Scope, as we described earlier, is a JavaScript object that binds the model properties and behavior (functions) to the HTML view.
Note
An important thing to realize here is that the scope object is not the model but it references our model.
This is a special object for the framework as the complete view behavior is tied to this object. The framework creates these scope objects based on some AngularJS constructs. The framework can create multiple scope objects, depending on how the views are structured. The thing that we should keep in mind is that scope objects are always created in the context of a view element and hence follow a hierarchical arrangement similar to that of HTML elements (with some exceptions).
It will be interesting to see what scopes we have created for our app. To dig into the available scopes, we will use an excellent chrome extension Batarang.
To use Batarang, perform the following steps:
Download and install this extension from the Chrome web store.
Navigate to the app page.
Open the Chrome developer console (F12).
Click on the AngularJS tab
In this tab, enable the the Enable checkbox and we are all set!
Note
This extension does not work if the file is loaded from a local filesystem (file:///
). See issue 52 at https://github.com/angular/angularjs-batarang/issues/52. I suggest you host the HTML page on any development web server. Refer to the earlier section Setting up a development server to learn how to set up a local web server.
If we now open the Models tab, we will see two scopes: the 002 and 003 IDs (the IDs may differ in your case) organized in a hierarchical manner, as seen in the following screenshot:
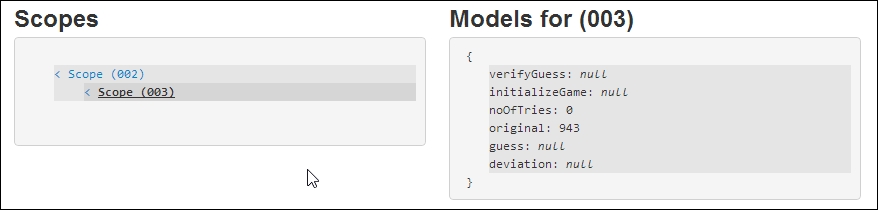
The parent scope (002) does not have any model properties but the child scope (003) contains all the properties and functions that we have been using as part of our app.
Note
Whenever we talk about the parent-child scope or scope hierarchy, it actually is an inheritance hierarchy. This implies that the child scope object inherits from the parent scope object (standard JavaScript prototypal inheritance).
It seems that we have been using this very scope (003) object to bind the view to the model. We can check this by clicking on the small < arrow that precedes each scope name. This should take us to this line in the HTML:
<div class="container" ng-controller="GuessTheNumberController">
Well, it looks like the scope (003) is tied to the previous div
element and hence is available within the start and end tags of the previous div
. Any AngularJS view construct that has been declared inside the previous div
element can access the scope (003) properties; in our case, this is the complete application HTML.
We can confirm this by copying the line containing the {{noOfTries}}
interpolation string and pasting it outside the previous div
element. The code should look something like this:
<div class="container" ng-controller="GuessTheNumberController"> <!--Truncated Code--> <p class="text-info">No of guesses : <span class = "badge"> {{noOfTries}}</span> <p> </div> <p class="text-info">No of guesses : <span class="badge">{{noOfTries}}</span><p>
If we now refresh the app, we will see two lines that should be tracking the number of tries. Try to guess the number the first tracker will increment but there is no effect on the second one. Please refer to the following screenshot:
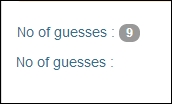
Interesting! Isn't it? And it implies that all scope objects are constrained/scoped.
Angular does not create these scopes at random. There is a reason why the Guess the Number! app has two scopes. The next section on app bootstrapping covers the underlying cause of multiple scope creation.
We have not talked about the parent scope (002) till now. If we try to click the < link next to 002 scope, it navigates to the <body>
tag with the ng-app
directive. This in fact is the root scope and was created as part of application bootstrapping. This scope is the parent of all scope objects that are created during the lifetime of applications and is hence called $rootScope
. We will throw some more light on this scope object in the next section where we talk about the app initialization process.
Let's summarize the key takeaways from our discussion on scope:
Scope objects bind the view and model together.
Scope objects are almost always linked to a view element.
There can be more than one scope object defined for an application. In fact, in any decent size app there are a number of scopes active during any given time.
More often than not, child scopes inherit from their parent scope and can access parent scope data.
This was a gentle introduction to scopes and I believe now we should have some basic understanding of scopes. There is more to scopes that we will be covering in the coming chapters. Nonetheless, this should be enough to get us started.
The last topic that we will cover in this chapter is the app initialization process. This will help us understand how and when these scope objects are created and linked to the view HTML.