.NET Framework, .NET Core, .NET Standard, and .NET Native are related and overlapping platforms for developers to build applications and services upon.
Microsoft's .NET Framework is a development platform that includes a Common Language Runtime (CLR) that manages the execution of code, and provides a rich library of classes to build applications.
Microsoft designed .NET Framework to have the possibility of being cross-platform, but Microsoft put their implementation effort into making it work best with Windows.
Practically speaking, .NET Framework is Windows-only, and a legacy platform.
Third parties developed a .NET implementation named the Mono project that you can read more about here: http://www.mono-project.com/.
Mono is cross-platform, but it fell well behind the official implementation of .NET Framework. It has found a niche as the foundation of the Xamarin mobile platform.
Microsoft purchased Xamarin in 2016 and now gives away what used to be an expensive Xamarin extension for free with Visual Studio 2017. Microsoft renamed the Xamarin Studio development tool to Visual Studio for Mac and has given it the ability to create ASP.NET Core Web API services. Xamarin is targeted at mobile development and building cloud services to support mobile apps.
Note
You will use Visual Studio for Mac in Chapter 15 , Building Mobile Apps Using Xamarin.Forms and ASP.NET Core Web API to create a mobile app for iOS and Android that calls an ASP.NET Core Web API service.
Today, we live in a truly cross-platform world. Modern mobile and cloud development has made Windows a much less important operating system. So, Microsoft has been working on an effort to decouple .NET from its close ties with Windows.
While rewriting .NET to be truly cross-platform, Microsoft has taken the opportunity to refactor .NET to remove major parts that are no longer considered core.
This new product is branded as .NET Core, which includes a cross-platform implementation of the CLR known as CoreCLR, and a streamlined library of classes known as CoreFX.
Scott Hunter, Microsoft Partner Director Program Manager for .NET, says, "Forty percent of our .NET Core customers are brand-new developers to the platform, which is what we want with .NET Core. We want to bring new people in."
The following table shows when important versions of .NET Core were released, and Microsoft's schedule for the next major release:
Version |
Released |
.NET Core RC1 |
November 2015 |
.NET Core 1.0 |
June 2016 |
.NET Core 1.1 |
November 2016 |
.NET Core 1.0.4 (LTS) and .NET Core (Current) 1.1.1 |
March 2017 |
.NET Core 2.0 |
Scheduled for release in Q3 2017 |
Tip
Good Practice
If you need to work with .NET Core 1.0 and 1.1, then I recommend that you read the announcement for .NET Core 1.1, although the information at the following URL is useful for all .NET Core developers: https://blogs.msdn.microsoft.com/dotnet/2016/11/16/announcing-net-core-1-1/
.NET Core is much smaller than the current version of .NET Framework because a lot has been removed.
For example, Windows Forms and Windows Presentation Foundation (WPF) can be used to build graphical user interface (GUI) applications, but they are tightly bound to Windows, so they have been removed from .NET Core. The latest technology used to build Windows apps is the Universal Windows Platform (UWP), and UWP is built on a custom version of .NET Core. You will learn about it in Chapter 13, Building Universal Windows Platform Apps Using XAML.
ASP.NET Web Forms and Windows Communication Foundation (WCF) are old web application and service technologies that fewer developers choose to use for new development projects today, so they have also been removed from .NET Core. Instead, developers prefer to use ASP.NET MVC and ASP.NET Web API. These two technologies have been refactored and combined into a new product that runs on .NET Core named ASP.NET Core. You will learn about ASP.NET Core MVC in Chapter 14, Building Web Applications Using ASP.NET Core MVC, and ASP.NET Core Web API in Chapter 15, Building Mobile Apps Using Xamarin.Forms and ASP.NET Core Web API.
The Entity Framework (EF) 6.x is an object-relational mapping technology to work with data stored in relational databases such as Oracle and Microsoft SQL Server. It has gained baggage over the years, so the cross-platform version has been slimmed down and named Entity Framework Core. You will learn about it in Chapter 8, Working with Databases Using Entity Framework Core.
Some common but old data types in .NET Framework have been removed from .NET Core, such as HashTable
and ArrayList
in System.Collections
, but can be added back using a separate class library or NuGet package. Some data types in .NET that are included with both .NET Framework and .NET Core have been simplified by removing some members. For example, in .NET Framework, the File
class has both a Close
and Dispose
method, and either can be used to release the file resources. In .NET Core, there is only the Dispose
method. This reduces the memory footprint of the assembly and simplifies the API you must learn.
As well as removing large pieces from .NET Framework to make .NET Core, Microsoft has componentized .NET Core into NuGet packages: small chunks of functionality that can be deployed independently.
Note
.NET Framework 4.6 is about 200 MB and must be deployed as a single unit. .NET Core 1.0 is about 11 MB. Eventually, .NET Core and all its NuGet packages may grow to hundreds of megabytes. Microsoft's primary goal is not to make .NET Core smaller than .NET Framework. The goal is to componentize .NET Core to support modern technologies and to have fewer dependencies so that deployment requires only those packages that your application needs.
