head>
element, we will load the external JavaScript libraries that we'll use for the examples. For all the examples, we'll at least load the two mentioned in this listing: Three.js and jquery-1.9.0.js
. In the <head>
element, we also add a couple of lines of CSS. These style elements remove any scroll bars when we create a full page Three.js scene. In the <body>
of this page you can see a single <div>
element. When we write our Three.js code, we'll point the output of the Three.js renderer to that element. In the previous code snippet, you can already see a bit of JavaScript. That small piece of code uses jQuery to call an anonymous JavaScript function when the complete page is loaded. We'll put all the Three.js code inside this anonymous function.
Three.js comes in two versions:
Three.min.js: This is the library you'd normally use when deploying Three.js sites on the internet. This is a minimized version of Three.js, created using UglifyJS, which is half the size of the normal Three.js library. All the examples and code used in this book are based on the Three.js
r60
project, which was released in August 2013.Three.js: This is the normal Three.js library. We will use this library in our examples, since it makes debugging much easier when you can read and understand the Three.js source code.
If we view this page in our browser, the results aren't very shocking. As you'd expect, all that you would see is an empty page:
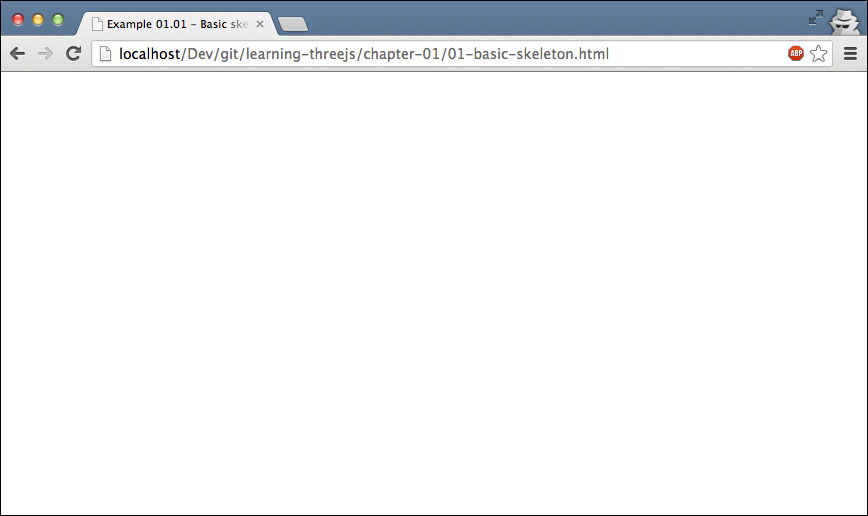
In the next section, you'll learn how to add the first couple of 3D objects and render those to the <div>
element that we had defined in our HTML skeleton page.