Getting Started with PHP Web Development
PHP is a server-side scripting language. Server-side scripting is a way that web servers can respond to client requests via HTTP. The way this works is that a client (a browser) requests a URL. This request is then sent by a web server to a script. The script then reads this request and, depending on the code in the script, returns the contents of a page.
This process happens every time a web page is visited. When working with forms, data is sent from the client to the server. The data is processed and a response is returned. A common example is that on Facebook, you enter a status update and press Enter. The text is sent via a POST
request to the server, checked by the scripts on the server, and then saved to a database. The web page is then updated with the new post. PHP sites can also be API services, which may be called either from JavaScript scripts (as AJAX calls, for instance) or from other services. In those and similar cases, there is no browser request involved.
The following tools are needed for web development:
- A browser such as Google Chrome, Firefox, or Microsoft Edge.
- A text editor such as Microsoft Visual Studio Code, or an Integrated Development Environment (IDE) such as PHP Storm.
- A server to run PHP Apache or NGINX can be used, as well as PHP's built-in server.
Built-in Templating Engine
PHP was created to write applications for the web. It can be written alongside HTML to create dynamic pages. We will see examples of this in a moment.
A PHP templating engine is a way to allow PHP code to output its content alongside HTML content. This gives flexibility to pages. Any page intended to use PHP code has a .php extension instead of an .html extension. This informs the web server to expect PHP content.
A PHP file has a .php extension, and it can contain HTML, JavaScript, and CSS, along with PHP. Since the PHP interpreter needs to know where the code is placed in a PHP file, PHP code is written between two special tags (<?php
...?>
). These tags are called opening and closing tags. A typical PHP file looks like this:
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <meta name="viewport" content="width=device-width, initial-scale=1.0"> 6 <meta http-equiv="X-UA-Compatible" content="ie=edge"> 7 <title>My First PHP Page</title> 8 </head> 9 <body> 10 <div> 11 <h1>The Heading</h1> 12 <p> 13 <?php 14 // your php code goes here 15 ?>
This page starts off with HTML declaring the doctype, which tells the browser to expect HTML content, followed by meta tags that inform the browser to expect UTF-8 content and a meta tag to use the latest rendering engine and zooming levels.
Note
HTML is covered in detail later in the chapter.
Alternatively, short open tags are also available in PHP, but they are turned off by default. This can be changed by editing a .phpini
configuration file when using Apache (this goes beyond the scope of this introduction). Short codes look like this:
<? // php code here ?>
In short, opening and closing tags inform the PHP interpreter when to start and stop interpreting the PHP code line by line.
Since PHP is a useful web development tool, you will often be working in the browser. However, you will also need to be familiar with the interactive shell.
PHP in the Interactive Shell
Interactive shells are known by a few different names. On Windows, they are referred to as Command Prompt. On Linux/Mac, Terminal is the name given to the computer application that allows commands to be issued and understood by the shell and picked up by PHP.
The interactive shell allows a PHP script to run without a browser. This is how scripts are commonly executed on a server.
Exercise 1.1: Printing Hello World to the Standard Output
In this exercise, we will print a simple statement using the interactive shell. The interactive shell can be used to execute PHP code and/or scripts. Before we begin, ensure that you have followed the installation steps in the preface. Follow these steps to complete the exercise:
- Open a Terminal/Command Prompt on your machine.
- Write the following command to start PHP's interactive shell and hit Enter:
php -a
You will obtain the following output:
Figure 1.1: Getting started with the interactive shell
Interactive shell
will appear on the prompt, and it changes tophp >
. Now, you've entered in PHP's interactive shell and can run PHP code and execute scripts. We will explore more interactive shells in upcoming exercises. - Write the following command:
echo "Hello World!";
We will shortly explain what
echo
means. Once you hit Enter, you will seeHello World!
printed on the shell, as shown in the following screenshot:
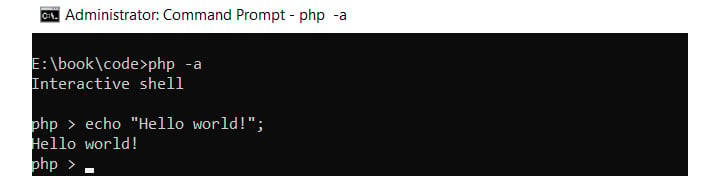
Figure 1.2: Printing output to the console
Congratulations! You have executed your first PHP code.
echo
is a PHP construct that prints anything passed to it. In the exercise, we passed Hello World!