The situation with .NET today is that there are three forked .NET platforms, all controlled by Microsoft:
.NET Framework
Xamarin
.NET Core
Each have different strengths and weaknesses because they are designed for different scenarios. This has led to the problem that a developer must learn three platforms, each with annoying quirks and limitations.
So, Microsoft is working on defining .NET Standard 2.0: a set of APIs that all .NET platforms must implement. At the time that I write this, in March 2017, there is .NET Standard 1.6, but only .NET Core supports it; .NET Framework and Xamarin do not.
.NET Standard 2.0 will be implemented by .NET Framework, .NET Core, and Xamarin. For .NET Core, this will add many of the missing APIs that developers need to port old code written for .NET Framework to the cross-platform .NET Core. However, Microsoft warns that some APIs will be "implemented", but throw an exception to indicate to a developer that they should not actually be used! You will learn how to handle this in Chapter 2, Speaking C#.
.NET Standard 2.0 is the near future of .NET, and it will make it much easier for developers to share code between any flavor of .NET, but we are not there yet. Microsoft says .NET Standard 2.0, and .NET Core 2.0, are scheduled for release in Q3 2017. That could mean July 1, 2017, but based on previous experience, I think late September 2017 is more realistic.
The following diagram summarizes how the three variants of .NET (sometimes known as app models) will share the common .NET Standard 2.0 and infrastructure:
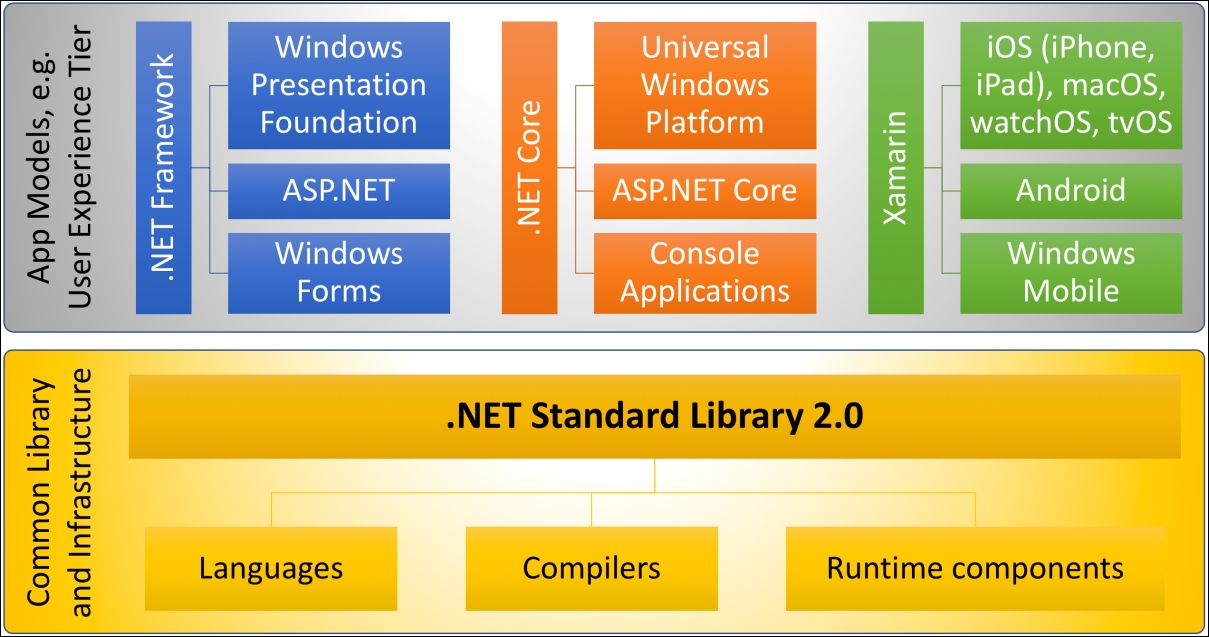
The first edition of this book focused on .NET Core, but used .NET Framework when important or useful features had not been implemented in .NET Core. Visual Studio 2015 was used for most examples, with Visual Studio Code shown only briefly.
The second edition has been purged of all .NET Framework code examples. It has been rewritten so that all code is pure .NET Core and can be written with either Visual Studio 2017 or Visual Studio Code on any supported operating system.
The only exceptions are in Chapter 13, Building Universal Windows Platform Apps Using XAML, that uses .NET Core for UWP and requires Visual Studio 2017 running on Windows 10, and in Chapter 15, Building Mobile Apps Using Xamarin.Forms and ASP.NET Core Web API, when we will write a Xamarin mobile app with Visual Studio for Mac.
Another .NET initiative is .NET Native. This compiles C# code to native CPU instructions ahead-of-time (AoT) rather than using the CLR to compile intermediate language (IL) code just-in-time (JIT) to native code later.
.NET Native improves execution speed and reduces the memory footprint for applications. It supports the following:
UWP apps for Windows 10, Windows 10 Mobile, Xbox One, HoloLens, and Internet of Things (IoT) devices such as Raspberry Pi
Server-side web development with ASP.NET Core
Console applications for use on the command line