What is the maximum number of characters that can be stored in a
string
?The maximum size of a
string
variable is 2 GB or about 1 billion characters because eachchar
variable uses 2 bytes due to the internal use of Unicode (UTF-16) encoding for characters.
When and why should you use a
SecureString
?The string type leaves text data in memory for too long and it's too visible. The
SecureString
type encrypts the text and ensures that the memory is released immediately. WPF'sPasswordBox
control stores the password as aSecureString
variable, and when starting a new process, thePassword
parameter must be aSecureString
variable. For more discussion, visit:http://stackoverflow.com/questions/141203/when-would-i-need-a-securestring-in-net
When is it appropriate to use a
StringBuilder
?When concatenating more than about three
string
variables, you will use less memory and get improved performance usingStringBuilder
than usingstring.Concat
method or the+
operator.
When should you use a
LinkedList<T>
?Each item in a linked list has a reference to its previous and next siblings as well as the list itself so should be used when items need to be inserted and removed from positions in the list without actually moving the items in memory.
When should you use a
SortedDictionary
variable rather than aSortedList
variable?The
SortedList
class uses less memory thanSortedDictionary
,SortedDictionary
has faster insertion and removal operations for unsorted data. If the list is populated all at once from sorted data,SortedList
is faster thanSortedDictionary
. For more discussion, visit:
What is the ISO culture code for Welsh?
cy-GB
For a complete list of culture codes, visit: http://timtrott.co.uk/culture-codes/
What is the difference between localization, globalization, and internationalization?
Localization affects the user interface of your application. Localization is controlled by a neutral (language only) or specific (language and region) culture. You provide multiple language versions of text and other values. For example, the label of a text box might be First name in English, and Prénom in French.
Globalization affects the data of your application. Globalization is controlled by a specific (language and region) culture, for example,
en-GB
for British English, orfr-CA
for Canadian French. The culture must be specific because a decimal value formatted as currency must know to use Canadian dollars instead of French Euros.Internationalization is the combination of localization and globalization.
In a regular expression, what does
$
mean?$
represents the end of the input.
In a regular expression, how would you represent digits?
\d+
[0-9]+
Why should you not use the official standard for e-mail addresses to create a regular expression to validate a user's e-mail address?
The effort is not worth the pain for you or your users. Validating an e-mail address using official specification doesn't check whether that address actually exists or whether the person entering the address is its owner. For more discussion, visit:
http://davidcel.is/posts/stop-validating-email-addresses-with-regex/
http://stackoverflow.com/questions/201323/using-a-regular-expression-to-validate-an-email-address
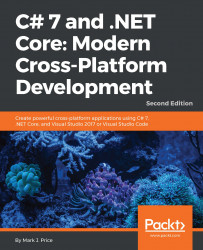
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
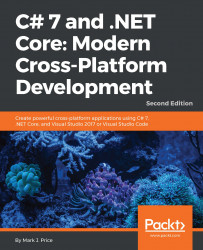
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
Overview of this book
If you want to build powerful cross-platform applications with C# 7 and .NET Core, then this book is for you.
First, we’ll run you through the basics of C#, as well as object-oriented programming, before taking a quick tour through the latest features of C# 7 such as tuples, pattern matching, out variables, and so on.
After quickly taking you through C# and how .NET works, we’ll dive into the .NET Standard 1.6 class libraries, covering topics such as performance, monitoring, debugging, serialization and encryption.
The final section will demonstrate the major types of application that you can build and deploy cross-device and cross-platform. In this section, we’ll cover Universal Windows Platform (UWP) apps, web applications, mobile apps, and web services. Lastly, we’ll look at how you can package and deploy your applications so that they can be hosted on all of today’s most popular platforms, including Linux and Docker.
By the end of the book, you’ll be armed with all the knowledge you need to build modern, cross-platform applications using C# and .NET Core.
Table of Contents (24 chapters)
C# 7 and .NET Core: Modern Cross-Platform Development - Second Edition
Credits
About the Author
About the Reviewer
www.PacktPub.com
Customer Feedback
Preface
Hello, C#! Welcome, .NET Core!
Speaking C#
Controlling the Flow, Converting Types, and Handling Exceptions
Using .NET Standard Types
Debugging, Monitoring, and Testing
Building Your Own Types with Object-Oriented Programming
Implementing Interfaces and Inheriting Classes
Working with Databases Using the Entity Framework Core
Querying and Manipulating Data with LINQ
Working with Files, Streams, and Serialization
Protecting Your Data
Improving Performance and Scalability with Multitasking
Building Universal Windows Platform Apps Using XAML
Building Web Applications Using ASP.NET Core MVC
Building Mobile Apps Using Xamarin.Forms and ASP.NET Core Web API
Packaging and Deploying Your Code Cross-Platform
Answers to the Test Your Knowledge Questions
Customer Reviews