Using Java packages
Packages are grouped collections of classes. If you look at the top of the code that we have written so far, you will see these lines of code:
import android.app.Activity; import android.view.Window; import android.os.Bundle;
These lines of code make available the Activity
and Bundle
classes along with their methods. Comment out two of the preceding lines like this:
//import android.app.Activity; import android.view.Window; //import android.os.Bundle;
Now look at your code, and you will see errors in at least three places. The word Activity
has an error because Activity
is a class that Android Studio is no longer aware of in the following line:
public class SubHunter extends Activity {
The word onCreate
also has an error because it is a method from the Activity
class, and the word Bundle
has an error because it is a class that since we commented out the previous two lines, Android Studio is no longer aware of. This next line highlights where the errors are:
protected void onCreate(Bundle savedInstanceState) {
Uncomment the two lines of code to resolve the errors, and we will add some more import…
code for the rest of the classes that we will use in this project, including one to fix the MotionEvent
class error.
Adding classes by importing packages
We will solve the error in the onTouchEvent
method declaration by adding an import
statement for the MotionEvent
class, which is causing the problem. Underneath the two existing import
statements, add this new statement, which I have highlighted:
package com.gamecodeschool.subhunter; // These are all the classes of other people's // (Android) code that we use for Sub Hunter import android.app.Activity; import android.os.Bundle; import android.view.MotionEvent;
Check the onTouchEvent
method, and you will see that the error is gone. Now, add these further import
statements directly underneath the one you just added, and that will take care of importing all of the classes that we need for this entire game. As we use each class throughout the next five chapters, I will introduce them formally. In the preceding code, I have also added some comments to remind me what import
statements do.
Add the highlighted code. The syntax needs to be exact, so consider copying and pasting the code:
// These are all the classes of other people's // (Android API) code that we use in Sub'Hunt import android.app.Activity; import android.view.Window; import android.os.Bundle; import android.view.MotionEvent; import android.graphics.Bitmap; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.Paint; import android.graphics.Point; import android.view.Display; import android.util.Log; import android.widget.ImageView; import java.util.Random;
Notice that the new lines of code are grayed-out in Android Studio. This is because we are not using them yet, and at this stage, many of them are technically unnecessary. Additionally, Android Studio gives us a warning if we hover the mouse pointer over the little yellow indicators to the right of the unused import
statements:
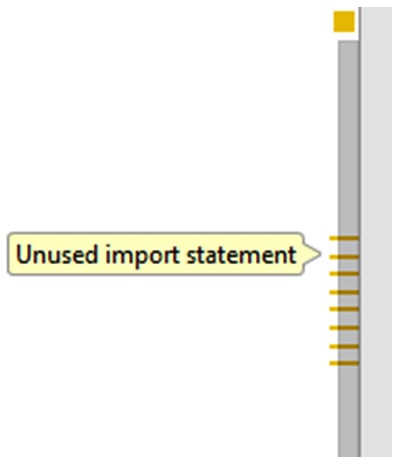
Figure 2.6 – Indicating unused import statements
This isn't a problem, and we are doing things this way for convenience as it is the first project. In the next project, we will learn how to add import
statements as and when needed without any fuss.
We have briefly mentioned the Activity
class. However, we need to learn a little bit more about it to proceed. We will do so while linking up our methods with method calls.