With both of the components having similar code, we can extract this similar code and create a mixin. This mixin can be imported in both of the components and their behavior will be the same:
- Create a file called defaultNumber.ts in the src/mixins folder.
- To code our mixin, we will import the Component and Vue decorators from the vue-class-component plugin, to be the base of the mixin. We will need to take a similar code and place it inside the mixin:
import Vue from 'vue';
import Component from 'vue-class-component';
@Component
export default class DefaultNumber extends Vue {
valueNumber: number = 0;
get formattedNumber() {
return `Your total number is: ${this.valueNumber}`;
}
}
- With the mixin ready, open the Counter.vue component on the src/components folder and import it. To do this, we need to import a special export from the vue-class-component called mixins and extend it with the mixin we want to extend. This will remove the Vue and Component decorators because they are already declared on the mixin:
<template>
<div>
<fieldset>
<legend>{{ this.formattedNumber }}</legend>
<button @click="increase">Increase By Ten</button>
<button @click="decrease">Decrease By Ten</button>
</fieldset>
</div>
</template>
<script lang="ts">
import Vue from 'vue';
import Component, { mixins } from 'vue-class-component';
import DefaultNumber from '../mixins/defaultNumber';
@Component
export default class CounterByTen extends mixins(DefaultNumber) {
increase() {
this.valueNumber += 10;
}
decrease() {
this.valueNumber -= 10;
}
}
</script>
- Now, when you run the npm run serve command on Terminal (macOS or Linux) or Command Prompt/PowerShell (Windows), you will see your component running and executing on screen:
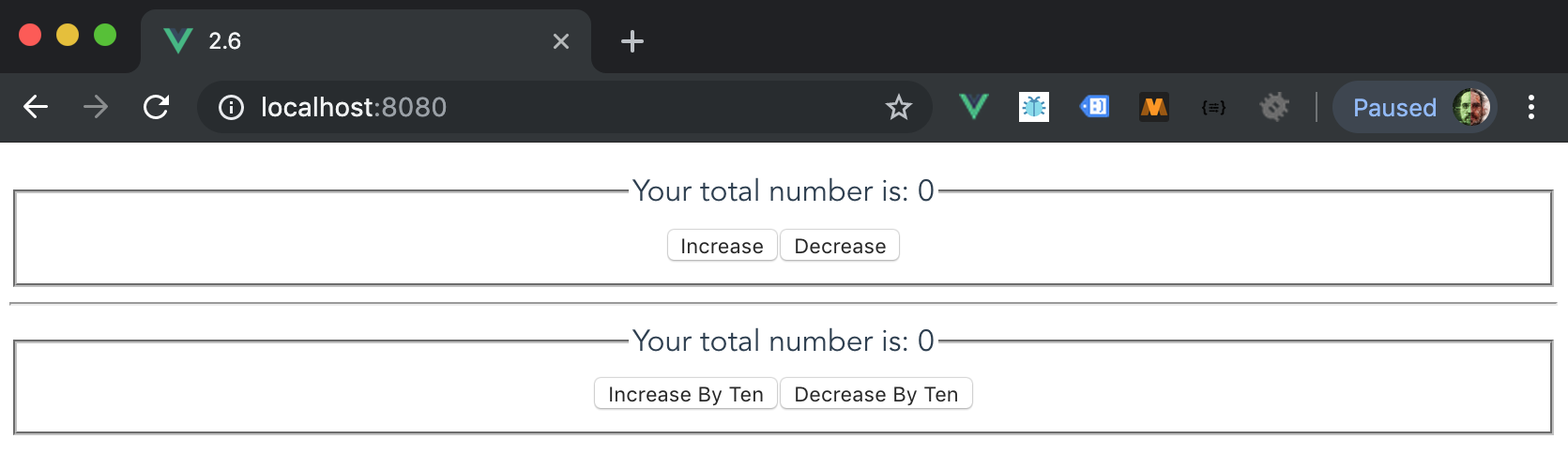