Working with comments
You might have noticed that LearningCurve
has an odd line of text (10 in Figure 2.6) starting with two forward slashes, which were created by default with the script.
These are code comments! In C#, there are a few ways that you can use to create comments, and Visual Studio (and other code editing applications) will often make it even easier with built-in shortcuts.
Some professionals wouldn't call commenting an essential building block of programming, but I'll have to respectfully disagree. Correctly commenting out your code with meaningful information is one of the most fundamental habits a new programmer can develop.
Single-line comments
The following single-line comment is like the one we've included in LearningCurve
:
// This is a single-line comment
Visual Studio doesn't compile lines starting with two forward slashes (without empty space) as code, so you can use them as much as needed to explain your code to others or your future self.
Multi-line comments
Since it's in the name, you'd be right to assume that single-line comments only apply to one line of code. If you want multi-line comments, you'll need to use a forward slash and an asterisk, (/*
and */
as opening and closing characters respectively) around the comment text:
/* this is a
multi-line comment */
You can also comment and uncomment blocks of code by highlighting them and using the Cmd + / shortcut on macOS and Ctrl + K + C on Windows.
Visual Studio also provides a handy auto-generated commenting feature; type in three forward slashes on the line preceding any line of code (variables, methods, classes, and more) and a summary comment block will appear.
Seeing example comments is good, but putting them in your code is always better. It's never too early to start commenting!
Adding comments
Open up LearningCurve
and add in three backslashes above the ComputeAge()
method:
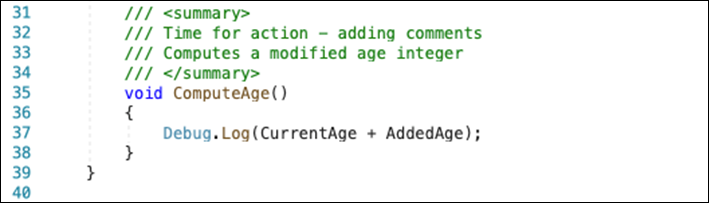
Figure 2.8: Triple-line comment automatically generated for a method
You should see a three-line comment with a description of the method generated by Visual Studio from the method's name, sandwiched between two <summary>
tags. You can, of course, change the text, or add new lines by hitting Enter just as you would in a text document; just make sure not to touch the <summary>
tags or Visual Studio won't recognize the comments correctly.
The useful part about these detailed comments is clear when you want to know something about a method you've written. If you've used a triple forward slash comment, all you need to do is hover over the method name anywhere it's called within a class or script, and Visual Studio will pop your summary:
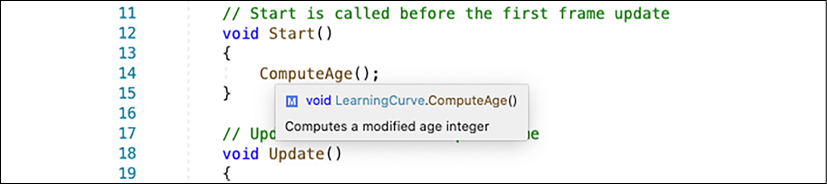
Figure 2.9: Visual Studio pop-up info box with the comment summary
Your basic programming toolkit is now complete (well, the theory drawer, at least). However, we still need to understand how everything we've learned in this chapter applies in the Unity game engine, which is what we'll be focusing on in the next section!