Let's dive a bit deeper and understand how React Native works under the hood, which will help us understand how JavaScript is compiled to a Native code and how the whole process works. It's crucial to know how the whole process works, so if you have performance issues some day, you will understand where it originates.
How the React Native bridge from JavaScript to Native world works?
Information flow
So we've talked about React concepts that power up React Native, and one of them is that UI is a function of data. You change the state, and React knows what to update. Let's visualize now how information flows through the common React app. Check out the following diagram:
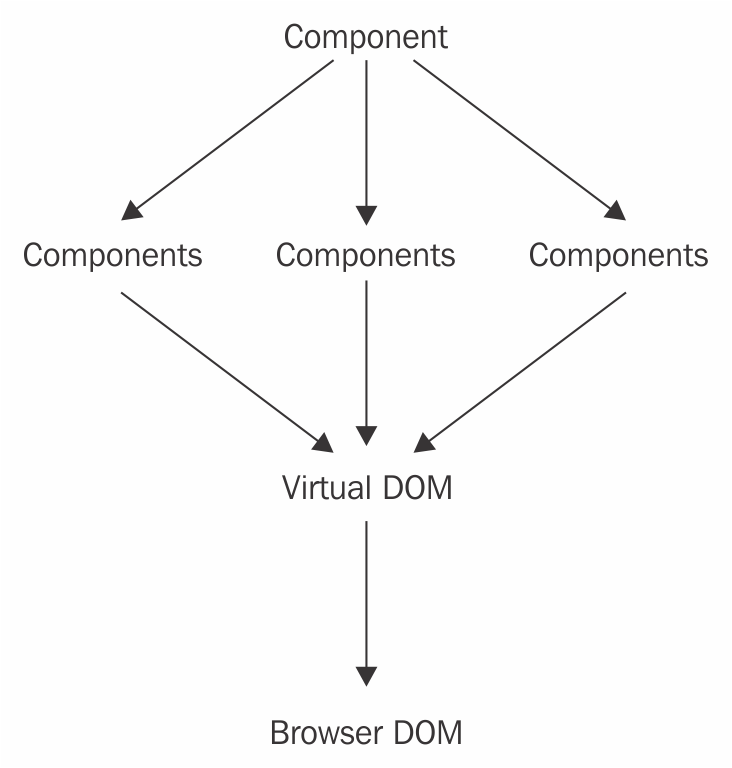
- We have a React component, which passes data to three child components
- What is happening under the hood is that a Virtual DOM tree is created, representing these component hierarchies
- When the state of the parent component is updated, React knows how to pass information to the children
- Since children are basically a representation of UI, React figures out how to batch Browser DOM updates and executes them
So now let's remove Browser DOM and think that instead of batching Browser DOM updates, React Native does the same with calls to Native modules.
So what about passing information down to Native modules? It can be done in two ways:
- Shared mutable data
- Serializable messages exchanged between JavaScript and Native modules
React Native takes the second approach. Instead of mutating data on shareable objects, it passes asynchronous serialized batched messages to the React Native Bridge. The Bridge is the layer that is responsible for gluing together Native and JavaScript environments.
