Technical requirements
You will need the following prerequisites to complete this chapter:
- The latest preview version of Visual Studio Community Edition or higher.
- Microsoft .NET 6 SDK.
- This book's source code: https://github.com/PacktPublishing/High-Performance-Programming-in-CSharp-and-.NET/tree/master/CH01.
- Optional: The latest Roslyn compiler built from source. The source code is available on GitHub at https://github.com/dotnet/roslyn. This should be automatically installed when you install the latest preview versions of Visual Studio.
Note
You can find the latest complete and up-to-date C# 10.0 feature set at https://github.com/dotnet/roslyn/blob/master/docs/Language%20Feature%20Status.md. At the time of writing, C# 10.0 is still undergoing much development and change. So, the contents of this book may not work as expected. If this turns out to be the case, then please refer to the preceding URL for the most relevant information to help you start working.
Obtaining and building the latest Roslyn compiler from the source code
Note
The build system of all .NET-related repositories has been in flux for several years now. We will provide the instructions for compiling Roslyn here; these were correct at the time of writing. For the latest instructions, please read the README.md
file located at https://github.com/dotnet/roslyn.
The following instructions are for downloading and building the latest version of the Roslyn compiler source on Windows 10:
- In the root of the
C:\
drive, clone the Roslyn source code by using the following command in the Windows Command Prompt:git clone https://github.com/dotnet/roslyn.git
- Then, run the following command:
cd Roslyn
- Restore the Roslyn dependencies by running the following command:
restore.cmd
- Build the Roslyn source code by running the following command:
build.cmd
- Test the Roslyn build by running the following command:
test.cmd
- Once all the tests have finished running, check the versions of C# that are accessible to the new computer. Do this by opening a Command Prompt window and navigating to
C:\roslyn\artifacts\bin\csc\Debug\net472
. - Then, run the following command:
csc /langversion:?
Note
I always run my Command Prompt as an administrator. Hence, the screenshots will show Command Prompt in administrative mode. But running Command Prompt as an administrator is not necessary for this exercise. Where Command Prompt must be executed as an administrator, this will be made clear as needed.
You should see something equivalent to the following:
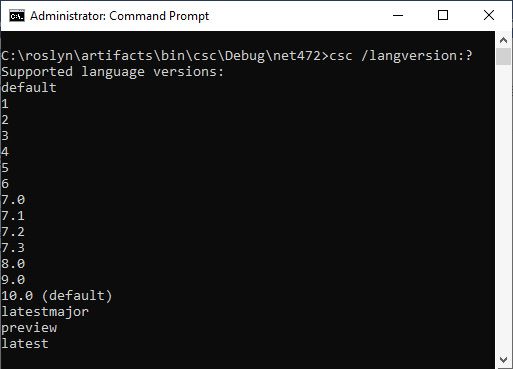
Figure 1.1 – The versions of the C# programming language supported by the compiler
As you can see, at the time of writing, version 10.0 of the C# language is available via the C# compiler. C# 10.0 is set as the default. The preview is still under development. The default version may be different on your computer.
Note
The latest version of Visual Studio 2022 should allow you to use the latest available C# 10.0 code features. If it doesn't, then compile the latest source and overwrite the files located at C:\Program Files (x86)\Microsoft Visual Studio\2022\Preview\MSBuild\Current\Bin\Roslyn
.
The following three sets of instructions provide compiler help for compiling a program that targets a specific C# version and then runs the program. These commands are for demonstrative purposes only, and you do not have to run them now:
csc /help csc -langversion:10.0 /out:HelloWorld.exe Program.cs csc HelloWorld
Now that you can build C# 10.0 from the command line and from within Visual Studio 2022, let's learn what kind of new development is taking place with Microsoft .NET 6.