The final recipe of the chapter combines all the building blocks learned so far. Here is the output of our first drawing. As a kid, everyone loves to draw this:
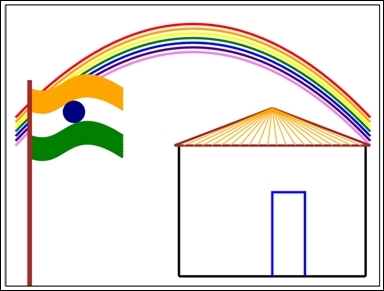
The recipe is just a combination of all the different functions developed and used so far. Here is the recipe:
<html> <head> <title>My House</title> <script type="text/javascript"> var can; var ctx; function init() { can = document.getElementById("myhouse"); ctx = can.getContext("2d"); drawRainbow(); drawHouse(); drawFlag(); } function drawLine(xstart,ystart,xend,yend,width,color) { ctx.beginPath(); ctx.lineJoin="miter"; ctx.strokeStyle=color; ctx.lineWidth=width; ctx.moveTo(xstart,ystart); ctx.lineTo(xend,yend); ctx.stroke(); ctx.closePath(); } function drawHouse() { x1=can.width/2-30; y1=can.height-20; x2=can.width-30; y2=can.height-20; color="black"; width=5; drawLine(x1,y1,x2,y2,width,color); //base drawLine(x1,y1,x1,can.height/2,width,color); //left wall drawLine(x2,y2,x2,can.height/2,width,color); //right wall x3=x1-10; y3=can.height/2; x4=x2+10; y4=can.height/2; drawLine(x3,y3,x4,y4,width,"brown"); //roof base x5=x1+(x2-x1)/2; //midpoint of the roof y5=can.height/2-80; drawLine(x3,y3,x5,y5,width,"brown"); //roof - left side drawLine(x4,y4,x5,y5,width,"brown"); //roof - right side //rslope=(x5-x4)/(y5-y4); j=20; k=23; g=1; m=4; for(i=1;i<=20;i++) { var X1=x3+j; var Y1=y3; var X2=x5; var Y2=y5; drawLine(var X1,var Y1,var X2,var Y2,2,"orange"); j=j+20; k=k+23; } //draw the door dX1=x1+(x5-x1); dY1=y1; dX2=x5; dY2=y5+180; ctx.beginPath(); ctx.strokeStyle="blue"; ctx.lineWidth=5; ctx.lineJoin="miter"; ctx.moveTo(dX1,dY1); ctx.lineTo(dX2,dY2); ctx.lineTo(dX2+70,dY2); ctx.lineTo(dX1+70,dY1); ctx.stroke(); } function drawRainbow() { y=can.height/2; x=can.width-20; mid=can.width/2; //rainbow - vibgyor yc=-100; drawQuadraticCurve(20,y,mid,yc,x,y,"violet"); drawQuadraticCurve(20,y-10,mid,yc-10,x,y-10,"indigo"); drawQuadraticCurve(20,y-20,mid,yc-20,x,y-20,"blue"); drawQuadraticCurve(20,y-30,mid,yc-30,x,y-30,"green"); drawQuadraticCurve(20,y-40,mid,yc-40,x,y-40,"yellow"); drawQuadraticCurve(20,y-50,mid,yc-50,x,y-50,"orange"); drawQuadraticCurve(20,y-60,mid,yc-60,x,y-60,"red"); } function drawFlag() { var xstart = 50; var ystart = 180; var xctrl1 = 130; var yctrl1 = 210; var xctrl2 = 130; var yctrl2 = 100; var xend = 250; var yend = ystart; //10 curves having orange color for(i=1;i<=10;i++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,"orange",6); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } //10 curves having white color for(j=1;j<=10;j++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,"white",6); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } //10 curves having green color for(j=1;j<=10;j++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,"green",6); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } x1=145; y1=228; drawArc(x1,y1,22,0,360,false,"black","navy"); //draw a stand for the flag drawLine(50,650,50,160,10,"brown"); } function drawQuadraticCurve(xstart,ystart,xControl, yControl, xEnd, yEnd,color,width) { //refer the Quadratic curve recipe //.... } function drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,color,width) { //refer the Bezier curve recipe //.... } function drawArc(xPos,yPos,radius,startAngle,endAngle,anticlockwise,lineColor,fillColor,width) { //refer the Arc recipe //.... } </script> </head> <body onload="init()"> <canvas id="myhouse" height="600" width="800" style="border:2px solid black;"> </canvas> </body> </html>
The recipe is built on the concepts learned in the previous recipes. You will see here the use of a line, an arc, a quadratic curve, and a Bezier curve. All of these drawings are the building blocks for this recipe of a house. The functions are the same as used in the previous recipes. These functions are called to create a flag, a rainbow, and a hut, using only lines, arcs, and curves. Shapes can make your job easier. The next chapter is all about shapes.