What is a delegate?
A delegate is a type-safe method reference. It can be used to execute any method with a matching signature.
What is an event?
An event is a field that is a delegate having the
event
keyword applied. The keyword ensures that only+=
and-=
are used; this safely combines multiple delegates without replacing any existing event handlers.
How is a base class and a derived class related?
A derived class (or subclass) is a class that inherits from a base class (or superclass).
What is the difference between the
is
andas
operators?The
is
operator returnstrue
if an object can be cast to the type. Theas
operator returns a reference if an object can be cast to the type; otherwise, it returnsnull
.
Which keyword is used to prevent a class from being derived from, or a method from being overridden?
sealed
Find more information on the
sealed
keyword at https://msdn.microsoft.com/en-us/library/88c54tsw.aspx
Which keyword is used to prevent a class from being instantiated with the
new
keyword?abstract
Find more information on the
abstract
keyword at https://msdn.microsoft.com/en-us/library/sf985hc5.aspx
Which keyword is used to allow a member to be overridden?
virtual
Find more information on the
virtual
keyword at https://msdn.microsoft.com/en-us/library/9fkccyh4.aspx
What's the difference between a destructor and a deconstructor?
A destructor, also known as a finalizer, must be used to release resources owned by the object. A deconstructor is a new feature of C# 7 that allows a complex object to be broken down into smaller parts.
What are the signatures of the constructors that all exceptions should have?
The following are the signatures of the constructors that all exceptions should have:
A constructor with no parameters
A constructor with a
string
parameter usually namedmessage
A constructor with a
string
parameter, usually namedmessage
, and anException
parameter usually namedinnerException
What is an extension method and how do you define one?
An extension method is a compiler trick that makes a static method of a static class appear to be one of the members of a type. You define which type you want to extend by prefixing the type with
this
.
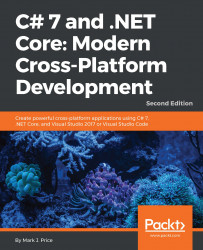
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
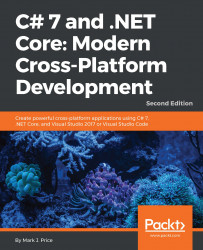
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
Overview of this book
If you want to build powerful cross-platform applications with C# 7 and .NET Core, then this book is for you.
First, we’ll run you through the basics of C#, as well as object-oriented programming, before taking a quick tour through the latest features of C# 7 such as tuples, pattern matching, out variables, and so on.
After quickly taking you through C# and how .NET works, we’ll dive into the .NET Standard 1.6 class libraries, covering topics such as performance, monitoring, debugging, serialization and encryption.
The final section will demonstrate the major types of application that you can build and deploy cross-device and cross-platform. In this section, we’ll cover Universal Windows Platform (UWP) apps, web applications, mobile apps, and web services. Lastly, we’ll look at how you can package and deploy your applications so that they can be hosted on all of today’s most popular platforms, including Linux and Docker.
By the end of the book, you’ll be armed with all the knowledge you need to build modern, cross-platform applications using C# and .NET Core.
Table of Contents (24 chapters)
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
Credits
About the Author
About the Reviewer
www.PacktPub.com
Customer Feedback
Preface
Hello, C#! Welcome, .NET Core!
Speaking C#
Controlling the Flow, Converting Types, and Handling Exceptions
Using .NET Standard Types
Debugging, Monitoring, and Testing
Building Your Own Types with Object-Oriented Programming
Implementing Interfaces and Inheriting Classes
Working with Databases Using the Entity Framework Core
Querying and Manipulating Data with LINQ
Working with Files, Streams, and Serialization
Protecting Your Data
Improving Performance and Scalability with Multitasking
Building Universal Windows Platform Apps Using XAML
Building Web Applications Using ASP.NET Core MVC
Building Mobile Apps Using Xamarin.Forms and ASP.NET Core Web API
Packaging and Deploying Your Code Cross-Platform
Answers to the Test Your Knowledge Questions
Customer Reviews