Now let's apply what we have learned in this chapter by stepping through an example, and see how we use D3.js to modify the DOM. The example will be the same as the one we just saw in the previous section; we'll walk through it to see how it functions.
The following is the entire entire HTML for the application:
<!DOCTYPE html> <html> <head> <meta name="description" content="D3byEX 1.1> </head> <body> <script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script> <script> d3.select('body') .append('h1') .text('Hello World!'); </script> </body> </html>
Note
bl.ock (1.1): http://goo.gl/7KkIuC
The code appends a level one header using an h1
tag to the body tag of the document. The h1
tag then has its content set to the text Hello World
. And as we saw earlier, the output in the browser looks like the following screenshot:
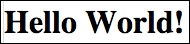
There are two primary parts to this application, both of which we will see in almost every example. The first part includes a reference to the D3.js script, which is performed with the following code placed just inside the <body>
tag:
<script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script>
This references the minified D3.js file directly from the D3.js (http://d3js.org/) website. You can also copy this file and place it locally on your web server or in a Web project. Since all the examples in this book are online, we will always use this URL.
Note that we also have to specify charset="utf-8
". This is normally not required for most JavaScript libraries, but D3.js is UTF-8 encoded and not including this can cause issues. So, make sure you don't forget this attribute.
The actual D3.js code in this example consists of the following three functions placed within another <script>
tag within the body of the document.
d3.select('body') .append('h1') .text('Hello World!');
Let's examine how this puts the text in the web page.
d3.select('body')
All D3.js statements will start with the use of the d3
namespace. This is the root of where we start accessing all the D3.js functions. In this line, we call the .select()
function, passing its body
. This is telling D3.js to find the first body element in the document and return it to us for performing other operations upon it.
The .select()
function returns a D3.js object representing the body DOM object. We can immediately call .append('h1')
to add the header element inside the body of the document.
The .append()
function returns another D3.js object, but this one represents the new h1
DOM element. So all we need to do is to make a chained call: .text('Hello World!')
, and our code is complete.
This process of calling functions in this manner is referred to in D3.js parlance as chaining, and in general, it is referred to as a fluent API in programming languages. This chaining is the aforementioned mini-language. Each chained D3.js function call further specifies the operation, allowing you to very easily describe how you want to modify the DOM through chained method calls.
This sometimes feels strange to those who have not had experience in using a fluent syntax, but once you get used to it, I guarantee that you will see the reason behind using this type of syntax. As we will see through the examples that we cover, this provides us with a very concise means of declaratively instructing D3.js on what we want in our visualization.
Note
For those familiar with jQuery, this syntax will look familiar. An equivalent piece of code could be written in JQuery as $('body').append('h1').text('Hello World');
But as we will see in more complex examples, the features provided by D3.js will give us much more power to create data visualizations than can be done with jQuery.