Now that the app is running, why don't we get a firsthand experience of how the view and controller interact. Put a breakpoint on the JavaScript code inside verifyGuess
. Enter a guess and click on Verify. The breakpoint will be hit.
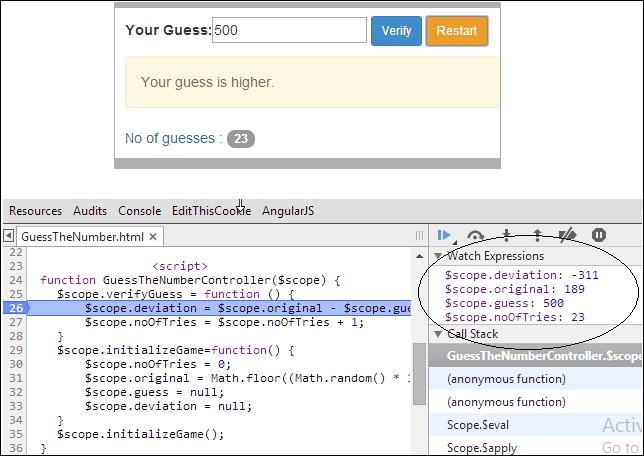
We can see that the value that we added in the input is available on the $scope
property guess
(see the Watch Expressions section in the previous screenshot). Inside the function, we use the guess
property and the original
property to calculate deviation
, and then update the noOfTries
property. As we return from the function, the view updates immediately based on model changes.
Let's try to analyze a few important aspects of an AngularJS controller:
To start with, if you are as observant as I am, you will have noticed that a scope object (
$scope
) gets passed as a parameter to the controller function (function GuessTheNumberController($scope)
), almost magically! We are nowhere calling the controller function and passing any parameter. And you may have guessed it already; if we are not doing it, then the framework must be responsible. How and when is something that we will discuss when we go into scopes in greater detail.Also, if you are more observant than me, you will realize that the controller does not reference the view! There are no DOM element references, no DOM selection, or reading or writing. The operations that the controller performs are always on the model data. As a consequence, the controller code is more readable, hence more maintainable (and, of course, testable).
Tip
When it comes to writing a controller, the golden rule is never ever, ever, ever reference the DOM element, either using plan JavaScript or with libraries such as jQuery, in the controller. Such code does not belong to the controller.
If you want to do DOM manipulations, use existing directives or create your own.
In AngularJS, unlike other frameworks, a model does not need to follow any specific structure. We do not have to derive it from any framework class. In fact, any JavaScript object and primitive types can act as a model. The only requirement is that the properties should be declared on a special object exposed by the framework called
$scope
. This$scope
object acts as glue between the model and view and keeps the model and view in sync through Angular bindings (an over-simplification). This$scope
object takes up some responsibilities that in traditional MVC frameworks belonged to the controller.
So far, we have been constantly discussing a scope object or $scope
. This is an important concept to understand as it can save us from countless hours of debugging and frustration. The next section is dedicated to learning about scopes and using them.