Architecture
Let's take a look at the following diagram, which explains how React Native architecture is structured and take a walk through the diagram:
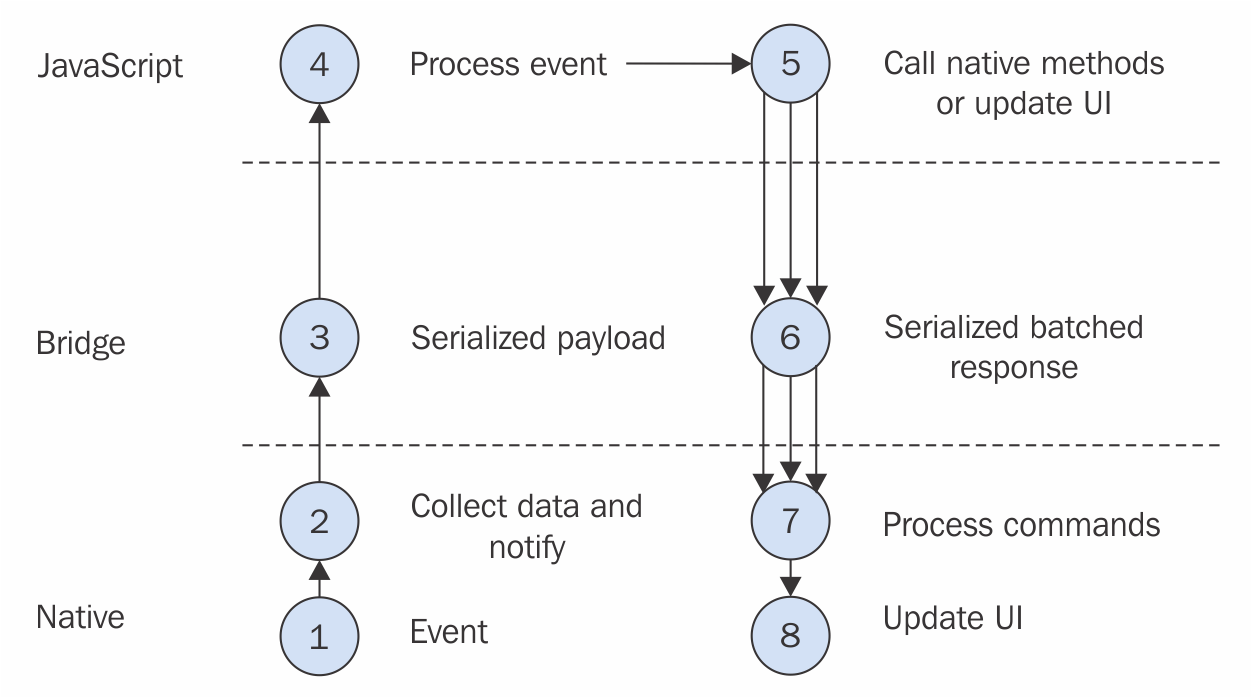
In the preceding diagram, three layers are mentioned: Native, Bridge, and JavaScript. The Native layer is pictured last in the picture because it's the layer that is closest to the device itself. The Bridge is the layer that connects JavaScript and Native modules and is basically a transport layer that transports asynchronous serialized batched response messages from JavaScript to Native modules.
When an event is executed on the Native layer--it can be a touch, timer, or network request--basically, any event involving device Native modules. Its data is collected and is sent to the Bridge as a serialized message. The Bridge passes this message to the JavaScript layer.
The JavaScript layer is an event loop. Once the Bridge passes Serialized payload to JavaScript, Event is processed and your application logic comes into play.
If you update the state, triggering your UI to rerender, for example, React Native will batch Update UI and send it to the Bridge. Bridge will pass this Serialized batched response to the Native layer, which will process all commands that it can distinguish from a serialized batched response and will Update UI accordingly.
Threading model
Up till now, we've seen that there is lots of stuff going on under the hood of React Native. It's important to know that everything is done on three main threads:
- UI (the application's main thread)
- Native modules
- JavaScript runtime
The UI thread is the main Native thread where native-level rendering occurs. It is here, where your platform of choice, iOS or Android, does measuring, layouting, and drawing.
If your application accesses any Native APIs, it's done on a separate Native modules thread. For example, if you want to access the camera, geo location, photos, and any other Native API, planning and gestures in general are also done on this thread.
The JavaScript runtime thread is the thread where all your JavaScript application code will run. It's slower than the UI thread since it's based on a JavaScript event loop, so if you do complex calculations in your application that leads to a lot of UI changes, these can lead to bad performance. The rule of thumb is that if your UI changes slower than 16.67 ms, then your UI will appear sluggish.