. Since Hello World!
is a string, we have double quotes wrapped around it. You can use echo
to print strings, variables, and other things.
The echo
construct is one way to print to the screen. Another way is to use print('Hello world!')
. While this will display the string passed to it, the main difference between echo
and print
is that print
only accepts a single argument.
There are also functions that look inside a variable, such as print_r($item)
. This will output the value of any variable passed to the function. This should not be used to display a message to the screen, but instead it should be used when you need to know the contents of a variable.
One important thing to note here is the semicolon at the end of the line. In PHP, the semicolon is mandatory at the end of each statement. PHP will throw an error if a statement does not end with a semicolon.
By now, you should have got the idea that we can execute basic statements in the interactive shell. We will try some more of these later in this chapter. All the functions that we can execute in PHP scripts can be executed from the interactive shell.
Now, we will run a PHP file to output Hello World
rather than coding directly using the shell.
Exercise 1.2: Printing Hello World by Executing a PHP File
By now, you have learned how to use the echo
statement. Let's now go ahead and create your first PHP script. We will print the same statement as before, but we will use a PHP file this time. Follow these steps:
- Create a folder named
book
on your machine. Create another folder inside it namedchapter1
. It is recommended that you follow this approach for further chapters as well. - Create a file named
hello.php
inside thechapter1
folder. - Open the
hello.php
file using a code editor such as Visual Studio Code or Sublime Text. - Write the following code in
hello.php
and save it:<?php echo "Hello World!"; ?>
- Now, open the Terminal and move to the
chapter1
folder. Usecd
followed by the folder name to move into the folder. To go up a folder, use../
. - Run the following command in Command Prompt:
php hello.php
You will see
Hello World!
printed on the screen, just like in the following screenshot:

Figure 1.3: Printing output to the Terminal
First, we have PHP's opening tag. The PHP interpreter will start processing lines one by one after it. The only line of code we have here is the echo
statement to which we are passing the Hello World!
string. The PHP interpreter processes it and then this string is printed on the Terminal.
All PHP files will be written like this. Some will have HTML and other code, while some may not. Also, remember that there can be multiple opening and closing tags in a single file. These can be placed anywhere in the file.
So, you've learned how to use the interactive shell and how to print simple strings using the echo
statement. We will now learn about creating and using variables in PHP.
Assigning and Using Variables
Just as with any other programming language, variables in PHP are used to store data. A key point of difference is that all variable names in PHP must start with the dollar sign, $
.
Variables must start with a letter. They cannot start with a number or symbol, but they can contain numbers and symbols.
Data stored in variables can be of the following types:
- Integer – whole numbers
- Boolean – true or false
- Float – floating-point number
- String – letters and numbers
The data that is stored in a variable is called the value of the variable.
Creating and Assigning Variables to Print Simple Messages on the Web Browser
Consider the following example, in which we are assigning a value to a variable:
<?php $movieName = "Avengers: Endgame"; ?>
Here, a variable named $movieName
has been created, and its value is the string "Avengers: Endgame
". Since the value is a string, double or single quotes are required around it. =
is called the assignment operator. The code basically translates to the following: Assign the value on the right-hand side of the assignment operator to the variable on the left-hand side.
Here are some more examples of creating variables:
<?php $language = "PHP"; $version = 7.3; echo $language; echo $version; ?>
If you run the preceding script, you will see PHP7.3
printed. Earlier, we were directly printing values using the echo statement, but now we have assigned the values to a variable. The value is now stored in the variable. One other thing to note is that since 7.3 is a number, it does not need quotation marks.
Suppose you have "PHP" written 50 times on a page. If you had to change it to "JavaScript," you would have to replace it in all 50 places. But if the same text, "PHP", is assigned to a variable, you only need to change it once and the change will be reflected everywhere.
There are some rules that must be followed while creating variables:
- All variable names in PHP must start with the dollar sign (
$
). - A variable name cannot start with a number. It must be either a letter or an underscore. For example,
$1name
and$@name
are not valid variable names. - Only A-z, 0-9, and _ are allowed in variable names.
- Variable names are case sensitive; for example,
$name
and$NAME
are different.
Variable names must be chosen thoughtfully. They should make sense to someone else reading the code. For example, in an application, if you have to create a variable that stores a user's bank balance, a variable name such as $customerBalance
is far more obvious than $xyz
.
