Object-oriented programming is a programming paradigm. It added a new way to manage code by using the concept of object as opposed to procedural programming where code follow the reading order. By using OOP, you gain reusability of code. The main gain is related to encapsulation or separation of concern. Each object has his own life.
To understand object orientation, let's take a simple example—animals. Your cat is a class. If you have four cats, you have four objects of type cat. Each of them has different properties name, age and sex values. A cat can move. In OOP terms, move is a method of the class cat.
Let's review some questions that can help you understand keywords and concepts we will implicitly use.
An object can be considered a thing that can perform actions and has properties. The action defines the object's behavior. For instance, the cat can jump. Your cat's age is eight so the property value is 8.
In pure OOP terms, an object is an instance of a class.
In an OpenLayers context, imagine you want a side-by-side map where you prepared a div with id
attribute equal to map1
and another one for the id
attribute with the value map2
. You will use the JavaScript new operator to declare two instances of the ol.Map
class like following:
var map1 = new ol.Map({ target: 'map1'}); var map2 = new ol.Map({ target: 'map2'});
An instance means that each object based on the class has it own properties values.
In our example, the target
property is different for the map1
and map2
objects.
A class can be considered as an extensible program-code-template.
When you already know OOP, you use the word class to declare it but in JavaScript, the class is known as a function. Also, functions are used as constructors.
The following excerpt from OpenLayers illustrates how a class looks:
ol.Map = function(options) { }
We also recommend you inspect the full ol.Map
class at http://openlayers.org/en/v3.0.0/apidoc/map.js.html after reviewing the following information box to discover on your own the class methods and properties.
Note
We will abusively use classes to describe object-oriented programming but the truth is that JavaScript is based on prototypes and not classes. We chose to simplify the explanation as more people understand OOP based on classes. The main goal is to explain inheritance in particular. To learn the difference. we recommend going to https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Details_of_the_Object_Model for more.
To instantiate an object, you need to use the new
keyword on a JavaScript function.
For example, when using new ol.Map()
, the function ol.Map
is called the constructor. It's the function you use with the new
keyword to create an object. You can give options to the constructor.
Note
There are other ways to create an object but to keep thing simple, we chose to restrict our explanation to the most common way when using the OpenLayers library. If you want to go further, go to https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Working_with_Objects.
Inheritance is the ability of a new class to be created, from an existing class by extending it. With this, you use the DRY (Don't repeat Yourself) principle. For example, you can reuse method or properties from a parent class. Don't sweat, it's time to explain!
Imagine after describing your cat, you also want to describe your dog in OOP.
Cats and dogs are not the same but they share some characteristics like their name, their sex or their age or they ability to move. However, a cat can jump from windows whereas a dog can't. We don't want to maintain two classes because they have common properties. You can achieve this by using a new class called animal. It can be represented like the following diagram:
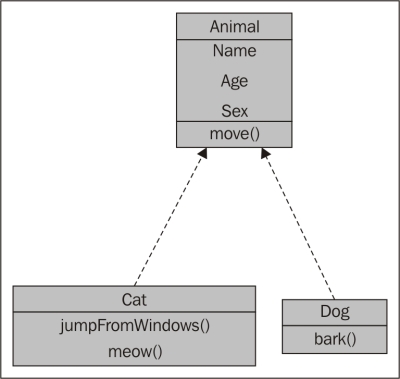
In your code, instead of declaring every common property and method twice, you declare that your Cat
class inherits from the Animal
class, so you can reuse properties and methods from the parent class. Cat
and Dog
are subclasses of the Animal
class. You can also says that the Cat
class extends the Animal
class.
Why do you think it's useful to review this abstract concept?
It's because you need to figure out the relations between OpenLayers 3 library components. The library heavily uses inheritance and for creating your own components, it's a requirement.
It's a class you use to define properties for other class that need to inherit it properties and / or methods but you never directly instantiate this class. The animal can be considered as an abstract class. However, in OpenLayers, the ol.source.XYZ
isn't an abstract class, although ol.source.OSM
is its child class.
Namespace helps you to organize your code by grouping it logically, and also by separating variables from the global.
You can declare a namespace with something like below:
var app = { main: "" }
or
var app = {}; app.main = {};
The ol.*
classes in the API documentation illustrate the namespace purpose http://openlayers.org/en/v3.0.0/apidoc/.
Methods are actions you can use within the object. Getters and setters are special types of methods. A setter's purpose is to set property within the object, whereas a getter is to get property from the object.
In OpenLayers, most classes inherit from the ol.Object
class. This class is fundamental for using setters and getters within the library. The excerpt from the official documentation is quite clear about them:
"Classes that inherit from this have predefined properties, to which you can add your own. The pre-defined properties are listed in this documentation as Observable Properties, and have their own accessors; for example,
ol.Map
has atarget
property, accessed withgetTarget()
and changed withsetTarget()
. However, not all properties are settable. There are also general-purpose accessors,get()
andset()
. For example,get('target')
is equivalent togetTarget()
."
Let's have a look at the API and understand how it uses the OOP concepts.
After reviewing the most important principles, let's inspect the API to sort out how to analyze it with OOP concepts.
