Let's start the demonstration by following these steps:
- Open the MainWindow.xaml page, and add two radio buttons and one button on it. The first radio button will cause an exception handled in a try {} catch {} block, whereas the second radio button will throw an exception that will go unhandled. Add the following code into your MainWindow.xaml:
<Window x:Class="CH01.UnhandledExceptionDemo.MainWindow" xmlns=
"http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="UnhandledException Demo" Height="120" Width="400"> <Grid Margin="10"> <StackPanel Orientation="Vertical"> <RadioButton x:Name="radioOne" GroupName="type" Content="Handle in Try/Catch Block" IsChecked="True" Margin="4"/> <RadioButton x:Name="radioTwo" GroupName="type" Content="Handle in Unhandled Block" IsChecked="False" Margin="4"/> </StackPanel> <Button Content="Throw Exception" Width="120" Height="30" VerticalAlignment="Top" HorizontalAlignment="Right" Margin="10" Click="OnThrowExceptionClicked"/> </Grid> </Window>
- Open the MainWindow.xaml.cs file to add the button-click event handler. Add the following code block inside the class:
private void OnThrowExceptionClicked(object sender, RoutedEventArgs e) { if (radioOne.IsChecked == true) { try { throw new Exception("Demo Exception"); } catch (Exception ex) { MessageBox.Show("'" + ex.Message + "' handled in Try/Catch block"); } } else { throw new Exception("Demo Exception"); } }
- Go to the App.xaml.cs file and override the OnStartup method to have the application level DispatcherUnhandledException event registered as shown in the following code:
protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); DispatcherUnhandledException += OnUnhandledException; }
- Add the DispatcherUnhandledException event handler into the App.xaml.cs and handle the exception as shown in the following code, but with an empty code block:
private void OnUnhandledException(object sender, DispatcherUnhandledExceptionEventArgs e) { }
- Let's build and run the application. You will see the following UI on the screen:
- It will have two radio selectors and one button in the application window. When the first radio button is checked and you click on the Throw Exception button, it will generate an exception in a try {} block, which will then immediately be handled by the associated catch {} block without crashing the application. The following message box will be shown on the UI:
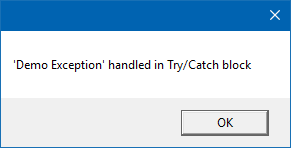
- For the second radio button, when checked, if you click on the Throw Exception button, the exception will go unhandled and will be caught in the App.xaml.cs file, under the OnUnhandledException event, and the application will crash:
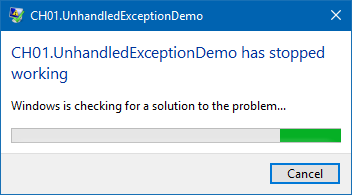
- Open the App.xaml.cs once again and modify the OnUnhandledException event implementation, as follows, to handle the thrown exception:
private void OnUnhandledException(object sender, DispatcherUnhandledExceptionEventArgs e) { e.Handled = true; }
- Now run the application once again, check the second radio button and click on the button. You will notice that the application will not crash this time.
- Click the Throw Exception button multiple times. The application will continue as-is, without causing any crash of the UI.