React Native brings with it many advantages for mobile development. We've covered some of them briefly before, but let's go over them now in more detail. These advantages are what has made React Native so popular and why it is trending now. Also, most of all, it helped web developers to start developing Native apps with a relatively short learning curve compared to overhead learning for Objective-C and Java.
The benefits of React Native
Developer experience
One of the amazing changes React Native brings to the mobile development world is enhancement of the developer experience. If we check the developer experience from the point of view of web developer, it's awesome. For a mobile developer, it's something that every mobile developer has dreamt of. Let's go over some of the features React Native brings for us out of the box.
Chrome DevTools debugging
Every web developer is familiar with Chrome Developer tools. These tools give us an amazing experience when debugging web applications. In mobile development, debugging mobile applications can be hard. Also, it's really dependent on your target platform. None of mobile application debugging techniques even come near the web development experience.
In React Native, we already know that a JavaScript event loop is running on a separate thread, and it can be connected to Chrome DevTools. By clicking on Ctrl/Cmd+D in an application simulator (we will cover all the steps of setting such a simulator in future chapters), we can attach our JavaScript code to Chrome DevTools and bring web debugging to a mobile world. Let's take a look at the following screenshot:
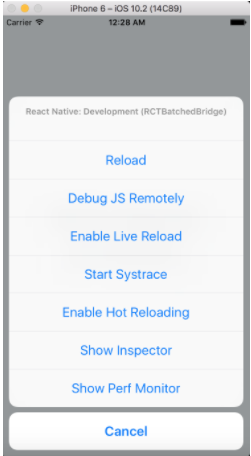
Here, you see a React Native debug tools. By clicking on Debug JS Remotely, a separate Google Chrome window is opened, where you can debug your applications by setting breakpoints, profiling CPU and memory usage, and much more.
The Elements tab in Chrome Developer tools won't be relevant, though. For that, we have a different option. Let's take a look at what we will get with Chrome Developer tools Remote debugger.
Currently, Chrome Developer Tools are focused on the Sources tab. You can note that JavaScript is written in ECMAScript 2015 syntax. For those of you who are not familiar with React JSX, you see a weird XML-like syntax. Don't worry, this syntax will also be covered in the book in the context of React Native.
If you put debugger inside your JavaScript code, or a breakpoint in your Chrome development tools, the app will pause on this breakpoint or debugger, and you will be able to debug your application while it's running.
Live reload
As you can see in the React Native debugging menu, the third row says Live Reload. If you enable this option, whenever you change your code and save, the application will be automatically reloaded.
This ability to live reload is something mobile developers only dream of. There is no need to recompile an application after each minor code change. Just save, and the application will reload itself in the simulator. This greatly speeds up application development and makes it much more fun and easy than conventional mobile development. The workflow for every platform is different, whereas the experience is the same in React Native--the platform for which you develop does not matter.
Hot reload
Sometimes, you develop a part of the application that requires several user interactions to get to. Consider, for example, logging in, opening the menu, and choosing an option. When we change our code and save, while live reload is enabled, our application is reloaded and we need to do these steps once again.
However, it does not have to be like that. React Native gives us an amazing experience of hot reloading. If you enable this option in React Native development tools and if you change your React Native component, only the component will be reloaded while you stay on the same screen you were on before. This speeds up the development process even more.
Component hierarchy inspections
I've said before that we cannot use the elements panel in Chrome development tools, but how do you inspect your component structure in React Native apps? React Native gives us a built-in option in development tools called Show Inspector. When you click on it, you will get the following window:
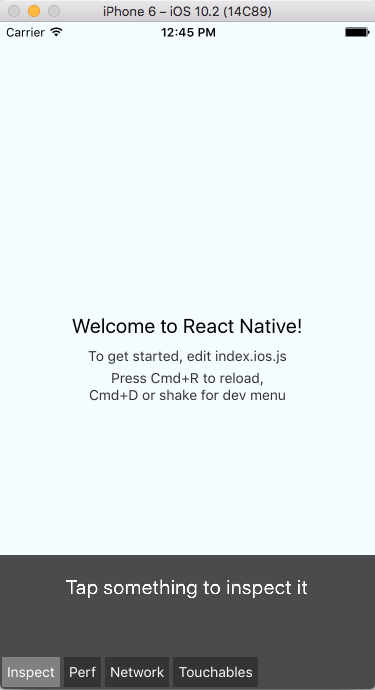
After the Inspector is opened, you can select any component on the screen and inspect it. You will get the full hierarchy of your components as well as their styling:
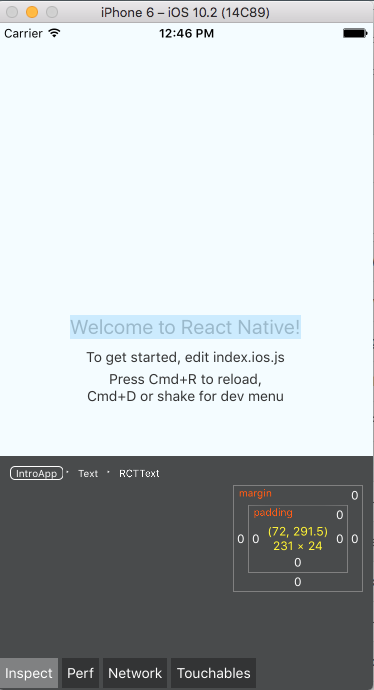
In this example, I've selected the Welcome to React Native! text. In the opened pane, I can see its dimensions, padding margin, and component hierarchy. As you can see, it's IntroApp/Text/RCTText.
RCTText is not a React Native JavaScript component, but a Native Text component, connected to React Native bridge. In that way, you also can see that this component is connected to a Native text component.
There are even more dev tools available in React Native that I will cover later on, but we can all agree that the development experience of React Native is outstanding.
Web-inspired layout techniques
Styling for Native mobile apps can be really painful sometimes. Also, it's really different between iOS and Android. React Native brings another solution. As you may have seen before, the whole concept of React Native is about bringing the web development experience to mobile app development.
That's also the case for creating layouts. The modern way of creating a layout for the web is using flexbox. React Native decided to adopt this modern technique for the web and bring it to the mobile world with a few differences.
In addition to layouting, all styling in React Native is very similar to using inline styles in HTML.
Let's take a look at an example:
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
});
As you can see in this example, there are several properties of flexbox used as well as a background color. This really reminds us of CSS properties; however, instead of using background-color, justify-content, and align-items, CSS properties are named in a camel case manner in order to apply these styles to the Text component, for example. It's enough to pass them as follows:
<Text styles={styles.container}>Welcome to React Native </Text>
Styling will be discussed in the next chapters; however, as you can see from the preceding example, styling techniques are similar to web techniques. They are not dependent on any platform and are the same for both iOS and Android.
Code reusability across applications
In terms of code reuse, if an application is properly architectured (something that you will also learn in this book), around 80% to 90% of code can be reused between iOS and Android. This means that in terms of development speed, React Native beats mobile development.
Sometimes, even code used for the web can be reused in a React Native environment with small changes. This really brings React Native to the top of the list of the best frameworks to develop Native mobile apps.