Which .NET data provider would you use to work with Microsoft Access .MDB database files?
.NET Framework Data Provider for OLE DB.
Which .NET data provider would you use to work with Microsoft SQL Server 2012 Express Edition?
.NET Framework Data Provider for SQL Server.
What must you do with a
DbConnection
variable before executing aDbCommand
?Ensure that its state is open by calling the
Open()
method.When would you use the
CommandBehavior.SequentialAccess
property?This option provides a way for a
DbDataReader
class to handle rows that contain columns with BLOBs (binary large objects), such as videos and images, by not loading the entire row at once and instead loading one column at a time, allowing skipping of columns, and reading the BLOB data as a stream.ADO.NET instead of Entity Framework?
You would use classic ADO.NET instead of Entity Framework when you need the best performance, when most data access must use stored procedures, and when maintaining legacy code written using classic ADO.NET.
When defining a
DbContext
class, what type would you use for the property that represents a table, for example, theProducts
property of a Northwind context?DbSet<T>
, whereT
is the entity type, for example,Product
.What are the EF conventions for primary keys?
The property named
ID
orClassNameID
is assumed to be the primary key. If the type of that property is any of the following, then the property is also marked as being anIDENTITY
column:tinyint
,smallint
,int
,bigint
,guid
.When would you use an annotation attribute in an entity class?
You would use an annotation attribute in an entity class when the conventions cannot work out the correct mapping between the classes and tables. For example, if a class name does not match a table name or a property name does not match a column name.
Why might you choose fluent API in preference to annotation attributes?
You might choose fluent API in preference to annotation attributes when the conventions cannot work out the correct mapping between the classes and tables, and you do not want to use annotation attributes because you want to keep your entity classes clean and free from extraneous code.
What is the difference between Database-First and Code-First in EF6?
Database-First creates a design-time file with the EDMX that contains XML files that define the conceptual, storage, and mappings between the two. These XML files must be kept synchronized with future changes to the classes and tables. Code-First does not need a design-time EDMX file. Instead, a combination of conventions, annotation attributes, and fluent API is used to define the conceptual storage and mappings between the two. Code-First is more difficult to learn in the short term but it is more manageable in the long term which is why Entity Framework Core 1.0 drops support for design-time EDMX files.
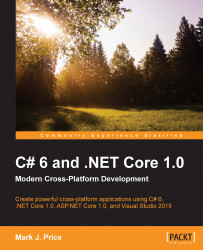
C# 6 and .NET Core 1.0
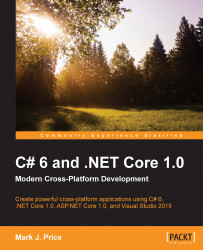
C# 6 and .NET Core 1.0
Overview of this book
With the release of .NET Core 1.0, you can now create applications for Mac OS X and Linux, as well as Windows, using the development tools you know and love. C# 6 and .NET Core 1.0 has been divided into three high-impact sections to help start putting these new features to work.
First, we'll run you through the basics of C#, as well as object-orient programming, before taking a quick tour through the latest features of C# 6 such as string interpolation for easier variable value output, exception filtering, and how to perform static class imports. We'll also cover both the full-feature, mature .NET Framework and the new, cross-platform .NET Core.
After quickly taking you through C# and how .NET works, we'll dive into the internals of the .NET class libraries, covering topics such as performance, monitoring, debugging, internationalization, serialization, and encryption. We'll look at Entity Framework Core 1.0 and how to develop Code-First entity data models, as well as how to use LINQ to query and manipulate that data.
The final section will demonstrate the major types of applications that you can build and deploy cross-device and cross-platform. In this section, we'll cover Universal Windows Platform (UWP) apps, web applications, and web services. Lastly, we'll help you build a complete application that can be hosted on all of today's most popular platforms, including Linux and Docker.
By the end of the book, you'll be armed with all the knowledge you need to build modern, cross-platform applications using C# and .NET Core.
Table of Contents (25 chapters)
C# 6 and .NET Core 1.0
Credits
About the Author
About the Reviewers
www.PacktPub.com
Preface
Hello, C#! Welcome, .NET Core!
Speaking C#
Controlling the Flow, Converting Types, and Handling Exceptions
Using Common .NET Types
Using Specialized .NET Types
Building Your Own Types with Object-Oriented Programming
Implementing Interfaces and Inheriting Classes
Working with Relational Data Using the Entity Framework
Querying and Manipulating Data with LINQ
Working with Files, Streams, and Serialization
Protecting Your Data and Applications
Improving Performance and Scalability with Multitasking
Building Universal Windows Platform Apps Using XAML
Building Web Applications and Services Using ASP.NET Core
Taking C# Cross-Platform
Building a Quiz
Answers to the Test Your Knowledge Questions
Creating a Virtual Machine for Your Development Environment
Index
Customer Reviews