For this, we will reuse the ol.source.XYZ
API documentation, http://openlayers.org/en/v3.0.0/apidoc/ol.source.XYZ.html. First, let's start with the content on top of the page.
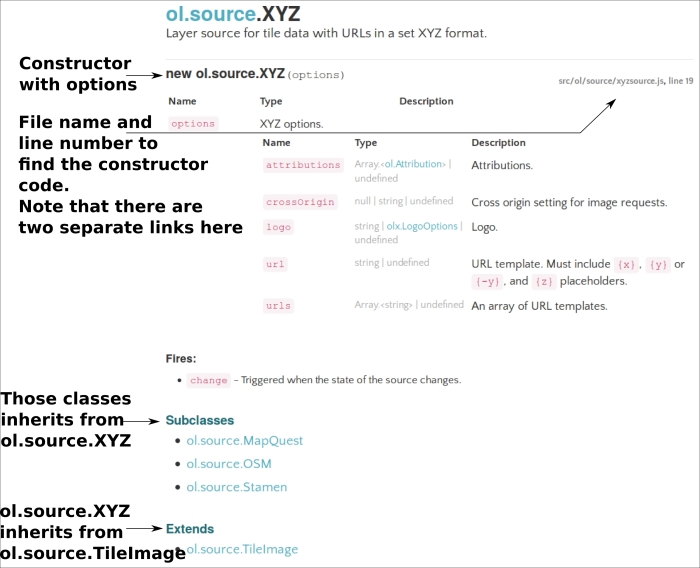
The illustration above is self-explanatory. You should follow the link for the constructor function, the subclasses to inspect properties, and methods subclasses inherits and discover provided properties and methods from ol.source.TileImage
.
Using the following screenshot, you will be able understand inheritance:
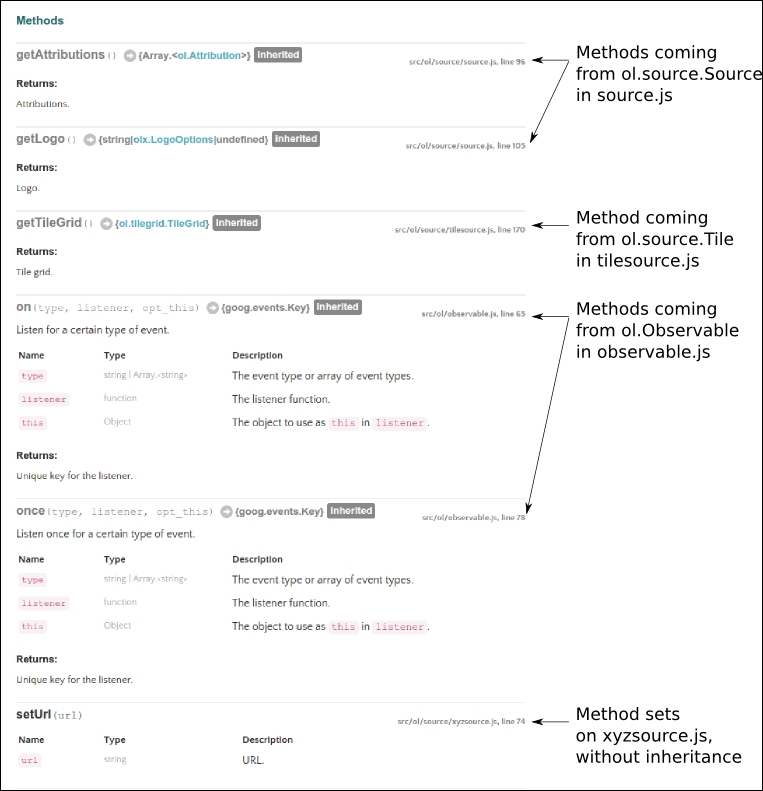
Looking at the screenshot, we are able to figure out most of the inheritance. We deduced the exact class inheritance by hovering over the inherited keyword with the mouse to see the URL that gives us a hint about it.
Within the previous screenshot, for readability, we had to remove the full ol.source.XYZ
class description. If we include and follow all the available methods, the relationships between ol.source.XYZ
class methods and properties and their parent and child classes, the result will be as follows:
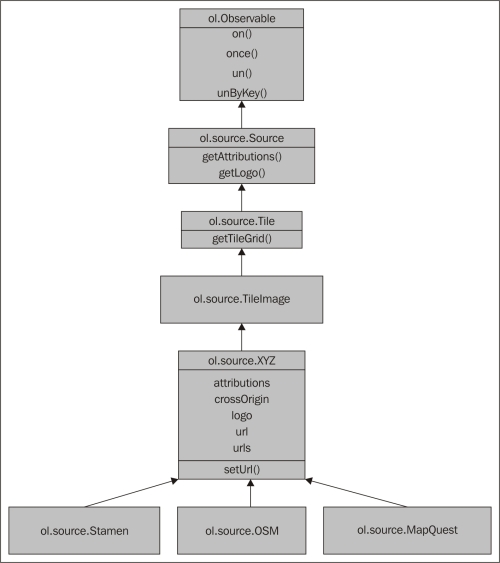