Another form of human-computer interaction is the keyboard. Next to the mouse, this is also one of the best-known devices to interact with computers. You can easily detect when a user presses a key, or releases it again, with Processing. One of the great things is that you can assign keys programmatically to execute pieces of code for you. This is one of the easiest ways to create a simple user-interface with Processing. For instance, you could use the D key to toggle a debug mode in your app, or the S key to save the drawing you made as an image.
We'll start by declaring some variables and writing the setup()
and draw()
functions. In this recipe, we'll write a basic Processing sketch that will change the values of the variables we've declared when we press certain keys on the keyboard.
int x; int y; int r; color c; boolean drawStroke; void setup() { size( 480, 320 ); smooth(); strokeWeight( 2 ); x = width/2; y = height/2; r = 80; c = color( 255, 0, 0 ); drawStroke = true; } void draw() { background( 255 ); if ( drawStroke == true ) { stroke( 0 ); } else { noStroke(); } fill( c ); ellipse( x, y, r*2, r*2 ); }
The next code we'll write are the functions that will take care of the keyboard events. There are three functions we can use: keyPressed()
, keyReleased()
, and
keyTyped()
.
void keyPressed() { if ( key == CODED ) { if ( keyCode == RIGHT ) { x += 10; } else if ( keyCode == LEFT ) { x -= 10; } else if ( keyCode == UP ) { y -= 10; } else if ( keyCode == DOWN ) { y += 10; } } x = constrain( x, r, width-r ); y = constrain( y, r, height-r ); } void keyReleased() { switch ( key ) { case 'r': c = color( 255, 0, 0 ); break; case 'g': c = color( 0, 255, 0 ); break; case 'b': c = color( 0, 0, 255 ); break; case 'c': c = color( 0, 255, 255 ); break; case 'm': c = color( 255, 0, 255 ); break; case 'y': c = color( 255, 255, 0 ); break; default: break; } }
void keyTyped() { if ( key == 's' ) { drawStroke = !drawStroke; } }
The result of this application looks as shown in the following screenshot:
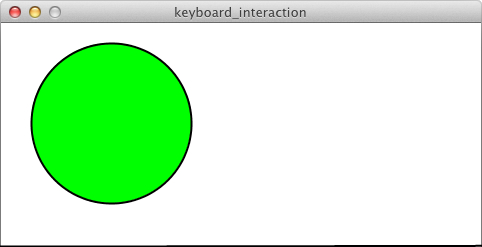
You can use the arrow keys to move the ball around. The S key will toggle the stroke. The R, G, B, C, M, and Y keys will change the color of the ball.
There are three different functions that catch key events in Processing: keyPressed()
, keyReleased()
, and keyTyped()
. These functions behave a little differently. The keyPressed()
function is executed when you press a key. You should use this one when you need direct interaction with your application. The
keyReleased()
function is executed when you release the key. This will be useful when you hold a key and change something in your running application when the key is released. The keyTyped()
function behaves just like the keyPressed()
function, but ignores all special keys such as the arrow keys, Enter, Ctrl, and Alt.
The system variable
key
contains the value of the last key that was pressed on the keyboard.The system variable
keyCode
is used to detect when special keys such as Shift, Ctrl, or the arrow keys are pressed. You'll most likely use this one within an if-statement that checks if the key isCODED
, just like you did in thekeyPressed()
function in the example. The value ofkeyCode
can beUP
,DOWN
,LEFT
,RIGHT
,ALT
,CONTROL
,SHIFT
,BACKSPACE
,TAB
,ENTER
,RETURN
,ESC
, orDELETE
.The system variable
keyPressed
is a boolean variable. The value of this variable is true if a key on the keyboard is pressed and false if no keys are pressed. This is a handy variable to use inside thedraw()
function.The
keyPressed()
function is executed once when you press a key.The
keyReleased()
function is executed once when you release a key.The
keyTyped()
function is executed when you type a key. Keys like Alt, Ctrl, or Shift are ignored by this function.
You've just learned how to react to single key presses. If you want to do something when a user presses multiple keys (shortcuts such as Ctrl + S to save an image), it won't work with these standard functions. There is an excellent article on the Processing Wiki that describes strategies for detecting multiple key presses at http://wiki.processing.org/w/Multiple_key_presses.