The more we can learn without installing tools the better it is to lower the learning entry. So, we will use the Closure Compiler online tool. Go to the official URL, http://closure-compiler.appspot.com and you will get the following screenshot:
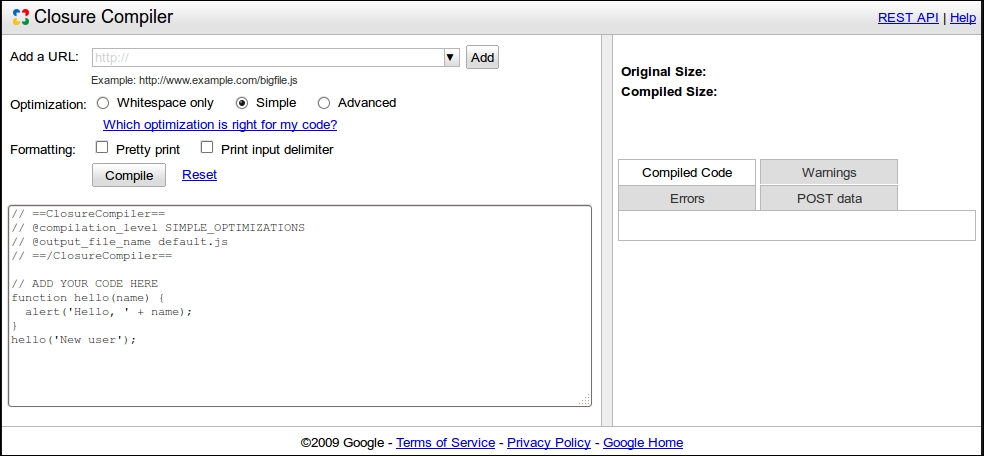
To display the ability of the Closure Compiler to compress files, we will ask you to try it online. Perform the following actions:
Cut and paste only the JavaScript content from inheritance use case replacing the
// ADD YOUR CODE HERE
section in the left-hand text area.Push on the Compile button and wait. You will see the Compiled Code tab going green.
Write on a paper or a spreadsheet Original Size and Compiled Size. You can also copy the generated code.
Change Optimization parameters to Whitespace only (Simple is the default that we have already tried) and note down the Original Size and the Compiled size again.
Repeat the last step with Optimization parameters set to Advanced.
The results you get from your experiment are as follows:
Compression level |
Original size |
Original gzipped size |
Save percentage |
Compiled size |
Compiled gzipped size |
Save gzipped percentage |
---|---|---|---|---|---|---|
WHITESPACE |
940 bytes |
391 bytes |
45.21% |
515 bytes |
238 bytes |
39.13% |
SIMPLE |
940 bytes |
391 bytes |
52.77% |
444 bytes |
224 bytes |
42.71% |
ADVANCED |
940 bytes |
391 bytes |
85.74% |
160 bytes |
141 bytes |
66.75% |
Let's take a look at the results of the different optimizations mode to compare:
goog.provide("myNamespace.layer.Layer");myNamespace.layer.Layer=function(options){this.color_=options.color||"grey"};myNamespace.layer.Layer.prototype.getColor=function(){return this.color_};goog.provide("myNamespace.layer.Vector");myNamespace.layer.Vector=function(options){goog.base(this,options);if(options.style)this.style_=options.style};goog.inherits(myNamespace.layer.Vector,myNamespace.layer.Layer);var myVector=new myNamespace.layer.Vector({color:"white",style:"myStyle"});console.log(myVector.getColor());
As you can see, everything is preserved. You only made the code compact by removing white space.
The SIMPLE mode:
var myNamespace={layer:{}};myNamespace.layer.Layer=function(a){this.color_=a.color||"grey"};myNamespace.layer.Layer.prototype.getColor=function(){return this.color_};myNamespace.layer.Vector=function(a){myNamespace.layer.Layer.call(this,a);a.style&&(this.style_=a.style)};goog.inherits(myNamespace.layer.Vector,myNamespace.layer.Layer);var myVector=new myNamespace.layer.Vector({color:"white",style:"myStyle"});console.log(myVector.getColor());
As you can see the compiler has done a substituting job by replacing all the
goog
functions. The only remaining namespaced function isgoog.inherits
. The function arguments are also renamed. It remains quite readable. If you replace the JavaScript from the example with this one, you can always call in your console the function with their original names.The ADVANCED mode:
function b(a){this.a=a.color||"grey"}function c(a){b.call(this,a);a.style&&(this.c=a.style)}goog.b(c,b);console.log((new c({color:"white",style:"myStyle"})).a);
As you can see in this case, most of the code is renamed. The code in itself will have the same behavior but you can't call it with the original functions you wrote.
The ADVANCED way of doing it is the more efficient way to compress, but it requires extra work as compared to the SIMPLE mode. In particular, you need to add extra comments to be able to run the compiler. We gave you a working example, but removed the text
@constructor
on the sample code and run again the ADVANCED mode and observe. Because of this mode when you are using foreign code such as an external library, you have to mention preserve this code from renaming. For this, you have to declare what we call externs. If you don't have available externs with the library you are using, your code will fail to execute correctly.Do not worry at the moment! The OpenLayers tools we will review already support jQuery externs, for example. We recommend that you go to the dedicated web pages from Google because it's an advanced feature (https://developers.google.com/closure/compiler/docs/api-tutorial3) and because we will review later how to solve it in the OpenLayers 3 context. You can also solve the problem using only the SIMPLE mode but you will lose a part of the gain of Closure Tools.
We will now see the application of what we learned so far in OpenLayers.