Unlike languages such as Java and .NET, PHP does not need to declare variables before using them. This means you can just create a variable whenever it's needed, although it's considered a best practice where possible to define your variables at the top of your scripts to make it clear their intent.
PHP also has what are called predefined variables. These are provided by PHP and are available to use by anyone.
One such variable is $argv
. This is a list of arguments passed through the Terminal by a script. Rather than executing the script on its own, you can pass values to a script that will be available to use in the $argv
variable.
Exercise 1.3: Using Input Variables to Print Simple Strings
In this exercise, we will alter the script from the previous exercise and use the input variables to print strings. Follow these steps:
- Reopen the
hello.php
file using your favorite code editor. - Replace the code with the following code and save the file:
<?php $name = $argv[1]; echo "Hello ". $name ?>
Don't worry about the syntax at the moment.
- Now, go to the Terminal inside the
chapter1
folder. - Run the following command:
php hello.php packt
You will see the following output on the Terminal:

Figure 1.4: Printing output to the console
What just happened? The hello.php
script printed the value you passed to it. Let's examine how it worked.
You passed the value packt
through the command line. This is called passing arguments. You can send multiple arguments shared by a space and these will all be available to the PHP script. But how?
Here comes $argv
. $argv
is a predefined variable, and once you execute a script, it gets filled with the values passed by the use. It is a list of values after the php
keyword on the Terminal. If no arguments are passed, the list only contains the filename. In our case, the list will have two values: hello.php
and packt
.
Coming back to the script, in the first line of code, we are assigning a value to the $name
variable. What is this value? $argv
is an array (more about that in later chapters, but basically, an array is a list of things) containing two values. With arrays, the counting begins from 0 instead of 1. So, the first value in $argv
is hello.php
, which can be taken out using $argv[0]
. We need the second value (must be character variables), hence we used $argv[1]
. Now, the packt
argument passed to the file is stored in the $name
variable.
In the second line, we are concatenating the text Hello
and the $name
variable. The dot operator (.
) is used to concatenate multiple values. After concatenation, the string becomes Hello packt
, which is then printed by the echo
statement.
Note
You can read about more predefined variables and their usage at https://packt.live/2nYJCWN.
You can use either single or double quotes for strings. However, there is a difference between them. You can use variables inside double-quoted strings, and they will be parsed. By this I mean that the value of the variable will be executed rather than simply displaying the name of the variable. On the other hand, single quotes do not do any additional parsing and display the content between the quotes as it is. For this reason, single quotes are slightly faster, and it is recommended to use them.
In the last exercise, we saw how to use the predefined $argv
variable. We will use one more predefined variable in this exercise called $_GET
. This allows information to be passed to the address bar, and PHP can read it. They are known as query strings
Query strings are key-value pairs that are separated by an ampersand (&). So, ?a=1&b=2
is also a valid query string.
Exercise 1.4: Using the Built-in Server to Print a String
In this exercise, we will use the built-in server to print Hello Packt
using the companyName=Packt
query string. This will allow you to start using the browser to view the output of your code, rather than just using the interactive shell. Follow these steps:
- Reopen the
hello.php
file using your favorite code editor. - Replace the code with the following code and save the file:
<?php $name = $_GET['companyName']; echo "Hello ". $name; ?>
- Go to the Terminal and go inside the
chapter1
folder. - Run the following command to run PHP's built-in web server:
php -S localhost:8085
- Now, open the browser and enter the following in the address bar and hit Enter:
http://localhost:8085/hello.php?companyName=Packt
You will see the following output on your screen:

Figure 1.5: Printing output to the browser
This is somewhat like the previous exercise, but rather than using the Terminal, we used the browser.
Notice the URL in the browser. After the filename, we have appended ?companyName=Packt
. The ?
denotes that what follows is a query string. In our code, a variable named companyName
with a value of Packt
is being passed to the PHP file.
Coming to the code now, in the first line, we have $_GET['companyName']
. $_GET
is also a predefined variable that is populated when any PHP string with a query string is executed. So, by using $_GET['companyName']
, we will get the value Packt
, which will be stored in the $name
variable. Remember that you can extract any value from the query string using the respective key.
The next line then combines them and displays the result on the browser.
Now that we have started to use the browser to view the output of our work, let's take a quick look at HTML. As discussed earlier, PHP and HTML are often used hand-in-hand, so an understanding of HTML will prove useful as you become more familiar with PHP.