What are the four access modifiers and what do they do?
private
: This modifier makes a member only visible inside the classinternal
: This modifier makes a member only visible inside the class or within the same assemblyprotected
: This modifier makes a member only visible inside the class or derived classespublic
: This modifier makes a member visible everywhere
What is the difference between the
static
,const
, andreadonly
keywords?static
: This keyword makes the member shared by all instances and accessed through the typeconst
: This keyword makes a field a fixed literal value that should never changereadonly
: This keyword makes a field that can only be assigned at runtime using a constructor
How many parameters can a method have?
A method with 16383 parameters can be compiled, ran, and called. Any more than that and an unstated exception is thrown at runtime. IL has predefined opcodes to load up to four parameters and a special opcode to load up to 16-bits (65,536) parameters. A best practice is to limit your methods to three or four parameters. You can combine multiple parameters into a new class to encapsulate them into a single parameter. You can find more information on this at http://stackoverflow.com/questions/12658883/what-is-the-maximum-number-of-parameters-that-a-c-sharp-method-can-be-defined-as.
What does a constructor do?
A constructor allocates memory and initializes field values.
Why do you need to apply the
[Flags]
attribute to anenum
type when you want to store combined values?If you don't apply the
[Flags]
attribute to anenum
type when you want to store combined values, then a storedenum
value that is a combination will return as the stored integer value instead of a comma-separated list of text values.What is a delegate?
A delegate is a type-safe method reference. It can be used to execute any method with a matching signature.
What is an event?
An event is a field that is a delegate having the
event
keyword applied. The keyword ensures that only+=
and-=
are used; this safely combines multiple delegates without replacing any existing event handlers.Why is the
partial
keyword useful?You can use the
partial
keyword to split the definition of a type over multiple files.
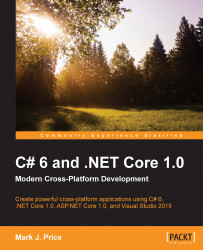
C# 6 and .NET Core 1.0
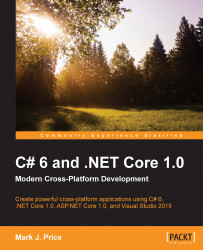
C# 6 and .NET Core 1.0
Overview of this book
With the release of .NET Core 1.0, you can now create applications for Mac OS X and Linux, as well as Windows, using the development tools you know and love. C# 6 and .NET Core 1.0 has been divided into three high-impact sections to help start putting these new features to work.
First, we'll run you through the basics of C#, as well as object-orient programming, before taking a quick tour through the latest features of C# 6 such as string interpolation for easier variable value output, exception filtering, and how to perform static class imports. We'll also cover both the full-feature, mature .NET Framework and the new, cross-platform .NET Core.
After quickly taking you through C# and how .NET works, we'll dive into the internals of the .NET class libraries, covering topics such as performance, monitoring, debugging, internationalization, serialization, and encryption. We'll look at Entity Framework Core 1.0 and how to develop Code-First entity data models, as well as how to use LINQ to query and manipulate that data.
The final section will demonstrate the major types of applications that you can build and deploy cross-device and cross-platform. In this section, we'll cover Universal Windows Platform (UWP) apps, web applications, and web services. Lastly, we'll help you build a complete application that can be hosted on all of today's most popular platforms, including Linux and Docker.
By the end of the book, you'll be armed with all the knowledge you need to build modern, cross-platform applications using C# and .NET Core.
Table of Contents (25 chapters)
C# 6 and .NET Core 1.0
Credits
About the Author
About the Reviewers
www.PacktPub.com
Preface
Hello, C#! Welcome, .NET Core!
Speaking C#
Controlling the Flow, Converting Types, and Handling Exceptions
Using Common .NET Types
Using Specialized .NET Types
Building Your Own Types with Object-Oriented Programming
Implementing Interfaces and Inheriting Classes
Working with Relational Data Using the Entity Framework
Querying and Manipulating Data with LINQ
Working with Files, Streams, and Serialization
Protecting Your Data and Applications
Improving Performance and Scalability with Multitasking
Building Universal Windows Platform Apps Using XAML
Building Web Applications and Services Using ASP.NET Core
Taking C# Cross-Platform
Building a Quiz
Answers to the Test Your Knowledge Questions
Creating a Virtual Machine for Your Development Environment
Index
Customer Reviews