What is the difference between using the
File
class and theFileInfo
class?The
File
class has static methods so it cannot be instantiated. It is best used for one-off tasks such as copying a file. TheFileInfo
class requires the instantiation of an object that represents a file. It is best used when you need to perform multiple operations on the same file.What is the difference between the
ReadByte
method and theRead
method of a stream?The
ReadByte
method returns a single byte each time it is called and theRead
method fills a temporary array with bytes up to a specified length. It is generally best to useRead
to process blocks of bytes at once.When would you use the
StringReader
, theTextReader
, and theStreamReader
classes?StringReader
is used for efficiently reading from a string stored in memoryTextReader
is an abstract class thatStringReader
andStreamReader
both inherit from for their shared functionalityStreamReader
is used for reading strings from a stream that can be any type of text file, including XML and JSON
What does the
DeflateStream
type do?DeflateStream
implements the same compression algorithm as GZIP but without a cyclical redundancy check, so although it produces smaller compressed files, it cannot perform integrity checks when decompressing.How many bytes per character does the UTF-8 encoding use?
It depends on the character. Most Western alphabet characters are stored using a single byte. Other characters may need two or more bytes.
What is an object graph?
An object graph is any instance of classes in memory that reference each other, thereby forming a set of related objects. For example, a
Customer
object may have a property that references a set ofOrder
instances.What is the best serialization format to choose for minimizing space requirements?
JavaScript Object Notation (JSON).
What is the best serialization format to choose for cross-platform compatibility?
eXtensible Markup Language (XML), although JSON is almost as good these days.
Which Microsoft technology uses the
DataContractSerializer
class by default?Windows Communication Foundation (WCF) for creating SOAP services.
Is it possible to create your own custom runtime serializers?
Yes. Create a class that implements the interface
System.Runtime.Serialization.IFormatter
. For details, visit:https://msdn.microsoft.com/en-us/library/system.runtime.serialization.iformatter(v=vs.110).aspx
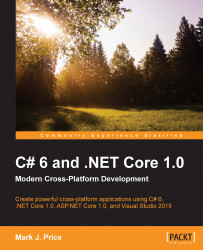
C# 6 and .NET Core 1.0
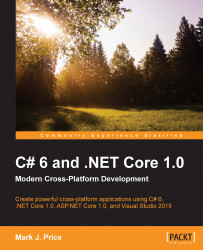
C# 6 and .NET Core 1.0
Overview of this book
With the release of .NET Core 1.0, you can now create applications for Mac OS X and Linux, as well as Windows, using the development tools you know and love. C# 6 and .NET Core 1.0 has been divided into three high-impact sections to help start putting these new features to work.
First, we'll run you through the basics of C#, as well as object-orient programming, before taking a quick tour through the latest features of C# 6 such as string interpolation for easier variable value output, exception filtering, and how to perform static class imports. We'll also cover both the full-feature, mature .NET Framework and the new, cross-platform .NET Core.
After quickly taking you through C# and how .NET works, we'll dive into the internals of the .NET class libraries, covering topics such as performance, monitoring, debugging, internationalization, serialization, and encryption. We'll look at Entity Framework Core 1.0 and how to develop Code-First entity data models, as well as how to use LINQ to query and manipulate that data.
The final section will demonstrate the major types of applications that you can build and deploy cross-device and cross-platform. In this section, we'll cover Universal Windows Platform (UWP) apps, web applications, and web services. Lastly, we'll help you build a complete application that can be hosted on all of today's most popular platforms, including Linux and Docker.
By the end of the book, you'll be armed with all the knowledge you need to build modern, cross-platform applications using C# and .NET Core.
Table of Contents (25 chapters)
C# 6 and .NET Core 1.0
Credits
About the Author
About the Reviewers
www.PacktPub.com
Preface
Hello, C#! Welcome, .NET Core!
Speaking C#
Controlling the Flow, Converting Types, and Handling Exceptions
Using Common .NET Types
Using Specialized .NET Types
Building Your Own Types with Object-Oriented Programming
Implementing Interfaces and Inheriting Classes
Working with Relational Data Using the Entity Framework
Querying and Manipulating Data with LINQ
Working with Files, Streams, and Serialization
Protecting Your Data and Applications
Improving Performance and Scalability with Multitasking
Building Universal Windows Platform Apps Using XAML
Building Web Applications and Services Using ASP.NET Core
Taking C# Cross-Platform
Building a Quiz
Answers to the Test Your Knowledge Questions
Creating a Virtual Machine for Your Development Environment
Index
Customer Reviews